Think tech interviews are just about algorithms? Think again. At Meta (formerly Facebook), even if you’ve practiced LeetCode to death, your interview can crash and burn if you freeze up or can’t communicate your thoughts clearly. Especially when the interviewer starts with something that sounds simple — like “You have m sorted arrays, total length n. Find the kth smallest element.” Sounds easy, but it’s a landmine.
Here’s a real story from a candidate we at CSOAHELP recently supported in their Meta technical interview. He’s not a coding competition veteran or a serial big-tech offer holder. But with our real-time remote interview assistance, he stayed calm, hit every critical point, and passed the round. We supported him silently throughout the session — offering well-timed code hints and structured responses that helped him navigate every tricky moment.
Five minutes into small talk, the Meta interviewer suddenly dropped the question, with no warm-up:
You have m arrays of sorted integers. The sum of the array lengths is n. Find the kth smallest value of all the values.
At first glance, this looks like a simple heap problem. But overlook one tiny part, and you’ll spiral. We’d already anticipated this kind of prompt, so as soon as the candidate heard it, we pushed our first screen-side message: “Clarify a few things — k is 1-based, right? Can we assume k ≤ total number of elements? Can any of the arrays be empty? Do we need to handle edge cases?”
These clarifying questions earned the candidate some precious breathing room — and earned the interviewer’s approval. The response came quickly: “Yes, k starts at 1. Input is always valid. No need to handle empty arrays.”
The candidate moved to the solution phase, and we synced up with a new message: “First propose the brute-force approach: merge all arrays and sort. Time complexity is O(n log n). But immediately explain: this isn’t scalable in production — optimization is needed.” Then we provided the optimized approach via min-heap: “Build a min-heap initialized with the first element of each array. Maintain a tuple (value, array_index, element_index). On every pop, push the next element from that array if available. After k pops, you’ll have the kth smallest.”
The candidate stumbled slightly when explaining this logic. We immediately supplemented with a concise line: “This way, you always consider the smallest current values across all arrays. Time complexity becomes O(k log m), space O(m). Ideal for merging sorted arrays at scale.” The interviewer was clearly impressed.
Then came the code portion. We pushed a prewritten Python function to the candidate’s secondary screen:
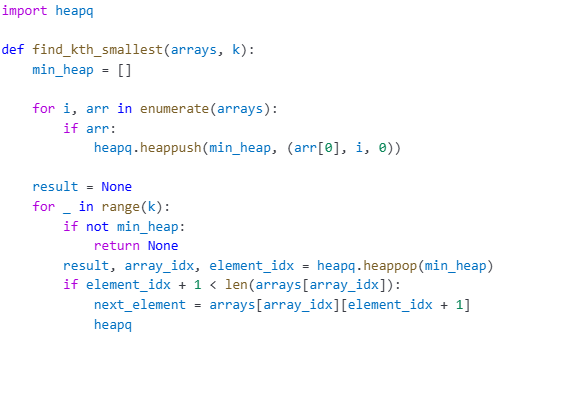
As he typed it out, he followed our guidance to narrate each step: why tuples are used, how the heap remains valid, and why this logic ensures correctness. The interviewer nodded consistently.
Once the code was complete, the interviewer immediately asked: “Can you explain the time and space complexity?” We had already prepared a clean and complete response and pushed it instantly: “Initial heap size is m → O(m). Each pop/push is O(log m), repeated k times → O(k log m). Heap holds at most m elements → space is O(m).” The candidate repeated it confidently and nailed the core question.
Then came the classic Meta follow-up: “Walk me through an example.” The candidate tensed up, so we pushed a walkthrough immediately:
“Let’s say arrays = [[1,3,5,7], [2,4,6], [8,9]], k = 5. Initial heap = [(1,0,0), (2,1,0), (8,2,0)]. 1st pop → 1, add (3,0,1). 2nd pop → 2, add (4,1,1). 3rd pop → 3, add (5,0,2). 4th pop → 4, add (6,1,2). 5th pop → 5, answer = 5.”
The candidate explained it linearly, showcasing the evolving heap. The interviewer smiled and said, “Very clear.”
At the end, the interviewer asked: “What did you learn from this problem?” A lot of people flounder here. We pushed a perfect closing line: “This reinforced how heap structure — though basic — can solve real-world scaling problems when used precisely. For merging sorted arrays, it’s incredibly effective. There’s room for improvement too, like handling duplicates or limiting output size.”
That statement wrapped things up perfectly. The interviewer’s final comment: “Great performance today — your explanations were very structured.”
The candidate later advanced to the system design round. He told us: “I’m not a strong algorithms person. I blank out when nervous. If you hadn’t been there feeding me what to say, helping connect the dots, and dropping code blocks when I froze, I would’ve bombed.”
That’s exactly what CSOAHELP is for — not to cheat or spoon-feed, but to make sure you have the right info at the right moment. Even if you’re not a star coder, we help you present like one. From clarifying questions to walkthroughs to time complexity breakdowns, we prep and deliver what you need — and all you need is to read, repeat, and stay calm.
What makes Meta interviews hard isn’t the questions. It’s having to demonstrate clear logic, complete structure, and smooth communication — all within 40 minutes. If you’ve got someone beside you whispering, “Mention this now,” or “Use this code snippet,” or “Don’t forget this edge case,” you’ll turn a scary question into a performance.
If you’re targeting Meta, Google, Stripe, or Apple — and you’re worried about blanking out under pressure — check out our real-time interview assistance. No matter your level, we’ll help you walk into that call as your best self.
Meta doesn’t just hire the smartest people — it hires the best prepared.
Let us help you be that person.
Message us to see how we can help you cross the finish line.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
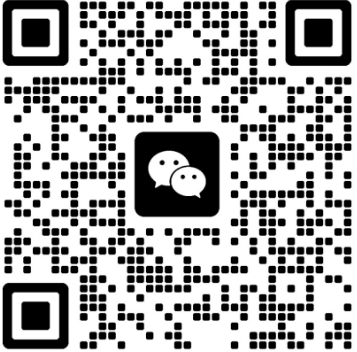