Meta's technical interviews are renowned for their complexity and the intense pressure they place on candidates. The Virtual Onsite (VO) stage, in particular, is designed not only to assess a candidate's coding abilities but also their capacity to remain composed under stress while handling layered questions from interviewers. In this story, we’ll explore how a candidate, with the silent guidance of CSOAHELP, managed to tackle a challenging problem and deliver a standout performance.
Interview Problem: Find Nodes at Distance K
Given a binary tree (pointer to the root), a target node anywhere in the tree, and an integer value K.
Return a list of the values of all the nodes that have a distance K from the target node.
The order of the list does not matter.
Example: Consider the following tree:
5
/ \
8 3
/ \ \
1 9 4
/ \
2 6
\
7
If the target node is 3
and K = 2
, the output is [2, 6, 7, 8]
(order does not matter).
The Candidate's Challenge
When the candidate first encountered the problem, they understood the requirements but froze momentarily. Despite prior training in tree traversal and pathfinding, the pressure of a high-stakes interview made it difficult to think clearly.
The candidate initially proposed a simple solution that involved recursively searching for nodes at distance K within the target node’s subtree. However, the interviewer quickly followed up with more challenging questions:
- “What if nodes at distance K are located in the parent or sibling branches of the target node?”
- “How would your solution handle large-scale trees with millions of nodes? Is your algorithm efficient?”
The intensity of these questions, coupled with the candidate’s nervousness, caused them to falter. This was the moment when CSOAHELP’s guidance became critical.
How CSOAHELP Turned the Situation Around
Behind the scenes, CSOAHELP provided the candidate with calm, structured prompts to help them refocus and refine their approach. The first nudge was:
“Break the problem into two parts: first, find nodes at distance K within the target’s subtree; second, backtrack to account for nodes at distance K via parent or sibling branches.”
This simple yet powerful suggestion allowed the candidate to regain composure and reorganize their thoughts. They quickly adapted their solution to include both forward and backward traversal, implementing the following code:
Solution Code
from collections import deque
def find_at_distance(root, target, K):
# Step 1: Build a parent mapping for all nodes
def build_parent_map(node, parent):
if not node:
return
if parent:
parent_map[node] = parent
build_parent_map(node.left, node)
build_parent_map(node.right, node)
# Step 2: Perform BFS to find nodes at distance K
def bfs_from_target(target, K):
queue = deque([(target, 0)]) # (current node, current distance)
visited = set([target])
result = []
while queue:
current, distance = queue.popleft()
if distance == K:
result.append(current.val)
elif distance < K:
for neighbor in (current.left, current.right, parent_map.get(current)):
if neighbor and neighbor not in visited:
visited.add(neighbor)
queue.append((neighbor, distance + 1))
return result
parent_map = {}
build_parent_map(root, None)
return bfs_from_target(target, K)
Interviewer’s Follow-Up Questions and the Candidate’s Responses
The interviewer pressed further:
- “How would you optimize your solution for very large trees?”
- “What if there are multiple target nodes? How would you adjust your approach?”
With CSOAHELP’s real-time assistance, the candidate responded confidently:
“For large-scale trees, I would avoid explicitly building a parent map and instead track parent relationships directly during recursive traversal. This reduces space complexity.”
When asked about multiple target nodes, they explained:
“In such cases, I would initiate BFS from all target nodes simultaneously. By sharing the visited set across searches, I can ensure efficiency and avoid redundant computations.”
CSOAHELP’s subtle guidance included:
“Emphasize shared visited sets and space-efficient traversal to handle scalability concerns.”
Finally, the candidate provided a comprehensive complexity analysis:
“The time complexity is O(N), where N is the number of nodes, as each node is visited at most once. The space complexity is also O(N), accounting for the BFS queue and visited set.”
The interviewer seemed impressed, nodding in acknowledgment of the candidate’s thorough and well-structured answers.
The Invisible Hand of CSOAHELP
Throughout the interview, CSOAHELP played an instrumental role in the candidate’s success:
- Refocusing Thought Processes: When the candidate froze, CSOAHELP provided a clear breakdown of the problem, allowing them to regain clarity.
- Navigating Follow-Ups: By supplying concise prompts, CSOAHELP ensured the candidate could handle complex questions without missing key points.
- Enhancing Communication: CSOAHELP helped the candidate articulate their thoughts with precision, especially during discussions about scalability and optimization.
The candidate appeared confident and composed, but behind the scenes, every critical response was shaped by CSOAHELP’s silent support.
Conclusion
Meta’s interviews are designed to push candidates to their limits, testing not only technical skills but also problem-solving resilience under pressure. With CSOAHELP’s assistance, this candidate overcame moments of self-doubt, delivered a well-rounded solution, and impressed the interviewer with their clarity and depth of understanding.
For anyone aspiring to succeed in challenging interviews, CSOAHELP serves as an invaluable companion, offering the subtle guidance needed to showcase one’s true potential. In this instance, the candidate demonstrated that even the most daunting challenges can be overcome with the right support system, paving their way to success at Meta.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
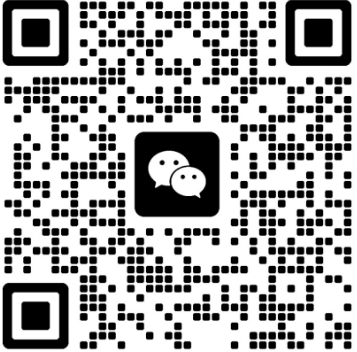