In early March, a candidate preparing for a Google SDE interview reached out to us. He had done plenty of LeetCode, had decent system design prep, but remained deeply concerned about handling the pressure of live problem-solving, communicating clearly, and managing unexpected question types. He said it plainly: "I can write code, but Google interviewers love to drill into your logic step by step. If I lose my rhythm, I freeze." This is a common situation for strong candidates—not a lack of skill, but fear of stumbling in the moment.
So he opted for CSOAHELP's real-time remote interview assistance. We walked him through device setup, did a dry run of the interview process, and customized a guidance playbook based on Google’s usual question styles. When the actual interview arrived, he stumbled out of the gate but managed to stabilize and answer fluently—earning a pass to the next round of system design.
The technical interview question was as follows:
An arithmetic sequence is a list of numbers with a definite pattern. If you take any number in the sequence then subtract it from the previous one, the difference is always a constant. A good arithmetic sequence is an arithmetic sequence with a common difference of either 1 or -1. For example, [4, 5, 6] is a good arithmetic sequence. So is [6, 5, 4], [10, 9], or [-3, -2, -1]. But, [1, 2, 1] (no common difference) or [3, 7] (common difference is 4) is NOT. Implied, any sequence that has only one element is a good arithmetic sequence. Given an integer array nums, return the sum of the sums of each subarray that is a good arithmetic sequence.
At first glance, it seemed manageable—some array traversal, subarray checks, summation. But the follow-up questions quickly threw the candidate off. The interviewer asked, "Let’s take it step by step—how would you check if a subarray fits this rule?"
We immediately pushed a complete guidance snippet to the candidate's second screen, so he could simply repeat it: "I’d start with brute-force enumeration of all subarrays, beginning with length 1. For each, I would check whether the subarray forms a valid arithmetic sequence where the difference between consecutive elements is either 1 or -1. If it qualifies, I calculate its sum and add it to the total."
The candidate repeated this with slight elaboration. The interviewer nodded, and he started regaining confidence.
Next, the interviewer asked, "How would you efficiently check if a subarray is a good arithmetic sequence?" Again, we sent a ready-to-use prompt: define a helper function is_good_subarray(start, end). If the length is 1, return True. If it’s 2 or more, calculate the first difference as diff = nums[start+1] - nums[start], then check whether each subsequent nums[i+1] - nums[i] equals diff, assuming diff is either 1 or -1.
The candidate essentially repeated the explanation verbatim and began writing out pseudocode. The interviewer followed with another question: "How does it perform? Any room for optimization?" This is often where candidates freeze, but we guided him again. Brute-force is O(n^2), acceptable for small input sizes. If time permits, mention optimizations like sliding window extension of valid ranges, and early termination (break) when the sequence breaks pattern.
Just by following our suggestions, he appeared composed. The interviewer then said, "Let’s see some code." We provided a clean framework to build from. He read and adapted it on the fly:
def sum_good_arithmetic_subarrays(nums):
n = len(nums)
total = 0
for i in range(n):
total += nums[i]
for j in range(i + 1, n):
if abs(nums[j] - nums[j - 1]) == 1:
total += sum(nums[i:j+1])
else:
break
return total
With minimal tweaks, the candidate completed the implementation and confidently explained how it worked, the checking logic, and its time complexity. Though he didn’t independently propose optimizations or deep insights, CSOAHELP’s real-time support allowed him to deliver a full, coherent, and technically sound answer under pressure.
The heart of this interview wasn’t writing perfect code—it was staying logical and clear-headed under stress. That’s where our service shines.
For companies like Google, which prioritize reasoning, communication, and process over rote output, real-time remote support makes a huge difference. Not everyone has polished live expression or on-the-spot logic structuring skills. CSOAHELP ensures your real capabilities aren’t buried under nerves.
After the interview, the candidate told us, "I thought Google interviews were hard because of the questions. Turns out they’re hard because you need to talk through your thinking like an engineer." He realized his actual weak point wasn’t technical—it was in conveying what he knew.
That’s exactly what CSOAHELP remote interview assistance is for. At every key step, we provide complete prompts so you can focus on delivering smooth, logical answers. We don’t answer for you. We don’t cross lines. But we do get you across the finish line.
If you're preparing for interviews at Google, Apple, Stripe, or other top tech companies, stop struggling through it alone. Choose CSOAHELP, and let us help you bring out your best, every time.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
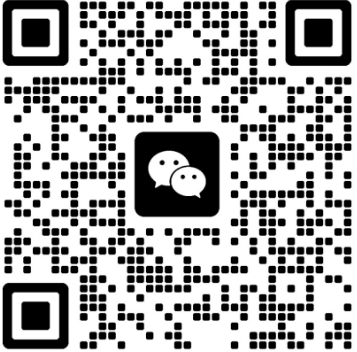