When preparing for a Google interview, many believe that as long as they've solved enough LeetCode problems and mastered algorithm patterns, they're ready. But the real challenge often isn’t just about writing functional code. You need to grasp the problem quickly, deconstruct it, implement a working solution, explain your logic clearly—and be prepared for rapid-fire follow-up questions from the interviewer. Under pressure, the easiest-looking questions can become traps.
This is a real interview case supported by CSOAHELP through full remote assistance. The candidate had three years of development experience and was applying for a Google backend SDE position. While technically solid, he lacked confidence in communication and real-time problem solving, so he chose our live support service. Here's exactly what happened in that Google video interview.
The interviewer presented the problem:
'(${operator} ${expression_1} ${expression_2} ${expression_3}....)'
Examples:
(+ 3 4 5) (+ 3 (+ 1 3) 5) (+ 3 4 (+ 2 3))
The task was to parse and evaluate this expression, supporting only addition and multiplication, with potential nesting.
At the start, the candidate attempted to reason through the structure and considered building an abstract syntax tree, but nerves took over and his thoughts got tangled. He froze. At that moment, we immediately provided text-based assistance on his secondary screen: use recursion to parse the expression. Every time you see a '(', treat it as a sub-expression. The first token is the operator, followed by numbers or nested expressions. Tokenize first, then recursively evaluate with a parse function.
This helped him quickly refocus, and he began implementing the tokenizing and recursive parsing logic. While typing, he echoed our prompt to the interviewer: “I plan to use recursion here. Every time I encounter a '(', I’ll extract the operator first, then recursively evaluate each operand.”
The interviewer pressed further: “What happens if there’s a deeply nested input with millions of layers? Wouldn’t that cause a stack overflow? How would you optimize it?”
We swiftly provided guidance: the current recursive approach is acceptable for mid-sized expressions in a controlled interview setting. In production, it could be converted to an iterative parser using manual stack management. Tail recursion or stack depth limits could also be introduced to prevent overflow. The candidate repeated our explanation confidently, successfully convincing the interviewer that his approach was reasonable for the context.
Later, the interviewer asked him to add support for multiplication. Once again, we delivered a full structural hint: just extend the apply_operator function to include logic for multiplication alongside addition. The candidate paraphrased this and implemented the following:
def evaluate_expression(expression):
tokens = [token.strip() for token in expression.split() if token]
def parse_expression(tokens, index):
index += 1
operator = tokens[index]
index += 1
operands = []
while index < len(tokens) and tokens[index] != ')':
if tokens[index] == '(':
value, index = parse_expression(tokens, index)
operands.append(value)
else:
operands.append(float(tokens[index]))
index += 1
index += 1
return apply_operator(operator, operands), index
def apply_operator(operator, operands):
if operator == '+':
return sum(operands)
elif operator == '*':
result = 1
for op in operands:
result *= op
return result
result, _ = parse_expression(tokens, 0)
return result
As he recited the code, we simultaneously provided a logical breakdown so he could explain each part clearly. The interviewer continued with probing questions like "How would you support other operators?" "What happens when nesting becomes extremely deep in practice?" and "Can you do this in a single pass?" Each of these was met with a prepared answer thanks to our support system.
The interviewer was ultimately satisfied with the candidate’s clarity and structure, and invited him to the next round—system design.
This candidate was not particularly outstanding technically. In fact, he froze under pressure multiple times. But with CSOAHELP’s real-time text-based guidance, he was able to regain focus at each critical moment, articulate his approach, and present clean, understandable solutions. Even without strong low-level coding fluency, simply following and repeating our structured prompts allowed him to appear logical and well-prepared.
We’ve seen this happen repeatedly. Many candidates panic in live interviews. It’s not that they don’t know the answer—it’s the sudden interruptions, questions, or pressure that throw them off. That’s where CSOAHELP’s value becomes undeniable.
All you need is your headset and camera. We’ll be on your second device offering step-by-step suggestions. That includes: full problem breakdowns, logical planning help, code structure templates, and sentence framing assistance. You don’t need to improvise from scratch—just follow the guide we prepare for you.
As interviews at Google, Meta, Apple, Stripe and others get faster and more engineering-focused, even the most well-prepared candidate can falter under stress. You don’t have to face it alone. CSOAHELP is your hidden tactical support team.
If you’re about to face a crucial interview round, stop relying on luck or hope. Use the right method, leverage a proven support system, and maximize your chances of success.
DM us for more details. We won’t promise 100% success—but we’ll make sure you show your 100% potential.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
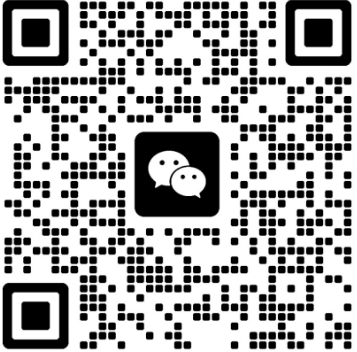