Recently, many candidates have been complaining that Meta's interview difficulty has reached a "hard" level. I've come across some challenging questions from this year, and today, I want to discuss one particularly tricky matrix problem. If you're preparing for a Meta interview, this is definitely one to practice.
Problem Description
Given a matrix of integers, print out its values along the diagonals that move in the top right to bottom left direction. Each diagonal goes down and to the left as shown in the example. There should be newlines between each diagonal.
For example, given this matrix:
[[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]]
The printout should be:
1
5 2
9 6 3
10 7 4
11 8
12
At first glance, this seems like a simple traversal problem, but if you analyze it carefully, you'll realize it’s much trickier than a standard matrix traversal.
Challenges in the Problem
- It’s not row-wise or column-wise traversal but diagonal traversal, which is not a common approach and can be difficult to conceptualize.
- The matrix may not be a square, so the implementation must handle cases where rows outnumber columns and vice versa.
- Determining the starting points for each diagonal is key to solving the problem. There are two sets of starting points:
- The first set includes all elements in the top row, from (0,0) to (0, n-1).
- The second set includes all elements in the rightmost column, from (1, n-1) to (m-1, n-1).
Solution Implementation
During the interview, CSOAHelp provided real-time assistance, guiding the candidate to quickly understand the problem and implement the solution. The candidate didn't need to think independently but followed CSOAHelp’s structured guidance step by step.
def print_diagonal(matrix):
if not matrix or not matrix[0]:
return
m, n = len(matrix), len(matrix[0])
# Traverse the upper triangular region (including the first row)
for col in range(n):
i, j = 0, col
while i < m and j >= 0:
print(matrix[i][j], end=" ")
i += 1
j -= 1
print()
# Traverse the lower triangular region (excluding the first column)
for row in range(1, m):
i, j = row, n - 1
while i < m and j >= 0:
print(matrix[i][j], end=" ")
i += 1
j -= 1
print()
Code Explanation
CSOAHelp broke down the approach and guided the candidate through the implementation to ensure clarity and efficiency.
- Traverse the upper triangular region (including the first row): Extract elements from
(0,0)
to(0, n-1)
as starting points for diagonals. - Traverse the lower triangular region (excluding the first column): Extract elements from
(1, n-1)
to(m-1, n-1)
as the starting points for the remaining diagonals. - Follow each diagonal: Starting from each point, move down-left (
i+1, j-1
) until the boundary is reached. - Print new lines between diagonals to ensure proper formatting.
Interview Follow-Up Questions
What is the time complexity of this solution?
CSOAHelp’s Hint: This approach visits every element in the matrix exactly once, leading to a time complexity of O(m * n)
, where m
is the number of rows and n
is the number of columns. Since there are no redundant calculations or nested loops, this is the most optimal time complexity.
Candidate’s Response: The time complexity is O(m * n)
because each element is visited exactly once without redundant operations.
How can we optimize space usage for very large matrices?
CSOAHelp’s Hint: The current solution has O(1) space complexity, as it only uses a few extra variables. However, for extremely large matrices, memory usage and computation efficiency might become concerns. We can adopt a streaming approach, printing elements directly during traversal instead of storing them in memory. Additionally, we can use block processing, dividing the matrix into smaller segments for sequential processing.
Candidate’s Response: This method has an O(1)
space complexity since it only uses a few extra variables. If the matrix is very large, we can optimize by printing elements immediately instead of storing them. Additionally, using block processing can help efficiently handle large datasets.
How can we improve code readability?
CSOAHelp’s Hint: Code readability can be enhanced through modularization. Extracting print()
logic into a separate function, such as print_diagonal_line()
, will make the main logic clearer. Additionally, adding comments to clarify traversal order and boundary conditions will help others understand the code. Using list comprehensions or iterators can also simplify traversal.
Candidate’s Response: To improve readability, we can encapsulate the print()
logic into a dedicated function print_diagonal_line()
, making the main function cleaner. Adding comments to explain traversal order and boundary conditions will make the code easier to follow. Additionally, using iterators or list comprehensions can make the code more concise.
The interviewer was impressed by the candidate’s clear understanding and structured responses.
Conclusion
Meta’s interview process has undoubtedly become more challenging. These non-traditional traversal problems test a candidate’s pattern recognition and code implementation skills. Without prior exposure to similar problems, candidates may struggle to develop an efficient solution under time constraints.
If you feel unprepared for such difficult questions, CSOAHelp can be your best ally. Our real-time interview assistance provides precise guidance, ensuring you stay on track and answer questions step by step with confidence. Want to ace your Meta interview? Try CSOAHelp today and make your interview experience smoother!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
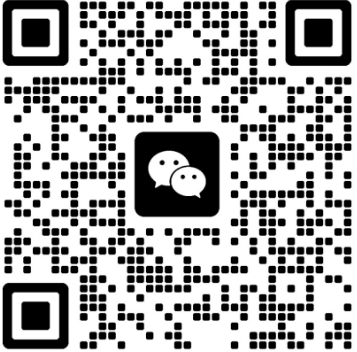