At CSOAHELP, we’ve supported candidates through countless interview scenarios—some heavy on algorithms, others focused on system design. But occasionally, we encounter challenges that blur the line between interview and real-world engineering. Waabi's "Speedup NeRF" task is a prime example. It wasn’t about solving a theoretical problem—it was a stripped-down research project. One where clear thinking under pressure, structured code, and a bit of real-time support made all the difference.
This article documents a real case where we helped a candidate tackle this challenge head-on: optimizing NeRF rendering from 2 hours down to under 5 minutes—without sacrificing output quality. It wasn’t a single function to tweak, but a dense, performance-sensitive pipeline.
The interview problem stated:
Speedup NeRF Here we give you a vanilla NeRF implementation (~100 lines of code) Your task: Make it faster! Goal is PSNR > 20 in 5 mins (original takes 2 hours) You’ll need to understand the code, implement a PyTorch model, debug and potentially do some parameter tuning Instructions: Briefly go over the code. Take care of the forward method in NerfModel Reimplement the forward method in FastNerfModel Some extra info: Volume AABB is -1.5 to 1.5 Suggested approach: Use volume representation (feature volume CxDxHxW) plus a small MLP You are welcome to explore Hashtable, Triplane, or other ideas, but they might be trickier to do in an hour
From the moment the interview began, the candidate was overwhelmed. The problem was straightforward on the surface, but the provided code—though compact—packed in dense sampling logic, MLP invocation, and volumetric rendering. The candidate had expected traditional algorithm or system design problems, but this real-time optimization task was a different beast.
Our CSOAHELP team immediately activated our remote live support, silently monitoring the interview session and feeding structured text prompts. We first recommended replacing the traditional MLP-heavy pipeline with a voxel-based feature grid. Each voxel would hold a low-dimensional feature vector, and trilinear interpolation would allow spatial sampling. These sampled features would then be passed to a lightweight MLP to produce RGB and density outputs. With that high-level architecture in mind, the candidate calmed down and started explaining their plan to the interviewer: replace dense sampling with a structured feature volume and a compact MLP decoder—striking a balance between quality and performance within memory constraints.
Then came the coding phase—the real test. The candidate had moderate familiarity with PyTorch, but struggled especially with 3D grid sampling. That’s where we stepped in with key code snippets. For initializing the feature grid:
self.feature_grid = nn.Parameter(torch.randn(C, D, H, W) * 0.1)
And for trilinear interpolation:
def interpolate_features(self, coords): coords = coords.view(1, 1, -1, 3) features = F.grid_sample(self.feature_grid.unsqueeze(0), coords, align_corners=True) return features.squeeze().permute(1, 0)
With those in place, the candidate simply adapted the code, followed our reminder to normalize the input to AABB, and moved on. We then pushed debugging suggestions: checking coordinate scaling, verifying output dimensions, and printing intermediate tensors to avoid common shape mismatches in 3D data.
Once the implementation was running, the interviewer shifted into follow-up mode. First came the question of spatial resolution: “Why 32x32x32? Random guess?” We immediately suggested a tradeoff-driven justification. The candidate followed our guidance: “I chose 32x32x32 because it's a sweet spot within a 2GB memory budget while maintaining enough spatial resolution. In production, I’d profile different grid sizes to balance speed and accuracy.”
Next came the question on quality control: “How do you ensure PSNR stays above 20?” We recommended a training-centric answer, touching on early stopping, smooth loss curves, and avoiding overfitting. The candidate responded: “During training, I monitor PSNR live. If performance degrades, I adjust the MLP structure or sampling rate. I use a warmup phase with low learning rates and keep the MLP compact—two layers, 64 units each—to minimize noise.”
Then came a tougher follow-up: “Can this structure batch render efficiently?” Our cue: highlight voxel-grid parallelism and GPU optimizations. The candidate replied: “Since each ray is independent during inference, this setup naturally supports batch rendering. It’s easy to scale across views and also allows export to ONNX or other formats for deployment.”
While the candidate didn't have all the answers independently, with our layered live support—from architecture suggestions to line-by-line code and reasoning aids—they were able to clearly and confidently present a complete, production-ready approach. The result: they passed the round and moved on to the system design phase at Waabi.
This interview highlights one truth: real engineering interviews, especially in AI, graphics, or vision fields, have moved far beyond solving puzzles. They are mini-projects—compressed reality checks. That’s exactly what CSOAHELP’s remote support is built for. We don’t answer for you—we coach you live. We deliver strategy, implementation scaffolding, and verbal reasoning guides in real-time. Even if you’re only halfway prepared, we help you perform like you’re ready for anything.
If you're walking into an interview where you're expected to debug, design, and explain under pressure—don’t go in alone. With CSOAHELP, you get a tactical coach, a backup brain, and a clear voice in your ear. You still own your performance—but we’ll make sure it’s your best.
Would you rather go in solo, or with a world-class team quietly supporting you?
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
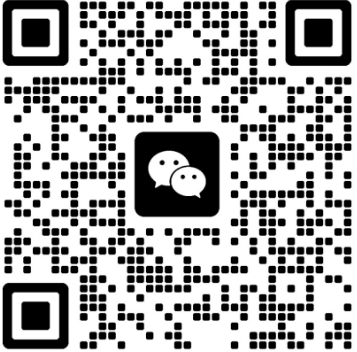