Question 6
Implement a Python decorator called timer
that takes a single argument, which is the function to be timed. The decorator should push the execution time (in milliseconds) of the function after it completes to a list called execution_time
which is already declared in the global scope. The function should return the same value it would return if it were not timed.
Example
function_name = "delay_max", args = [3,4,1], time_delay = 150
Decorate the function delay_max
with timer
, and call the decorated function with arguments 3, 4 and 1 with delay = 150.
decorated_func = timer(delay_max)
print(decorated_func(3, 4, 1, delay=150))
The above code should output 4
, which is the return value of the function call delay_max(3, 4, 1, delay=150)
. The function delay_max
takes 150 ms to complete, so, the decorator should push a value close to 150 to the list execution_time
.
Function Description
Complete the function timer
in the editor below. The function is a decorator with the below properties.
timer
has the following parameter:
func
: A function
Constraints
- 1 ≤ n ≤ 20
- 1 ≤ args[i] ≤ 100
function_name()
∈ {“delay_max”, “delay_min”, “delay_sum”}- 50 ≤
time_delay
≤ 400 time_delay
is a multiple of 50
Input Format for Custom Testing
Sample Case 0
Sample Input for Custom Testing
delay_max 3 2 1 100
delay_sum 2 3 5 200
delay_min 4 5 1 300
Sample Output
3
10
1
[100, 200, 300]
Explanation
Here, there are 3 function calls
function_name = "delay_max", args = [3, 2, 1], time_delay = 100
decorate the function delay_max
with timer
, and call the decorated function with arguments 3, 2 and 1 with delay = 100. It should return 3
. The decorator should push a value close to 100 to the list execution_time
.
function_name = "delay_sum", args = [2, 3, 5], time_delay = 200
decorate the function delay_sum
with timer
, and call the decorated function with arguments 2, 3 and 5 with delay = 200. It should return 10
. The decorator should push a value close to 200 to the list execution_time
.
function_name = "delay_min", args = [4, 5, 1], time_delay = 300
decorate the function delay_min
with timer
, and call the decorated function with arguments 4, 5 and 1 with delay = 300. It should return 1
. The decorator should push a value close to 300 to the list execution_time
.
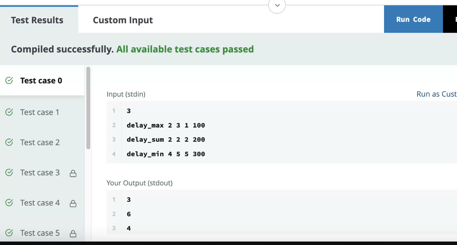
Question 7
Use the HTTP GET method to retrieve information from a database of weather records. Query:
https://jsonmock.hackerrank.com/api/weather/search?name={keyword}
to search all the records where name has a keyword anywhere in its string value. The query result is paginated and can be further accessed by appending to the query string &page=num
where num
is the page number.
The query response from the API is a JSON with the following five fields:
Response Fields
page
: the current pageper_page
: the maximum number of results per pagetotal
: the total number of records in the search resulttotal_pages
: the total number of pages to query to get all the resultsdata
: an array of JSON objects that contain weather records
The data
field in the response contains a list of weather records with the following schema:
Weather Record Fields
name
: the name of the cityweather
: temperature recordedstatus
: an array of wind speed and humidity records
Filter records to include a given keyword in the name parameter. Return an array such that each element is a string of comma-separated values: city_name, temperature, wind, humidity
.
For example:
{
"name": "Adelaide",
"weather": "15 degree",
"status": {
"wind": "6kmph",
"humidity": "61%"
}
}
The JSON record is stored as:
Adelaide,15 degree,6kmph,61%
Return the list sorted by city name.
Function Description
Complete the function weatherStation(keyword)
in the editor below.
weatherStation
has the following parameters:
string keyword
: the string to search
Returns
string[]
: the weather data for each city
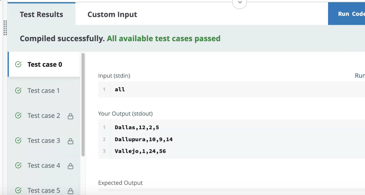
Question 8
Implement the SumTable
data structure in Python. Its purpose is to manage tabular data of integers according to the following requirements:
- It is constructed as
table = SumTable(arr)
wherearr
is a list ofn
integer lists, each of lengthm
. This constructs aSumTable
withn
rows andm
columns wherecell(i, j)
initially contains the value ofarr[i][j]
. - It supports the
get
operator such that the expressiontable[i][j][2]
returns the sum of values of all cells(i', j')
such that0 ≤ i' ≤ i
and0 ≤ j' ≤ j
. - It supports the assignment operator such that the statement
table[i][j] = k
assigns the valuek
to the cell(i, j)
.
Implement any additional methods as necessary.
The implementation will be tested by a code stub using several input files. Each input file contains the data to initialize the table followed by the description of the operations to be performed. First, a table instance of the SumTable
class is constructed using the initialization data. Then, the operations are performed. There are two types of operations: get
and set
.
get i j
callstable[i][j][2]
and appends the value of that expression to the results list.set i j k
executes the assignmenttable[i][j] = k
.
Values returned by the get
operation are appended to the results list which is printed by the provided code stub.
Constraints
- 1 ≤ n, m ≤ 500
- 1 ≤ number of operations in one test file ≤ 10^5
- -100 ≤ arr[i][j], k ≤ 100
- There are at most 50 assignment operations in one test file
- 0 ≤ i < n
- 0 ≤ j < m
Sample Case 0
Sample Input
2
3
5
STOIN
2 rows
3 columns
arr = 2 x 3
arr = [[1, 2, 3], [4, 5, 6]]
5 number of queries q = 5
get 1 2
set 0 0 10
get 1 2
set 0 1 0
get 1 2
Sample Output
16
24
24
Explanation
The table has 2 rows and 3 columns and its values are initialized according to the given input array. There are 5 operations to be performed.
The first operation asks what is the sum of cells (i, j) in the table for 0 ≤ i ≤ 1 and 0 ≤ j ≤ 2
. There are 6 such cells: (0,0), (0,1), (0,2), (1,0), (1,1), (1,2). The sum of their values is 1 + 2 + 3 + 4 + 5 + 6 = 21.
The second operation sets the value of cell (0,0) to 10. The third operation again asks for the sum of the same cells as the first operation asked. This sum is now 10 + 2 + 3 + 4 + 5 + 6 = 30.
The fourth operation sets the value of cell (0,1) to 0. The fifth operation asks what is the sum of cells (i, j) in the table for 0 ≤ i ≤ 1 and 0 ≤ j ≤ 2
. There are 6 such cells: (0,0), (0,1), (0,2), (1,0), (1,1), (1,2). The sum of their values is 10 + 0 + 3 + 4 + 5 + 6 = 28.
The values returned by all the get
operations are printed to the standard output.
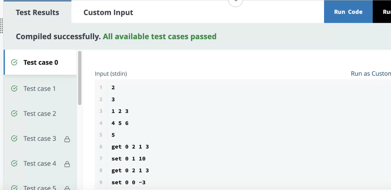
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
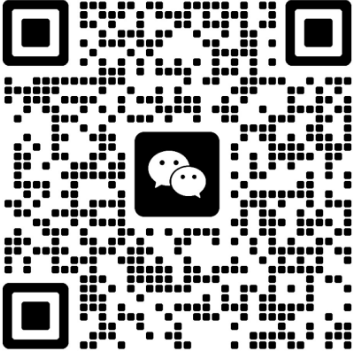