The new year has arrived! 2025 is here, and first of all, I wish everyone a year of success, continuous technical improvement, and successful interviews! Every new year, many people plan their career moves, and an Apple technical interview is undoubtedly a dream challenge for many developers. Today, let’s explore a classic Apple interview question and see how, with the help of CSOAHelp, you can efficiently solve problems and impress the interviewer.
This Apple interview question involves task dependency sorting, where a set of tasks is given, and some tasks must be completed before others can start. The goal is to find a valid order of execution so that all tasks can be completed. If multiple valid orders exist, any one of them is acceptable.
At the start of the interview, the interviewer briefly introduced the background of the problem and highlighted key aspects:
"We have a new project which is very critical to Apple. Many tasks need to be done to finish the project. There are, naturally, dependencies between tasks, for example, you have to finish the database schema design before you can load the seed data to the database; you have to finish the UX design before implementing the UI implementation..."
After hearing the problem, the candidate took a moment to think, while CSOAHelp quickly provided a structured approach:
CSOAHelp’s Guidance:
- This problem is essentially a topological sorting problem on a directed graph.
- We can solve it using Kahn’s algorithm (BFS) or DFS recursion to find a valid execution order.
- First, construct a dependency graph (adjacency list) and calculate the in-degree for each task.
- Identify tasks with an in-degree of 0 (tasks with no dependencies) and execute them first. Gradually remove completed tasks and update dependencies.
- If the final count of completed tasks matches the total number of tasks, return the sorted order; otherwise, a circular dependency exists, making execution impossible.
The candidate repeated CSOAHelp’s guidance and explained the core concept of topological sorting to the interviewer, emphasizing the advantages of using BFS, such as efficiently handling large-scale task dependencies. The interviewer acknowledged the explanation and asked the candidate to implement the code.
CSOAHelp’s Code Implementation:
from collections import deque
def find_task_order(n, dependencies):
graph = {i: [] for i in range(n)} # Build task dependency graph
in_degree = {i: 0 for i in range(n)} # Track in-degree of each task
for a, b in dependencies:
graph[b].append(a)
in_degree[a] += 1
queue = deque([i for i in range(n) if in_degree[i] == 0]) # Find tasks with zero in-degree
order = []
while queue:
task = queue.popleft()
order.append(task)
for next_task in graph[task]:
in_degree[next_task] -= 1
if in_degree[next_task] == 0:
queue.append(next_task)
return order if len(order) == n else [] # Return empty list if cycle exists
The candidate implemented the code step by step and explained the logic as per CSOAHelp’s instructions. The interviewer then asked:
Interviewer: What is the time complexity? How would you optimize it for a very large number of tasks?
CSOAHelp’s Guidance:
- Since all tasks and dependencies are traversed, the time complexity is O(n + m), where
n
is the number of tasks andm
is the number of dependencies. - For large-scale task dependencies, parallel computation techniques such as multithreading or distributed computing can help reduce scheduling bottlenecks.
- Another approach is incremental task graph construction, where only newly added or modified tasks are adjusted rather than recalculating the entire order every time.
The candidate repeated CSOAHelp’s response and added personal insights on distributed computing. The interviewer was satisfied with the answer.
At the end of the interview, the interviewer asked about the candidate’s views on Apple’s corporate culture and engineering team.
Interviewer: Apple emphasizes innovation and engineering quality. In your experience, how do you balance efficient development with high-quality code?
CSOAHelp’s Guidance:
- Ensure quality through code reviews and automated testing.
- Use iterative development to avoid overcomplicating the implementation at once.
- Focus on maintainability, writing clean, modular code so team members can quickly understand and improve it.
The candidate directly repeated CSOAHelp’s answer and supplemented it with examples of how teams use code reviews and continuous integration to ensure code quality. The interviewer acknowledged the response.
The interview concluded successfully, and the candidate expressed gratitude for CSOAHelp’s guidance throughout the process.
As we step into the new year, may everyone tackle technical interviews with confidence and land their dream offers! A new year brings new challenges and opportunities—wishing all readers success in 2025!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
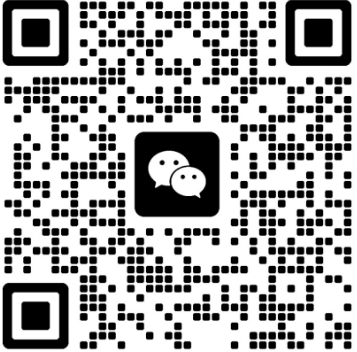