At Jane Street, technical interviews are well known for their focus on problem-solving, mathematical rigor, and efficient design. In this article, we’ll walk through a candidate’s approach to solving a challenging game simulation problem involving an infinite 2D grid. We’ll highlight each step, including problem clarification, solution design, code discussion, and interview best practices with csoahelp providing key preparation support.
The Interview Problem: 2D Infinite Grid Game
Problem Statement:
"Simulate a game on a 2D infinite grid. The grid extends infinitely in the left, right, and top directions but is limited at the bottom (y = 0).
- Two players, Red ('R') and Blue ('B'), take turns placing pieces.
- Implement the following functionality:
- Maintain the state of the board efficiently.
- Design a move function that takes an x-coordinate and places the player’s piece at the bottom of the column.
- Check for a win condition where a player has K consecutive pieces either vertically or horizontally.
Constraints:
- The grid must handle an infinite range of x positions.
- The solution should aim for optimal performance.
Step 1: Problem Clarification
Before jumping into the implementation, the candidate clarified key aspects of the problem:
Candidate:
“Just to confirm, the grid extends infinitely to the left and right, which means x-coordinates can include negative values, correct? Also, y-coordinates start at 0 and only increase upward?”
Interviewer:
“Yes, that’s correct. The grid is infinite in the horizontal direction, but y starts at 0.”
Candidate:
“To represent the grid efficiently, I think a hashmap would work well. The key can represent the x-coordinate, and the value will be an array of pieces in that column.”
Why Clarification Matters:
csoahelp emphasizes the importance of asking clarifying questions. It shows attention to detail and ensures alignment on constraints before starting implementation.
Step 2: Solution Design
The candidate then presented a well-structured approach:
- Data Representation:
- Use a hashmap where:
- The key is the x-coordinate (supports negative integers).
- The value is a list that represents the column's stack of pieces.
- Adding a new piece to the column is an O(1) operation using list append.
- Use a hashmap where:
- Move Function:
- Add the player’s piece (‘R’ or ‘B’) to the end of the list for the given x-coordinate.
- Win Condition:
- Check for consecutive pieces:
- Vertical Check: Count consecutive pieces starting from the bottom of the column.
- Horizontal Check: Count consecutive pieces by scanning left and right across neighboring columns at the same y-level.
- Check for consecutive pieces:
Candidate:
“I chose a hashmap because it efficiently supports infinite x-coordinates and only stores columns that have pieces. Adding a piece to the end of a list is O(1), which is optimal.”
Interviewer:
“Good reasoning. Can you explain how you’ll detect the win condition?”
Candidate:
“Sure, I’ll check two directions:
- Vertical: Traverse the current column from bottom to top to count consecutive pieces.
- Horizontal: For the same row, check consecutive pieces to the left and right in neighboring columns.”
Step 3: Handling Edge Cases
The interviewer then challenged the solution with edge cases:
Interviewer:
“What happens if two players simultaneously achieve a win condition?”
Candidate:
“I’ll handle this by writing a helper function to check both players simultaneously. If a move triggers wins for both players, the function can return both winners.”
Interviewer:
“Interesting. What if a move shifts rows upward and inadvertently causes another alignment?”
Candidate:
“That’s a great point. I’ll account for this by always checking the bottom-most piece and evaluating its neighbors after any upward shifts. This ensures we don’t miss new alignments.”
How Preparation Helps:
With csoahelp training, the candidate practiced thinking through edge cases and communicating solutions effectively under pressure.
Step 4: Time and Space Complexity Analysis
After explaining the implementation, the candidate provided a clear analysis of the solution’s efficiency:
Candidate:
- Move Function: Adding a piece to a column is O(1) due to the list append operation.
- Win Check:
- Vertical Check: Linear scan of a single column, O(K), where K is the number of pieces to win.
- Horizontal Check: Traverse neighboring columns up to K positions left and right, also O(K).
Overall Time Complexity: O(K) per move.
Space Complexity: Proportional to the number of columns and pieces, O(N), where N is the total number of pieces placed.
Interviewer:
“Good explanation. Your solution is efficient and meets the problem constraints.”
Step 5: Questions for the Interviewer
At the end of the interview, the candidate asked thoughtful questions to show genuine interest in the role and team:
- “Could you describe the team structure and typical collaboration process?”
- “What does the onboarding process look like for new team members?”
- “What types of tasks or projects would I be working on at my level?”
Why It Matters:
csoahelp advises candidates to prepare thoughtful questions that show curiosity about the team and company culture. It leaves a strong, positive impression.
Conclusion: The Role of csoahelp in Interview Success
In this Jane Street technical interview, the candidate demonstrated the ability to:
- Clarify Requirements: Ensuring a complete understanding of problem constraints.
- Design Efficient Solutions: Selecting an optimal data structure (hashmap) and explaining design choices clearly.
- Handle Edge Cases: Accounting for simultaneous wins and shifted alignments.
- Communicate Effectively: Providing step-by-step explanations and clear time/space complexity analyses.
- Ask Insightful Questions: Leaving the interviewer with a positive impression.
csoahelp played a key role in the candidate’s success by:
- Offering targeted mock interviews for design problems.
- Providing structured feedback to improve problem-solving and communication skills.
- Training candidates to handle edge cases and analyze complexity effectively.
If you’re preparing for challenging interviews like this, csoahelp can help you build the confidence, skills, and strategies needed to succeed. Take your interview performance to the next level with csoahelp!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
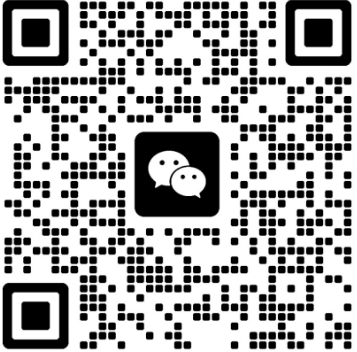