"I’ve done hundreds of LeetCode problems. Why do I still choke in big tech interviews?"
Because what you think is an algorithm test is actually about: real-time problem solving, structured thinking, and communication under pressure.
One candidate who advanced to the next round of a Bloomberg interview proves this perfectly.
In a real Bloomberg interview, a candidate faced a deceptively simple yet layered problem. Luckily, he wasn’t alone.
CSOAHELP provided real-time remote support during the entire interview. While the candidate spoke with the interviewer via the main screen, our team silently monitored the session from a secondary device. Before each response, we pushed clear, complete written guidance, logical breakdowns, and if needed, code drafts. All the candidate had to do was naturally paraphrase or type the code.
Here’s the original interview prompt:
You're creating a website where people can track the bus and figure out when to go to the station.
The buses move between the stations in one direction.
The goal is to find the station any bus is currently at or find how many stops away the nearest bus is to a requested station.
Buses are never between stations, they are only stopped at a station.
Your task is to implement this class:
class BusTracker {
constructor(stationList, busLocations) {}
public nearestBusToStation(stationId: string): number {
return -1;
}
public getBusLocation(busId: number): string {
return "";
}
};
At first glance, this looked like a basic data structure question. But it was actually testing system modeling, interface design, and live communication skills. The candidate’s initial reaction was, “Isn’t this just a Map lookup and index difference check?”
Just before he said that aloud, our system pushed this written guidance to his secondary screen:
“Break stationList into an array called stationArray. Map each station to its index. Use busLocations to build a busId-to-station map, and also a station-to-buses map to track which buses are currently at each station. The nearestBusToStation method should search backward from the target station until it finds one with a bus, then return the offset. The getBusLocation method just returns from the map.”
The candidate skimmed the message and smoothly replied: “I’ll split the station string into an array and create a stationIndexMap to store the index of each station. Then I’ll search backwards from the target station to see if a bus is stopped at any earlier station. If so, I’ll return the distance.”
The interviewer approved and asked him to write the code.
We then pushed a clean TypeScript draft. The candidate typed smoothly with no pauses:
class BusTracker {
stationArray: string[];
stationIndexMap: Map<string, number>;
stationToBuses: Map<string, number[]>;
busToStation: Map<number, string>;
constructor(stationList: string, busLocations: Record<number, string>) {
this.stationArray = stationList.split("-");
this.stationIndexMap = new Map();
this.stationToBuses = new Map();
this.busToStation = new Map();
for (let i = 0; i < this.stationArray.length; i++) {
this.stationIndexMap.set(this.stationArray[i], i);
this.stationToBuses.set(this.stationArray[i], []);
}
for (let busId in busLocations) {
const station = busLocations[+busId];
this.busToStation.set(+busId, station);
this.stationToBuses.get(station)?.push(+busId);
}
}
nearestBusToStation(stationId: string): number {
const index = this.stationIndexMap.get(stationId);
if (index === undefined) return -1;
for (let i = 0; i <= index; i++) {
const station = this.stationArray[i];
const buses = this.stationToBuses.get(station);
if (buses && buses.length > 0) {
return index - i;
}
}
return -1;
}
getBusLocation(busId: number): string {
return this.busToStation.get(busId) ?? "";
}
}
The implementation was clean and logical, with proper variable names. The interviewer confirmed the correctness—but the challenge didn’t end there.
Next came a follow-up: “What if each bus moves forward automatically every second? How would you redesign this class?”
The candidate hesitated. We instantly pushed this support message:
“Each bus can store a startTime and initial station. Assume it moves one station per second. Calculate the elapsed time and derive its current position: start index + time difference ÷ interval. Recommend moving this logic outside of BusTracker to a dedicated module.”
The candidate regrouped and responded: “Each bus can store its starting station and timestamp. Then based on the current time, I can calculate how many stations it’s moved and update its position. To keep things clean, a helper module can handle this logic so BusTracker stays focused.”
The interviewer smiled and pushed further: “What if a bus moves beyond the last station?”
Again, we supported with a key reminder: “If the computed index exceeds the array length, return undefined or the final station. Add boundary protection in the design.”
The candidate responded confidently and added a brief mention of buffer handling and fail-safes.
This session showed a complete arc: correct implementation, clear structure, and confident handling of multiple follow-ups. While the candidate was still developing his modeling and explanation skills, CSOAHELP filled every gap, allowing him to maintain momentum and move to the next round.
The real challenge wasn’t the code—it was being able to break down the problem live, propose a clean structure, explain it fluently, and adapt to pressure.
And that’s exactly what CSOAHELP gives you. We don’t just help you solve the problem. We help you say it, code it, and stay composed.
You practice LeetCode to get ready. But CSOAHELP is your second brain in the actual fight.
Live support, real-time strategy, question prediction, response structuring. If you can read and speak, we can guide you to pass.
This Bloomberg interview is just one example.
We hope the next one is yours.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
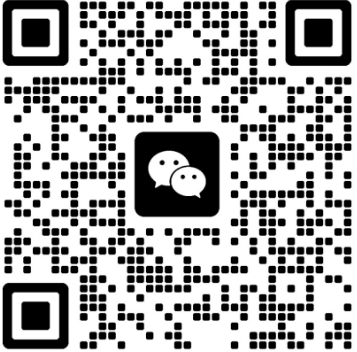