In tech communities like Zhihu and Reddit, there’s a growing belief that Amazon interviews are getting easier. Many assume that brushing up on LeetCode is enough to secure an offer. But once you’re in the actual interview, faced with live questioning, complex follow-ups, and time pressure, it becomes clear this isn’t just about algorithms. Amazon wants problem solvers with clear thinking, strong communication, and the ability to stay logical under pressure.
This is a real case from a CSOAHELP-supported Amazon interview. The candidate was faced with what seemed like a standard combination optimization problem. With guided real-time assistance, they broke the problem down under pressure, responded strategically to follow-ups, and successfully passed the interview. This was both a technical challenge and a psychological test—and our service made a real impact.
Amazon frequently offers product bundles to customers, where multiple related products are combined and sold together at a discounted price. The goal is to create attractive bundle offers that maximize potential revenue.
Given two equally sized arrays, A and B, where A represents the individual selling prices of a set of products, and B represents the individual selling prices of another set of related products. The size of these arrays, N, represents the number of products in each set.
Amazon wants to identify the top K most lucrative product bundle combinations by combining one product from set A and one product from set B. The revenue generated from a bundle is calculated as the sum of the individual product prices in the bundle.
Example:
A = [1, 2, 3, 4]
B = [2, 7, 1, 2]
When the interview began, the interviewer introduced the problem. Our candidate, coached by CSOAHELP beforehand, immediately asked clarifying questions like: “Are we measuring revenue strictly by summing prices?”, “Can we assume K ≤ N²?”, and “Should the output be tuples like (revenue, index in A, index in B)?” These questions helped prevent misinterpretations and showed good reasoning.
The candidate first offered a brute-force solution: iterate over all pairs, calculate revenue, sort them in descending order, and return the top K combinations. The interviewer immediately pushed back, asking: “Would this scale to large datasets? Is this practical in a production scenario?”
We pushed a real-time text hint: brute-force has a time complexity of N² log N² and space complexity of N²—okay for small datasets or testing but not production-scale. The candidate followed up smoothly, acknowledging these limitations and naturally pivoted to an optimized heap-based approach.
They explained that by using a min-heap, they could track the top K bundles as they iterate over all combinations. If the heap isn’t full, insert the new bundle. If it is and the new bundle’s revenue is higher than the smallest in the heap, replace it. This reduces space usage dramatically, only maintaining K entries.
The interviewer didn’t stop there. They asked, “If A and B each have tens of thousands of items, does your solution still hold? Can you avoid iterating over every combination?”
The candidate hesitated—that was outside their initial preparation. We immediately pushed a new prompt via the side screen: sort both arrays, use a greedy + heap strategy, generate only two new candidate pairs per step (by incrementing indices in A or B), and use a visited set to avoid duplicates. This reduces time complexity closer to K log K.
With this direction, the candidate smoothly explained the improved strategy to the interviewer, detailing how sorted arrays and controlled index movement limit unnecessary work, while the heap functions like a priority queue to manage revenue order. The interviewer started nodding in approval.
When it came to coding, we again provided step-by-step structural hints. The brute-force + heap version looked like this:
min_heap = []
for i, a in enumerate(A):
for j, b in enumerate(B):
revenue = a + b
if len(min_heap) < k:
heapq.heappush(min_heap, (revenue, i, j))
elif revenue > min_heap[0][0]:
heapq.heappushpop(min_heap, (revenue, i, j))
The candidate recited and explained the logic clearly, walking through how the heap works and why heappushpop is used to maintain the top K. The interviewer then asked, “How would you make this code more robust for even larger inputs?”
We instantly provided another suggestion: use a greedy two-pointer approach to generate only the next best pair, insert into heap, and repeat—avoiding the need to precompute all combinations. The candidate followed our phrasing, gave an example, and explained how this strategy reduces memory and boosts performance. The interviewer didn’t push back—in fact, they began taking notes.
The interview lasted about 45 minutes. The candidate started off nervous, but with our assistance pacing the session, they found their rhythm. We pushed structure, logic, and phrasing guidance at every key point—from clarifying the problem to proposing optimizations and writing code. The candidate simply repeated or paraphrased the hints naturally, without awkward pauses or missteps.
After the interview, the first thing the candidate said was: “I’m so glad I had you guys. I wouldn’t have thought to optimize the heap part, and that whole greedy step-by-step logic saved me.” We didn’t answer for them, but we gave timely help so they could showcase their best.
This case proves once again: algorithms are only part of it. Clear thinking, engineering trade-offs, and structured reasoning under pressure are what top companies really care about.
That’s exactly where CSOAHELP’s real-time remote assistance comes in.
We not only help you prepare problem-solving templates in advance—we actively support you during real interviews, using a second device to monitor and silently feed prompts, helping you give clear, complete, logical answers every time. We’re not replacing your effort; we’re keeping your momentum, focus, and clarity on track.
Whether you're interviewing at Amazon, Google, Meta, Apple, or Stripe, CSOAHELP can customize support just for you.
Big tech interviews today are more like battles of reasoning and communication than pure coding drills. Are you ready to step up?
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
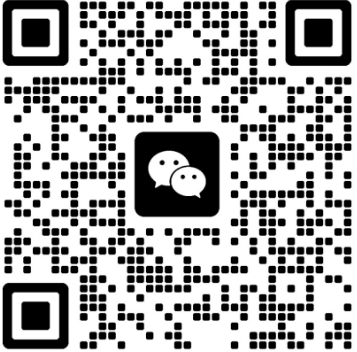