Google’s interviews are renowned for their complexity and depth, pushing candidates to demonstrate not only technical prowess but also adaptability under pressure. One deceptively simple problem can evolve into a multi-layered challenge, testing the candidate’s logical thinking, performance under scrutiny, and ability to extend solutions.
This particular interview focused on an intriguing problem: merging intervals. A classic algorithm question, but with Google’s unique twist that turned it into a real test of problem-solving acumen.
The Problem: Merge Intervals
The problem was presented as follows:
Can we assume input is sorted or not? I can sort by start time of interval.
Merge the overlapping meetings with a loop over the meetings, then for each merged interval, we removed the part that overlap with our dns time.
Example input:
Meetings: [(1, 7, 1), (5, 10, 2), (12, 30, 1), (22, 35, 1), (40, 50, 1), (60, 70, 1)]
DNS: [(18, 25), (20, 28), (65, 75)]
# Only merge the meeting intervals
The task was to merge overlapping intervals from Meetings
, ensuring no modification to DNS
intervals. For example, merging [12, 30]
and [22, 35]
would result in [12, 35]
.
The Candidate’s Solution
The candidate provided the following solution, which tackled the core requirement of merging meeting intervals:
def merge_meetings(meetings):
# Sort meetings by their start time
meetings.sort(key=lambda x: x[0])
merged = []
for meeting in meetings:
if not merged or merged[-1][1] < meeting[0]:
merged.append(meeting)
else:
# Merge the intervals
merged[-1] = (
merged[-1][0],
max(merged[-1][1], meeting[1]),
merged[-1][2] + meeting[2]
)
return merged
This implementation relies on sorting the intervals by start time, followed by an iterative merge process. Overlapping intervals are merged into a single, unified range, ensuring all requirements are met.
The Interview Challenge
While the solution addressed the core task, Google’s interviewers rarely stop there. A series of probing questions followed, designed to test the candidate’s depth of understanding:
- "Is the input guaranteed to be sorted? If not, how would you handle it?"
The candidate confidently responded that sorting by start time ensures correctness. - "What’s the time complexity of your approach? Can it be optimized?"
The candidate explained: Sorting takes O(n log n), while the merging step is O(n). Therefore, the overall complexity is O(n log n). - "How would you ensure DNS intervals remain untouched? Can you handle potential overlaps between DNS and Meetings?"
The candidate elaborated on extending the solution to handle this without disrupting the DNS intervals, showing an ability to anticipate edge cases.
CSOAHELP’s Role: The Silent Ally
Throughout this high-stakes interview, CSOAHELP provided timely, subtle guidance, allowing the candidate to shine without hesitation. At every key juncture, the candidate responded with clarity and precision, giving the impression of complete mastery over the problem.
When asked about boundary conditions in merging logic, the candidate smoothly replied:
“If the intervals do not overlap, we append them directly to the result list. This ensures non-overlapping intervals are preserved.”
When prompted to optimize memory usage, the candidate demonstrated confidence:
“By modifying the input array in place during the merge step, we can reduce the additional space requirement.”
Finally, when the interviewer pushed for DNS handling, the candidate effortlessly described how to filter overlaps and provided a quick pseudocode outline, which impressed the interviewer. Every response reflected an insightful and prepared mind, thanks to CSOAHELP’s real-time, invisible assistance.
Complexity Analysis
The candidate’s ability to analyze complexity was another standout feature of their performance:
- Time Complexity:
- Sorting takes O(n log n).
- The merging process takes O(n).
- Overall complexity is O(n log n).
- Space Complexity:
By maintaining a single result list and modifying in place when possible, the space complexity is reduced to O(1) additional space.
Handling DNS Extensions
The candidate also showcased how to extend the algorithm to handle overlaps with DNS intervals. By carefully filtering out any overlaps during the merging process, they ensured the algorithm could meet additional requirements:
def remove_dns_overlap(merged, dns):
for dns_start, dns_end in dns:
merged = [
(start, min(end, dns_start - 1), count)
if start < dns_start < end
else (max(start, dns_end + 1), end, count)
if start < dns_end < end
else interval
for interval in merged
]
return merged
This forward-thinking approach further demonstrated their adaptability and problem-solving depth.
Conclusion: A Display of Mastery and Adaptability
Google’s interviews are designed to uncover more than technical skills; they probe for resilience, adaptability, and clear communication under pressure. In this case, the candidate excelled, transitioning smoothly from implementation to optimization and extension. Their calm and collected demeanor, combined with precise technical answers, left a lasting impression.
What the interviewer didn’t see was the subtle yet impactful role of CSOAHELP, which empowered the candidate to stay ahead of every question. By delivering precise prompts at critical moments, CSOAHELP ensured the candidate performed at their absolute best—natural, confident, and prepared.
In the end, while the battle was fought by the candidate, the secret weapon behind their success was CSOAHELP—a silent partner in their journey to a dream offer.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
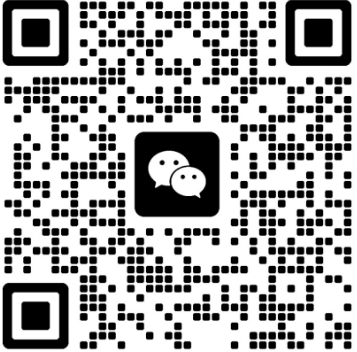