"I could’ve solved this on my own—my mind just went blank under pressure, the time was tight, and the interviewer was watching. I ended up spiraling."
That's what one of our clients said after completing a technical interview with Bloomberg.
The issue wasn't ability. It was the lack of real-time support when it mattered most.
That’s where CSOAHELP comes in. We're here to ensure you’re never alone in a live interview. With a seasoned technical support team quietly assisting you in real time, we help you move past anxiety and perform at your best.
Let’s walk through a real Bloomberg interview scenario where our support helped a candidate successfully land a second-round invitation.
On the interview day, the candidate joined the Zoom call on time. The Bloomberg engineer, known for being to-the-point, skipped the small talk and immediately shared the problem:
"There are a number of CSV files scattered throughout a folder and its subfolders, containing information about trades. Implement the function
process_path
which takes a path to the folder and finds all of the CSV files and sum all of the values in the second column. The second column always contains integers."
At first glance, it doesn’t seem difficult—file traversal, content reading, parsing, and summing. But as with most real interviews, the challenge isn’t whether you can do it, but whether you can do it calmly and correctly under pressure, with clean logic and solid edge-case handling.
The candidate had the right idea—directory traversal combined with CSV parsing. But he stalled right away: "Should I use os.walk or glob? Or write my own recursion?"
We immediately provided a tip via the secondary screen: os.walk is the standard for recursive directory traversal. Combine it with os.path.join to build full paths. For reading CSVs, use Python's built-in csv.reader, read each line, and convert row[1] to an integer for summing.
With that guidance, he started coding. But another common mistake came up. He correctly checked:
if file.endswith('.csv')
—but opened the file with:
with open(file, 'r') as f:
This would throw an error, because file is just the name—not the full path.
We stepped in: file is just the filename. You need os.path.join(root, file) to get the full path.
He quickly updated it:
filepath = os.path.join(root, file)
with open(filepath, 'r') as f:
The core logic was coming together, but he hesitated again: "What if there's an empty row? Should I check that? And what if there's a header—should I skip the first row?"
We followed up: Add a guard clause like if not row or len(row) < 2
to skip malformed lines. If you’re worried about headers, use next(reader)
to skip the first line. Since the prompt doesn’t specify headers, you can mention your assumptions or leave a comment explaining it.
These weren’t just technical hints. They showed the interviewer that the candidate thought like a real engineer—handling data quality and ambiguous specs thoughtfully.
Eventually, he arrived at a complete, well-structured solution:
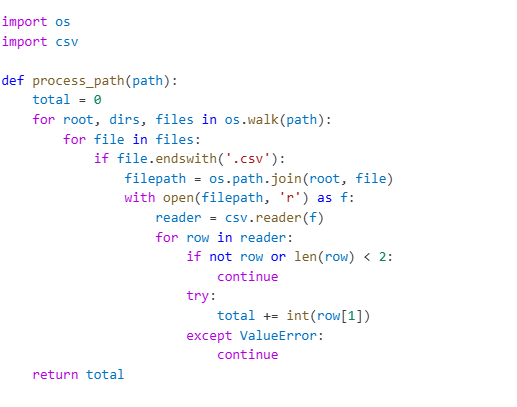
When it came time to explain his solution, he was clearly nervous. We sent over a structured breakdown he could use: Start with how os.walk was used for directory recursion, then os.path.join for building full paths. Mention how csv.reader handled the reading and row[1] was summed. Point out the exception handling for robustness.
He repeated this almost verbatim, and the interviewer nodded, clearly satisfied with the clear, logical thought process.
That’s when the follow-up questions started coming.
"What if the CSV format isn’t consistent and the second column isn’t always an integer?"
We advised: Emphasize that try...except handles ValueError to prevent crashes from badly formatted rows.
"Can this be parallelized? What if there are tens of thousands of files?"
We suggested: Acknowledge that IO is the bottleneck. Consider multithreading or async IO using concurrent.futures or asyncio—but only if files are independently readable.
"Is there a more robust way to access the second column than using row[1]?"
We explained: If there’s a header, use DictReader to access fields by column name. He added, “If the format is guaranteed, my way is simpler—but for inconsistent formats, DictReader is safer.”
The interviewer nodded. It was clear that these thoughtful responses gave the impression of a competent and adaptable developer.
He passed and was invited to the next round: system design.
Afterward, he admitted, “I’m not that fast. I forget stuff. Without your help in the background, I would’ve blanked.”
That’s what CSOAHELP offers. It’s like spinning up a second brain while your main thread handles the interview.
We’re not here to cheat the process—we’re here to help you present what you already know in the best, clearest way possible.
We offer:
Real-time written guidance: Where to start, what tools to use, how to structure your solution. Code snippet suggestions: If you stall, we give you clean templates to adapt or reuse. Explanation phrasing: We prep you to clearly and confidently explain your thought process. Robustness reminders: We highlight edge cases, data issues, and performance considerations you might forget under pressure. If you're preparing for interviews at Bloomberg, Google, Stripe, Amazon, or any company that expects more than just code—they want clarity, completeness, and communication—our remote live-assist service can help you deliver.
You're not alone. Let us be your second mind during high-pressure interviews.
Interviews are no longer just about solving problems—they’re about how you handle problems. Smart preparation means getting support when it counts.
Curious how we can help? Reach out or share your own interview challenge. Maybe the next success story will be yours.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
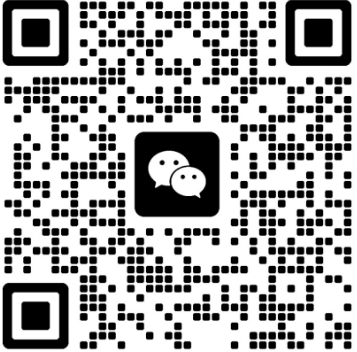