Problem Description
You are given an integer array prices
, where prices[i]
represents the stock price on day i
. Your task is to find the maximum profit you can achieve with at most two transactions.
Constraints:
- You must sell the stock before buying again.
- If no profit can be achieved, you may choose not to make any transactions.
Examples
- Input:
prices = [3,3,5,0,0,3,1,4]
Output:6
Explanation:- First transaction: Buy on day 4 (price = 0), sell on day 6 (price = 3), profit = 3.
- Second transaction: Buy on day 7 (price = 1), sell on day 8 (price = 4), profit = 3.
- Total profit = 3 + 3 = 6.
- Input:
prices = [1,2,3,4,5]
Output:4
Explanation:- Buy on day 1 (price = 1), sell on day 5 (price = 5), profit = 4.
- Input:
prices = [7,6,4,3,1]
Output:0
Explanation: No transactions are possible for a profit.
Approach
This problem can be solved using Dynamic Programming (DP) combined with Recursion and Memoization. Here's a step-by-step explanation:
Key Insights
- Divide the Problem:
- Find the maximum profit for one transaction before or up to day
i
. - Find the maximum profit for one transaction after or from day
i
. - Combine the two profits to find the global maximum.
- Find the maximum profit for one transaction before or up to day
- State Representation:
i
: Current day (0 to n-1).t
: Number of completed transactions (0, 1, or 2).can_buy
: A flag indicating if you can buy (1 = buy allowed, 0 = sell allowed).
- Base Case:
- If
i == n
(past the last day) ort == 2
(completed two transactions), the profit is0
.
- If
- State Transition:
- If
can_buy == 1
: Choose to buy or skip.profit = max(-prices[i] + dfs(i+1, t, 0), dfs(i+1, t, 1))
- If
can_buy == 0
: Choose to sell or skip.profit = max(prices[i] + dfs(i+1, t+1, 1), dfs(i+1, t, 0))
- If
Code Implementation
def maxProfit(prices):
n = len(prices)
memo = {}
# Recursive helper function
def dfs(day, transactions, can_buy):
# Base cases
if day == n or transactions == 2:
return 0
if (day, transactions, can_buy) in memo:
return memo[(day, transactions, can_buy)]
# Skip current day
profit = dfs(day + 1, transactions, can_buy)
if can_buy:
# Buy or skip
profit = max(profit, -prices[day] + dfs(day + 1, transactions, 0))
else:
# Sell or skip
profit = max(profit, prices[day] + dfs(day + 1, transactions + 1, 1))
# Memoize and return result
memo[(day, transactions, can_buy)] = profit
return profit
return dfs(0, 0, 1)
Interview Simulation
Interviewer Question 1: How did you divide the problem?
Candidate Answer:
"The problem is split into two phases: finding the maximum profit before a given day and finding the maximum profit after that day. Combining these two gives the total profit. The DP approach efficiently handles overlapping subproblems using memoization."
Interviewer Question 2: How would this algorithm change if we allowed unlimited transactions?
Candidate Answer:
"For unlimited transactions, we wouldn’t limit transactions
to 2. Instead, we’d iterate through the days while tracking the profit. The state would then depend only on whether we can buy or sell, reducing the complexity."
Interviewer Question 3: Can you optimize space usage?
Candidate Answer:
"Yes, instead of storing results for all days, we can use a rolling array to store only the results of the current and previous days, thereby reducing space complexity from O(n*2*3)
to O(2*3)
."
Example Walkthrough
- Input:
prices = [3,3,5,0,0,3,1,4]
- Day 4: Buy (price = 0).
- Day 6: Sell (price = 3), profit = 3.
- Day 7: Buy (price = 1).
- Day 8: Sell (price = 4), profit = 3.
- Total profit = 6.
- Input:
prices = [7,6,4,3,1]
- No transactions are made; profit = 0.
Challenges and CSOAhelp’s Role
- Dynamic Problem-Solving: Candidates often struggle with recursion and memoization. CSOAhelp simplifies these concepts with step-by-step examples and detailed explanations.
- Mock Interviews: By simulating the actual interview process and providing detailed prompts, CSOAhelp prepares candidates to handle tough questions confidently.
- Time Complexity Optimization: Our hints help candidates avoid redundant calculations and explain their approach clearly to interviewers.
With CSOAhelp, you’re never alone in tackling challenging problems like this. Prepare smarter, ace your interviews, and land your dream role!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
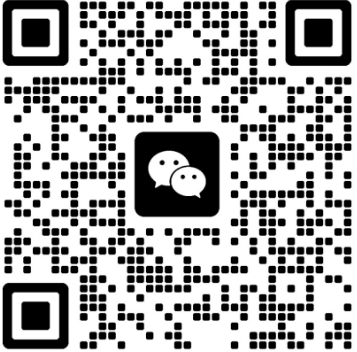