1. Question 1
You are given the first 2^N integers, of which K are "special".
Your task is to remove all the integers from the array with the minimum possible cost, following these rules:
Removal Rules
1.
If a segment contains L integers, and X of them are special, the cost of removing this segment is calculated as:
Cost = L × X × P, where P is a constant.
2.
If a segment contains L integers, and none are special, the cost of removing this segment is:
Cost = Q, where Q is another constant.
3.
If a segment contains L integers and L is divisible by 2, you have two options:
Option 1: Use either of the rules above to remove the segment as is
Option 2: Divide the segment into two smaller segments, each containing L/2 integers, and
recursively find the removal cost for both parts separately, then add the costs together.
Find the minimum cost of removing all 2^N integers, modulo (10^9 + 7).
NOTE: The integers must remain in their original order while dividing the current part into two smaller parts. If the current part contains integers [1, 2, 3, 4], the only correct way to divide it is [1, 2] and [3, 4].
Example
N = 2
P = 2
Q = 1
special_integers = [1, 3]
Start with the first 2^N integers: [1, 2, 3, 4] in which integers 1 and 3 are special, shown in bold.
2. Question 2
A permutation P is called unstable if it keeps changing every second based on the rule below.
- Every element of the permutation is changing every second independently following a rule, i.e., after one second every P[i] becomes P[P[i]]
Given a permutation, find the minimum time after which it will become stable. If it will never become stable, return -1.
Example
Given N = 3 and P = [3,2,1],
In one second it will become P[P[3]], P[P[2]], P[P[1]] = [1, 2, 3].
The permutation [1,2,3] is stable as it will not change further after applying the same rule again.
Function Description
Complete the function solve
in the editor below. The function should return a single integer as stated above.
solve
has the following parameter:
- P[1]...P[n]: an array of integers
Constraints
- 1 ≤ n ≤ 10⁵
- 1 ≤ P[i] ≤ n
Input Format For Custom Testing
The first line contains an integer n that denotes the length of permutation.
The second line contains permutation P of n distinct space-separated integers.
Sample Case 0
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
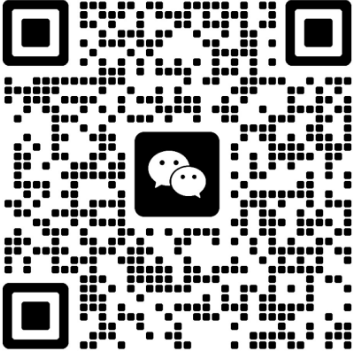