1. Filtration
Given a linked list, which contains a loop (the end node points to one of the nodes in the list), output the pointer to the node from where the loop starts.
Example
Let the input linked list be arr = [1, 2, 3, 4, 5]
, with length being n = 5
. Also, the last node points to node at loopIndex = 2
. Then, the list will look like:
1 → 2 → 3 → 4 → 5
↑ ↓
← ← ←
The function should return a pointer to the node with value 3
in this case.
Function Description
Complete the function filter
in the editor below.
filter
has the following parameter(s):
L
: aLinkedListNode
pointer to the head of the singly-linked listL
Returns
x
: aLinkedListNode
pointer to the head of the loop
Constraints
1 ≤ n ≤ 100
- The values in the linked list nodes will be in the range
0
to10000
inclusive 0 ≤ loopIndex < n
- Values in nodes can be repeated
3. Delete Nodes Greater Than X
Given a singly linked list and an integer, x
, remove nodes greater than x
.
Example
List = 100 → 105 → 50
x = 100
List becomes 100 → 50
Return a reference to the root node of the list after removing 105
.
Function Description
Complete the function removeNodes
in the editor with the following parameter(s):
node listHead
: a reference to the root node of the singly-linked listint x
: the maximum value to be included in the returned singly-linked list
Returns
node
: a reference to the root node of the final list
Constraints
1 ≤ n, x ≤ 10^5
1 ≤ SinglyLinkedListNode values ≤ 10^5
4. Condensed List
Given a list of integers, remove all nodes containing values that have appeared earlier in the list, and return a reference to the head of the modified list.
For instance, in the following list, the value 3
appears as a duplicate initially:
Linked List:
3 → 4 → 3 → 6
Redundant nodes are labeled 3
After calling condense:
3 → 4 → 6
Remove the node at position 2
in the list above, 0-based indexing.
Function Description
Complete the function condense
in the editor with the following parameters:
head
: the head of a singly-linked list of integers, aLinkedListNode
Note
A LinkedListNode
has two attributes:
data
: an integernext
: a reference to the next item in the list or the language equivalent ofnull
at the tail
Returns
reference to LinkedListNode
: the head of the list of distinct values
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
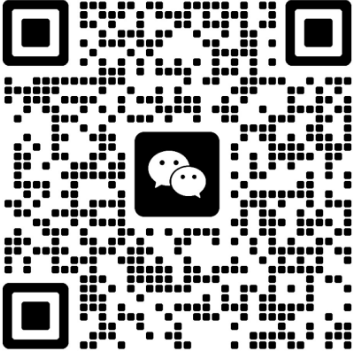