1. Pairs
Consider two arrays of integers, a[n] and b[n]. What is the maximum number of pairs that can be formed where a[i] > b[j]? Each element can be in no more than one pair.
Find the maximum number of such possible pairs.
Example:
Input:
n = 3
a = [1, 2, 3]
b = [2, 1, 1]
Explanation:
Two ways the maximum number of pairs can be selected:
- {a[1], b[0]} = {2, 1} and {a[2], b[2]} = {3, 1} are valid pairs.
- {a[1], b[0]} = {2, 1} and {a[2], b[1]} = {3, 2} are valid pairs.
No more than 2 pairs can be formed, so return 2.
Function Description:
Complete the function findNumOfPairs in the editor below.
Function Signature:
def findNumOfPairs(a: List[int], b: List[int]) -> int:
Parameters:
- a[n]: an array of integers
- b[n]: an array of integers
Returns:
- int: the maximum number of pairs
Constraints:
- 1 ≤ n ≤ 10^5
- 1 ≤ a[i] ≤ 10^9
- 1 ≤ b[i] ≤ 10^9
Input Format for Custom Testing:
Sample Input:
5
1
2
3
4
5
5
6
6
1
1
1
Sample Output:
3
Explanation:
Valid pairs are:
- {a[1], b[2]}
- {a[2], b[3]}
- {a[3], b[4]}
Other possible valid pairs include:
- {2, 1}, {3, 1}, {4, 1}.
The maximum number of pairs is 3. Return 3 as the answer.
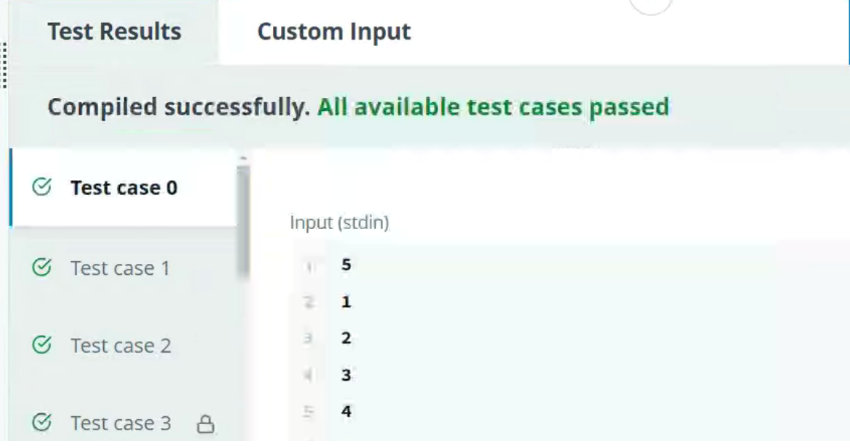
2. Two Operations
Two integer operations are defined as:
- ADD_1: Increment the integer by 1
- MULTIPLY_2: Multiply the integer by 2
Given an integer value k, determine the minimum number of operations it takes to get from 0 to k using the two operations specified above.
Example:
Input:
kValues[i] = 8
Explanation:
Starting from 0:
- Add 1 → then multiply by 2 three times.
It takes a minimum of 4 operations to get to 8, so store 4 in index 0 of the return array.
Function Description:
Complete the function getMinOperations in the editor.
Function Signature:
def getMinOperations(kValues: List[int]) -> List[int]:
Parameters:
- kValues[n]: an array of integers representing the target values.
Returns:
- List[int]: answers to a list of queries in the given order.
Constraints:
- 1 ≤ n ≤ 10000
- 0 ≤ kValues[i] ≤ 10^16
Input Format for Custom Testing:
Sample Input:
2
5
3
Sample Output:
4
3
Explanation:
- To get from 0 to kValues[0] = 5:
ADD_1 → MULTIPLY_2 → MULTIPLY_2 → ADD_1- 0 + 1 → 1 × 2 → 2 × 2 → 4 + 1 = 5
- To get from 0 to kValues[1] = 3:
ADD_1 → MULTIPLY_2 → ADD_1- 0 + 1 → 1 × 2 → 2 + 1 = 3
Final Output:
Return the array [4, 3] as the answer.
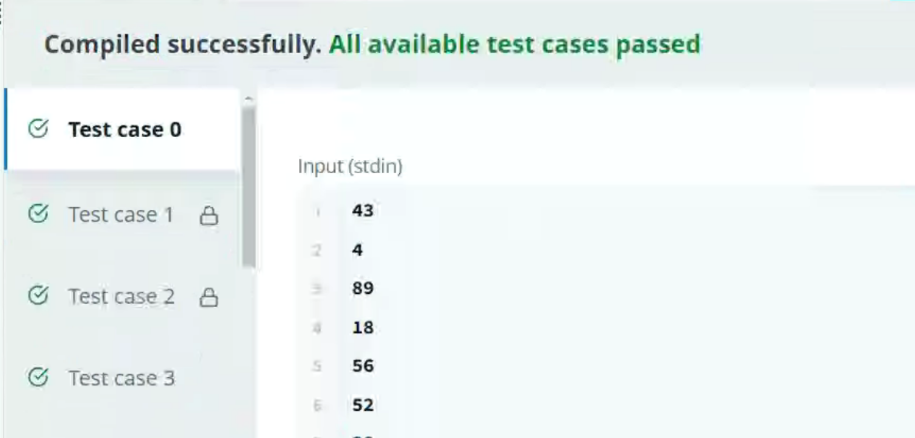
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
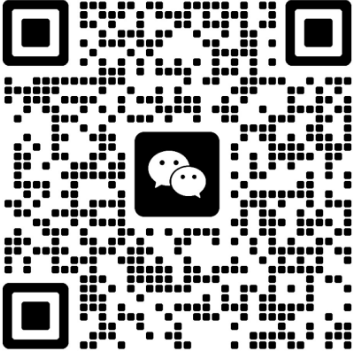