Background and Context
The interview question focuses on simulating an optimal parking strategy, where the aim is to maximize the minimum distance between parked cars. This is a classic optimization problem, often encountered in scenarios where resource allocation or spatial optimization is critical.
Problem Statement
You are given an array parkings
of size N
, which represents a parking lot. Each slot can either be:
- 0: An empty slot.
- 1: A slot already occupied.
You are also provided an integer K
, the number of cars to park. The task is to determine the maximum possible minimum distance between parked cars, leveraging the empty slots. The output should return this maximum minimum distance.
Key Constraints and Assumptions
- The array
parkings
only contains values0
and1
. - The integer
K
is always valid (0 ≤ K ≤ the number of0
s in the array). - The goal is to maximize the smallest distance between any two parked cars, ensuring equal distribution wherever possible.
Thought Process and Solution Outline
This problem combines binary search with greedy simulation. Here's the breakdown:
1. Understanding the Objective
The objective is to maximize the minimum distance. This naturally suggests a binary search on the result:
- Start with a possible range of distances:
low = 1
,high = N
(maximum distance). - The middle value (
mid
) in this range is tested as a potential minimum distance.
2. Greedy Validation
To verify if a given distance is feasible:
- Iterate through the parking array.
- Try to place
K
cars while ensuring each car is at leastmid
units apart from the previous one. - If it’s possible to place all
K
cars, the distance is valid. Otherwise, it’s not.
3. Binary Search for Optimization
Binary search adjusts the range based on the feasibility of the current mid
:
- If placing
K
cars atmid
distance is possible, search for a largermid
(maximize distance). - Otherwise, reduce the range by decreasing
high
.
Steps in Solution
- Initialize:
- Use two pointers:
low = 1
,high = N
. - Perform binary search until
low
exceedshigh
.
- Use two pointers:
- Validation Function:
- Implement a helper function to check if
K
cars can be placed with at leastmid
distance.
- Implement a helper function to check if
- Binary Search Logic:
- For every
mid
, validate feasibility using the helper function. - Adjust
low
orhigh
accordingly.
- For every
- Edge Cases:
- All slots are
0
: Place cars with uniform distribution. - Insufficient slots for
K
: Return 0 or handle as invalid input.
- All slots are
Insights from the Interview
During the interview discussion, the candidate was asked to reason about the binary search approach and explain why greedy validation works. The interviewer emphasized algorithm efficiency, particularly the O(N log N)
complexity due to the binary search over N
slots.
The dialogue also touched on practical considerations, such as:
- Why greedy placement ensures optimality.
- How binary search narrows down the solution space efficiently.
The candidate's ability to reason through these steps demonstrated proficiency in problem-solving and algorithmic thinking.
Conclusion
This problem is a variation of the "Aggressive Cows" problem, a popular competitive programming question. It evaluates a candidate's skills in:
- Formulating the problem mathematically.
- Applying binary search for optimization.
- Implementing and debugging algorithms efficiently.
This exercise also tests critical thinking and the ability to communicate solutions effectively during high-pressure interviews.
面试对话详解:深入剖析候选人逻辑能力
在初步阐述了解决问题的整体思路后,面试官开始对关键细节进行逐步提问,以测试候选人的算法细节理解和应变能力。以下是几轮交锋的对话记录与分析:
第一轮交锋:算法核心设计
面试官:
“你提到了使用二分搜索来确定最大最小距离。那么,为什么这个问题可以用二分搜索解决?它的单调性体现在哪里?”
候选人:
“二分搜索的核心是找到解空间中的一个mid
值,并验证它是否符合题目条件。对于这个问题,当我们选择一个mid
值作为最小距离时,如果可以满足条件,我们可以尝试更大的距离,因为所有比mid
更小的距离也一定是可行的。这体现了问题的单调性——当某个mid
值是可行解时,更小的值一定也是解。”
面试官反馈:
“解释清晰。单调性在此问题中是关键,它确保了二分搜索的有效性。接下来,你能详细描述如何验证一个mid
值是否可行吗?”
第二轮交锋:验证逻辑细化
候选人:
“验证mid
值是否可行可以通过贪心法实现。我们从第一个空车位开始,放置第一辆车,然后尽可能选择离上一辆车mid
距离之外的下一个空车位,直到放置所有K
辆车。如果放置完K
辆车时没有违反mid
的限制,这个mid
值就是可行的。”
面试官:
“对于贪心选择,你提到‘尽可能选择下一个满足条件的空位’。如果停车场很稀疏,比如大部分是1
,你如何有效跳过这些非空位来节省时间?”
候选人:
“可以直接跳过所有值为1
的索引,只在0
的索引处尝试放置车辆。这种方式能减少无效的遍历,保证验证逻辑的效率。”
面试官反馈:
“很好,这表明你理解了如何优化验证过程。但如果停车场大小非常大,比如N=10^6
,你认为这种方式的时间复杂度是合理的吗?”
第三轮交锋:复杂度分析与优化
候选人:
“验证函数的时间复杂度是O(N)
,主要由遍历数组决定。由于二分搜索的迭代次数是log(N)
,总复杂度是O(N log N)
,在N=10^6
的情况下,这种复杂度是可接受的。”
面试官:
“如果再进一步优化,你能想到其他方式吗?”
候选人:
“如果停车场的数据是稀疏的,可以将所有空车位的索引预先存储到一个数组中,然后在验证时仅对这些空位进行二分查找和判断,从而降低实际处理的索引数量。”
面试官反馈:
“非常好,这种方式能有效降低处理稀疏数据时的复杂度。接下来,我们看看你的解决方案在边界条件下的表现。”
第四轮交锋:边界条件的讨论
面试官:
“如果停车场只有一个空位且需要停车,你的算法如何处理?”
候选人:
“对于这种情况,K
不能大于空位数量。如果K=1
,结果就是停车场唯一的空位;如果K>1
,题目输入本身就不合法,可以直接返回错误或边界处理。”
面试官:
“那么,如果K=0
呢?”
候选人:
“这种情况下无需停车,直接返回Infinity
或其他特殊值表示‘不需要停车’。”
面试总结:考察的深度与广度
通过多轮交锋,面试官全面考察了候选人在以下几个方面的能力:
- 算法思维:是否能够从问题的本质出发,提出高效且符合逻辑的解法。
- 复杂度分析:能否合理分析时间和空间复杂度,并在面试官的引导下进一步优化。
- 边界条件处理:对异常输入和边界情况是否有足够的敏感性和应对方案。
- 沟通表达:能否在高压下清晰解释每一步逻辑,并及时调整应对面试官的追问。
候选人的表现与收获
这次面试充分展示了候选人扎实的算法功底与思维灵活性,同时也体现了充分准备和专业辅导的重要性。在这场面试中,候选人经过 CSOAHelp 的精心辅导,包括针对性的问题分析、优化思路的引导,以及 VO support 提供的实际模拟面试体验,才得以在面试官的多轮追问下从容应对,展现出完美的表现。
对于类似的问题,建议求职者在准备时多从以下几个角度入手:
- 理解问题的核心需求和潜在变种。
- 提前练习时间复杂度较高的边界场景。
- 熟悉常用的优化技巧,比如稀疏数据处理、预处理索引等。
借助 CSOAHelp 的支持,候选人在面试中不仅成功展示了自己的实力,还获得了面试官的高度认可,为未来的职业发展打下了坚实基础。
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
Candidate's Performance and Takeaways
This interview demonstrated the candidate's solid algorithmic skills and flexible thinking. It also highlighted the importance of thorough preparation and professional guidance. In this case, the candidate benefited significantly from CSOAHelp's tailored interview preparation, including in-depth problem analysis, optimization techniques, and realistic mock interview sessions provided through VO support. These resources enabled the candidate to confidently navigate multiple rounds of questioning and deliver a flawless performance.
Here is the detailed dialogue exchange in English:
Round 1: Algorithm Design Fundamentals
Interviewer:
“You mentioned using binary search to find the maximum minimum distance. Why does this problem lend itself to binary search? Where does monotonicity come into play?”
Candidate:
“Binary search works here because we are searching for the largest feasible mid
value (the minimum distance). If a certain mid
value is valid, all smaller values will also be valid, as they impose less restrictive conditions. This monotonic property ensures that binary search can effectively narrow down the solution space.”
Interviewer Feedback:
“Good explanation. The monotonicity is indeed the key. Now, can you describe in detail how you would validate a given mid
value?”
Round 2: Validation Logic
Candidate:
“To validate a mid
value, I would use a greedy approach. Starting from the first empty slot, I would place the first car there and attempt to place subsequent cars at least mid
units apart. If I can place all K
cars following this rule, the mid
value is valid; otherwise, it is not.”
Interviewer:
“In your greedy placement, you mentioned ‘choosing the next available slot.’ How would you handle sparse parking lots with many occupied slots (value 1
)?”
Candidate:
“To optimize the validation process, I would skip over all occupied slots (1
s) during iteration and only focus on empty slots (0
s). This ensures we minimize unnecessary operations and improve efficiency.”
Interviewer Feedback:
“Good optimization. Skipping occupied slots demonstrates your attention to efficiency. If the parking lot is extremely large, say N = 10^6
, do you think your approach is scalable?”
Round 3: Complexity Analysis and Further Optimization
Candidate:
“The validation function has a time complexity of O(N)
because it involves a single traversal of the parking lot. Since the binary search involves log N
iterations, the overall complexity is O(N log N)
. This is efficient enough for N = 10^6
.”
Interviewer:
“What if the parking lot data is sparse? Can you further optimize your approach?”
Candidate:
“In the case of sparse data, I can preprocess the indices of all empty slots into a separate array. During validation, I would perform binary search or direct index access within this array, reducing the effective size of the data being processed.”
Interviewer Feedback:
“That’s an excellent idea. Preprocessing can significantly reduce complexity for sparse data. Let’s move on to edge cases.”
Round 4: Edge Case Handling
Interviewer:
“If there is only one empty slot in the parking lot, how would your algorithm handle it?”
Candidate:
“If K
is greater than the number of empty slots, the input itself is invalid. If K = 1
, the result is simply the location of the only empty slot. This case would be handled at the input validation stage.”
Interviewer:
“What about the case where K = 0
?”
Candidate:
“In that scenario, no parking is required, so the function could return a placeholder value like Infinity
or simply indicate that no parking is necessary.”
Final Takeaways
Through multiple rounds of discussion, the interviewer thoroughly assessed the candidate’s abilities in:
- Algorithm Design: Crafting efficient and logical solutions to complex problems.
- Complexity Analysis: Evaluating and improving time and space complexity in practical scenarios.
- Edge Case Handling: Proactively considering and addressing boundary conditions.
- Communication Skills: Articulating solutions and reasoning clearly under pressure.
Thanks to the rigorous preparation provided by CSOAHelp's Interview Coaching and VO Support, the candidate excelled in this challenging interview. They confidently tackled each question, earning the interviewer’s praise and securing a solid opportunity for their future career. For aspiring candidates, this demonstrates the value of structured preparation and expert guidance.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
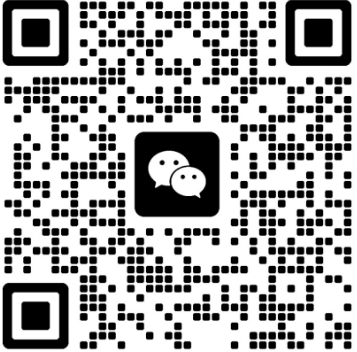