Problem 1: Split and Sort Array
Description
You are given an array A of N integers. You can split the array into two non-empty parts, left and right, sort the elements in each part independently and join them back together.
For example, given array A = [1, 3, 2, 4], you can split it in the following three ways:
- left = [1], right = [3, 2, 4]. Sorting the elements and joining the parts back together results in the array:
[1, 2, 3, 4]
. - left = [1, 3], right = [2, 4].
Resulting sorted and rejoined array:[1, 3, 2, 4]
. - left = [1, 3, 2], right = [4].
Resulting sorted and rejoined array:[1, 2, 3, 4]
.
Your task is to find the number of ways of splitting the array into two parts such that, after sorting the two parts and rejoining them into a single array, the resulting array will be sorted in non-decreasing order.
For the array shown above, the answer is 2: the first and third splits result in a sorted array.
Function Signature
class Solution {
public int solution(int[] A);
}
Given an array A of length N, returns the number of different ways of obtaining a sorted array by applying the procedure described above.
Examples
- Given A = [1, 3, 2, 4], the function should return 2, as explained above.
- Given A = [3, 2, 10, 9], the function should return 1.
The successful split is [3, 2], [10, 9]. After sorting and rejoining the parts you will receive[2, 3, 9, 10]
. - Given A = [5, 5, 5], the function should return 2.
The splits that result in a sorted array are:- left = [5], right = [5, 5]
- left = [5, 5], right = [5]
- Given A = [3, 1, 2], the function should return 0.
There are no splits that would result in a sorted array.
Constraints
- N is an integer within the range [2, 100,000]
- Each element of array A is an integer within the range [1, 1,000,000,000]
Problem 2: Minimize Absolute Sum
Description
There is an array A consisting of N integers. Choose at most one element to multiply by -1 in order to obtain an array whose sum of elements is as close to 0 as possible. That is, find the sum with the minimum absolute value.
Function Signature
int solution(vector<int> &A);
Given an array A, returns the minimum absolute value of the sum of A that can be obtained.
Examples
- For A = [1, 3, 2, 5], after multiplying the last element by -1, A will be equal to [1, 3, 2, -5].
Its sum is 1. It is not possible to obtain any sum closer to 0. The function should return1
. - For A = [-4, 0, -3, -3], we can multiply -4 by -1 and therefore obtain A = [4, 0, -3, -3].
Its sum is -2. The function should return2
. - Assume that A = [4, -3, 5, -7].
Its sum is -1. There is no possible move that could improve this result.
The function should return1
. - Assume that A = [-15, 18, -1, -1, 10, -22].
It is optimal to change -1 to 1. The function should return9
.
Constraints
- N is an integer within the range [1..100,000]
- Each element of array A is an integer within the range [-1,000..1,000]
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
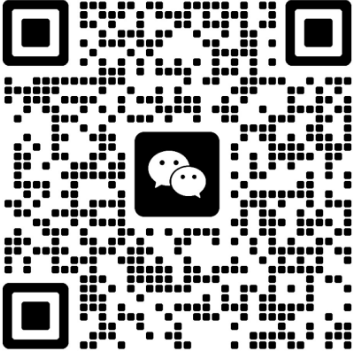