1. this Keyword Usage
What does the this
or self
keyword refer to in context of Object Oriented Programming?
Pick ONE option
- A reference to the current object.
- The superclass constructor.
- A reference to the class itself.
2. Efficient Data Search with Limited Memory
In a low-memory device, you need to search for a target element in a sorted array. Choose the best approach.
Pick ONE option
- LinearSearch(array, target)
- BinarySearchRecursive(array, target)
- BinarySearchIterative(array, target)
3. Optimal Cache Eviction Strategy Implementation
Your application cache needs to evict items to free up space when full. To optimize for performance, the least recently used item should be evicted. Which pseudocode snippet correctly implements a least-recently-used (LRU) cache eviction strategy?
Pick ONE option
- initialize array cache
if cache is full:
remove first element
add new item to cache - initialize queue cache
if cache is full:
dequeue an element
enqueue new item to cache - initialize doubly linked list cache
with hashmap lookup
4. Dynamic Range Query for Stock Price Analysis
You are analyzing stock prices and need to perform dynamic range queries to get the minimum stock price.
- Updates: Modifying the stock price at a specific date.
- Efficient range queries: Finding the minimum stock price in a given date range.
Which data structure and pseudocode correctly supports efficient range minimum queries and updates?
Pick ONE option
- initialize array prices
for each query:
update value at given index
find minimum by iterating through subarray within range - initialize binary search tree prices
for each query:
update value at given index
traverse tree within range and find minimum - initialize segment tree prices
for each query:
update value at given index
query tree for minimum in range
5. User Age Validation
Write a function validateAge
that returns true
if the age
argument is between 18 and 65 inclusive.
function validateAge(age) {
return <code>
}
Pick ONE option
return age >= 18 && age <= 65
return age < 18 || age > 65
return age >= 18 || age <= 65
6. Equalize Server Latency
Problem Description
TikTok's server network is structured as a perfect binary tree with n
servers where servers are numbered from 0
to n - 1
. Each server is connected to its parent with the following configuration:
- The parent of server
i
is serverfloor((i - 1) / 2)
, fori ≥ 1
. Here,floor(x)
denotes the greatest integer less than or equal tox
. - The children of server
i
are the servers numbered2 * i + 1
and2 * i + 2
, if they exist. - The root server (server 0) handles requests, which are passed down to its children.
- The latency cost between server
i
and its parent is defined as the time taken for data to travel along the edge between them and is given bylatency[i - 1]
fori ≥ 1
. - The latency from the root server to any leaf server is the sum of the latencies along the path from the root to that leaf.
Goal
Ensure that the latency from the root to every leaf server is the same.
You are allowed to increase the latency of some connections but cannot decrease any latency.
Find the minimum amount of additional latency needed to equalize the latency from the root to all leaf servers.
7. TikTok Task Chain Balancer
Problem Description
On the TikTok platform, various tasks such as video processing, content moderation, and recommendation generation are represented as a sequence of jobs, where each job has a processing cost given by the array taskCosts
.
To optimize resource usage, the system needs to find the smallest subarray in which a subsequence whose combined processing cost equals a given value k
. If no such subsequence exists, return -1.
Note
- A subarray of an array is defined as any contiguous segment of the array.
- A subsequence of an array is a sequence that can be derived from the array by deleting some or no elements without changing the order of the remaining elements.
Example
Given:
n = 8
k = 100
taskCosts = [12, 42, 11, 2, 28, 6, 61, 88]
Some of the task subarrays containing a subsequence with combined processing cost k
:
- Tasks 3 to 7:
[11, 2, 28, 6, 61] # choosing subsequence [11, 28, 61] → 11 + 28 + 61 = 100 = k
- Tasks 1 to 7:
[12, 42, 11, 2, 28, 6, 61] # choosing subsequence [11, 28, 61] → 100 = k
- Tasks 1 to 8:
[12, 42, 11, 2, 28, 6, 61, 88] # choosing subsequence [11, 28, 61] → 100 = k
- Tasks 2 to 7:
[42, 11, 2, 28, 6, 61] # choosing subsequence [11, 28, 61] → 100 = k
- Tasks 3 to 8:
[11, 2, 28, 6, 61, 88] # choosing subsequence [11, 28, 61] → 100 = k
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
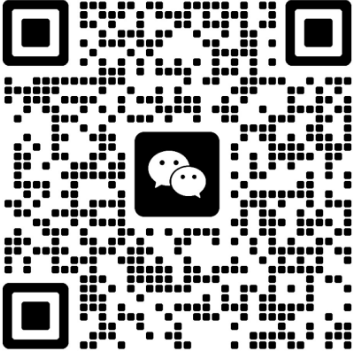