Given a string message and an integer n, replace every nth consonant with the next consonant from the alphabet while keeping the case consistent (e.g. 'b' becomes 'c', 'X' becomes 'Y', etc.).
Notes:
- The list of consonants in alphabetical order is:
'b', 'c', 'd', 'f', 'g', 'h', 'j', 'k', 'l', 'm', 'n', 'p', 'q', 'r', 's', 't', 'v', 'w', 'x', 'y', 'z' - 'z' must be replaced with 'b' (and 'Z' with 'B').
- Also note that you are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(message.length²) will fit within the execution time limit.
Example:
For message = "CodeSignal" and n = 3, the output should be
solution(message, n) = "CodeTignam"
Explanation:
- The given string "CodeSignal" contains the following consonants: 'C', 'd', 'S', 'g', 'n', 'l'.
- 'C' is the first consonant so it is unchanged.
- 'd' is the second consonant so it is unchanged.
- 'S' is the third consonant so it is replaced with 'T'.
- 'g' is the first consonant so it is unchanged.
- 'n' is the second consonant so it is unchanged.
- 'l' is the third consonant so it is replaced with 'm'.
- Finally, the resulting string becomes "CodeTignam".
In a logistics company, you are given an array of integers packageCodes, representing the codes assigned to packages processed by the system.
Analyze packageCodes and find the codes that are both divisible by 3 and contain the digit '7' at least twice. Return the number of such codes.
Note:
You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(packageCodes.length²) will fit within the execution time limit.
Example:
For packageCodes = [771, 3702, 3727, 777, 273, 777, 456], the output should be
solution(packageCodes) = 3.
Explanation:
- 771: contains the digit '7' twice and is divisible by 3.
- 3702: contains the digit '7' only once.
- 3727: contains the digit '7' twice but is NOT divisible by 3.
- 777: contains the digit '7' thrice and is divisible by 3.
- 273: contains the digit '7' only once.
- 777: contains the digit '7' thrice and is divisible by 3. Even though the same code already appeared in the list earlier, we count it again.
- 456: does not contain the digit '7' at all.
So, the number of codes that are both divisible by 3 and contain the digit '7' at least twice is 3. These codes are 771, 777, and 777.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
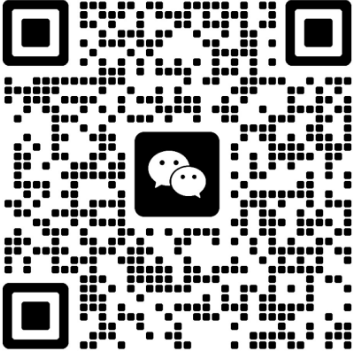