Stripe Authorization Request Processing
Stripe accepts payments on behalf of merchants. These payments begin as Authorization Requests, which represent an intent to transfer funds from an end user's credit card to a merchant. The system receives these requests and decides the Outcome of each Authorization Request. For now, the system's policy is to APPROVE all requests.
Authorization Requests Format
Authorization requests arrive in the following format:
timestamp_seconds,unique_id,amount,card_number,merchant
Input Example
5,R1,5.60,4242424242424242,bobs_burgers
10,R2,500.00,4242111111111111,a_corp
Specifications
- Data Rules:
- No data fields in this file will include commas or whitespace surrounding the field values.
- Field values are limited to alphanumeric characters, underscores, and periods.
- The input is pre-validated for correctness.
- Each
timestamp
is a positive integer (in seconds). - Each
amount
is a decimal value greater than zero.
- Output Format:
- Generate a human-readable report including the following fields:sql复制代码
timestamp unique_id amount outcome
- Fields should be separated by spaces.
- Generate a human-readable report including the following fields:sql复制代码
Output Example
5 R1 5.60 APPROVE
10 R2 500.00 APPROVE
Task
Write a function that:
- Produces a report for all requests in the described format.
- Ensures the requests are ordered chronologically by
timestamp
.
Additionally, write tests to demonstrate the correctness of your solution.
问题分析
该问题可以分为三个主要部分:
- 数据解析:解析输入数据,将每行数据拆解为对应字段并存储。
- 数据排序:根据时间戳
timestamp
对解析后的请求进行排序,确保输出结果的顺序正确。 - 格式化输出:将每条记录按照要求生成易读的输出格式,并附加处理结果
APPROVE
。
解决方案详细步骤
1. 数据解析
任务目标:
从输入中提取字段信息,并将其存储为结构化的数据。
步骤:
- 遍历输入的每一行,按逗号分割字段。
- 将每个字段解析为对应的变量:
timestamp
:时间戳,转为整数。unique_id
:请求标识符,保持原字符串格式。amount
:金额,转为浮点数。card_number
:信用卡号。merchant
:商户名称。
- 将解析结果存储为列表中的字典对象,方便后续操作。
示例解析:
plaintext复制代码输入行:5,R1,5.60,4242424242424242,bobs_burgers
解析结果:
{
"timestamp": 5,
"unique_id": "R1",
"amount": 5.60,
"card_number": "4242424242424242",
"merchant": "bobs_burgers"
}
2. 数据排序
任务目标:
按照时间戳 timestamp
对所有记录进行升序排序。
步骤:
- 使用排序函数
sorted()
,指定以timestamp
字段为排序键。 - 确保排序结果稳定,以防同一时间戳的记录出现乱序。
排序结果:
输入记录:
[
{"timestamp": 10, "unique_id": "R2", "amount": 500.00, ...},
{"timestamp": 5, "unique_id": "R1", "amount": 5.60, ...}
]
排序后:
[
{"timestamp": 5, "unique_id": "R1", "amount": 5.60, ...},
{"timestamp": 10, "unique_id": "R2", "amount": 500.00, ...}
]
3. 格式化输出
任务目标:
将每条记录格式化为指定的输出格式,并添加 APPROVE
结果字段。
步骤:
- 遍历排序后的记录。
- 对每条记录,按以下模板生成输出字符串:plaintext复制代码
"{timestamp} {unique_id} {amount:.2f} APPROVE"
- 使用
{:.2f}
格式化金额,确保输出两位小数。
- 使用
- 将生成的输出字符串逐行存储。
示例输出:
5 R1 5.60 APPROVE
10 R2 500.00 APPROVE
时间与空间复杂度分析
时间复杂度:
- 数据解析: 每行解析一次,时间复杂度为
O(n)
。 - 排序: 基于时间戳排序,时间复杂度为
O(n log n)
。 - 格式化输出: 遍历记录生成字符串,时间复杂度为
O(n)
。
综合时间复杂度:O(n log n)。
空间复杂度:
- 使用额外的列表存储解析后的记录,空间复杂度为 O(n)。
示例解析
输入:
5,R1,5.60,4242424242424242,bobs_burgers
10,R2,500.00,4242111111111111,a_corp
解析过程:
[
{"timestamp": 5, "unique_id": "R1", "amount": 5.60, ...},
{"timestamp": 10, "unique_id": "R2", "amount": 500.00, ...}
]
排序:
[
{"timestamp": 5, "unique_id": "R1", "amount": 5.60, ...},
{"timestamp": 10, "unique_id": "R2", "amount": 500.00, ...}
]
输出:
5 R1 5.60 APPROVE
10 R2 500.00 APPROVE
CSOAHelp 提供的面试辅助
在候选人完成这道面试题的过程中,CSOAHelp 提供了以下帮助:
- 任务拆解与思路引导:
- 解释问题的逻辑结构,将任务拆分为解析、排序和格式化三步。
- 引导候选人选择合适的数据结构(如字典与列表)。
- 复杂度优化建议:
- 强调使用内置排序方法以提高效率。
- 提醒注意格式化金额输出中的小数点问题。
- 边界条件分析:
- 处理空输入数据的情况。
- 确保记录中没有重复的
unique_id
或无效时间戳。
总结
这道 Stripe 面试题不仅考查了候选人对数据处理与排序的能力,还要求能够生成清晰、正确的报告。在 CSOAHelp 的实时指导下,候选人高效完成任务,展示了优秀的算法设计与实现能力。
如果您也希望在面试中表现出色,欢迎联系 CSOAHelp!我们提供全面的面试辅导与实时支持服务,助您成功拿到心仪 Offer!
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
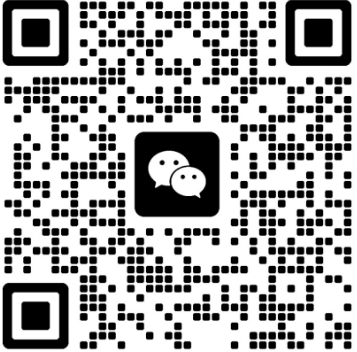