“刷了几百道 LeetCode,为什么我还是在大厂面试中频频卡壳?”
因为你以为自己在考算法,其实对方在考:临场反应、表达逻辑、系统能力。
这位拿下 Bloomberg 面试下一轮的候选人,就是一个典型案例。
在 Bloomberg 的一场真实面试中,一位求职者遇到了一道乍看不难、实则充满陷阱的题目。幸运的是,他不是一个人在战斗。
这场面试中,CSOAHELP 提供了远程实时辅助服务。候选人通过主设备与面试官正常对话,我们在他的副设备上同步观察整个面试过程,并在他每次回答前,推送清晰、完整的文字分析、逻辑思路和代码草稿。候选人只需自然地复述或抄写,即可顺利应答。
面试题原文如下:
You're creating a website where people can track the bus and figure out when to go to the station.
The buses move between the stations in one direction.
The goal is to find the station any bus is currently at or find how many stops away the nearest bus is to a requested station.
Buses are never between stations, they are only stopped at a station.
Your task is to implement this class:
class BusTracker {
constructor(stationList, busLocations) {}
public nearestBusToStation(stationId: string): number {
return -1;
}
public getBusLocation(busId: number): string {
return "";
}
};
这是一道典型的“变形题”:结构简洁,看似是考基本的数据结构,其实意在考察建模思维、接口设计能力以及临场表达逻辑。候选人第一反应是:“这不就是一个 Map 查询和索引差值的判断吗?”
正当他准备直接说出这个想法时,我们的提示系统立刻在副设备上推送如下文字建议:
“建议将 stationList 拆分为数组 stationArray,并为每个站点建立 index 映射;busLocations 可构建为 busId 到站点的映射,同时记录每个站点当前停靠的所有 busId;nearestBusToStation 方法的逻辑可转化为:从目标站点往前查找,直到找到有公交车的站点,返回差值;getBusLocation 方法可直接返回映射结果。”
候选人在看到提示后,快速整理语言,对面试官自然地回答道:“我会把站点字符串按顺序拆分为数组,并建立 stationIndexMap 记录每个站的索引,然后反向查找当前站点之前是否有公交车停靠,如果找到了,计算出距离即可。”
面试官表示认可,并让他现场写代码。
此时我们提供了精炼的 TypeScript 草稿,候选人按照提示输入代码,全程顺利无卡顿:
class BusTracker {
stationArray: string[];
stationIndexMap: Map<string, number>;
stationToBuses: Map<string, number[]>;
busToStation: Map<number, string>;
constructor(stationList: string, busLocations: Record<number, string>) {
this.stationArray = stationList.split("-");
this.stationIndexMap = new Map();
this.stationToBuses = new Map();
this.busToStation = new Map();
for (let i = 0; i < this.stationArray.length; i++) {
this.stationIndexMap.set(this.stationArray[i], i);
this.stationToBuses.set(this.stationArray[i], []);
}
for (let busId in busLocations) {
const station = busLocations[+busId];
this.busToStation.set(+busId, station);
this.stationToBuses.get(station)?.push(+busId);
}
}
nearestBusToStation(stationId: string): number {
const index = this.stationIndexMap.get(stationId);
if (index === undefined) return -1;
for (let i = 0; i <= index; i++) {
const station = this.stationArray[i];
const buses = this.stationToBuses.get(station);
if (buses && buses.length > 0) {
return index - i;
}
}
return -1;
}
getBusLocation(busId: number): string {
return this.busToStation.get(busId) ?? "";
}
}
这份代码逻辑清晰,变量命名合理,面试官很快确认了实现正确。但并没有就此结束。
紧接着面试官发问:“如果公交车会自动每秒移动一站,该怎么扩展这个类的设计?”
这个问题并不容易。候选人略显迟疑,我们立刻推送如下提示:
“可以为每辆车增加起始时间字段 startTime 和初始位置 currentStation,设定每秒移动一站。根据当前时间计算出经过的秒数,即可推算当前站点为 起始站点索引 + 时间差除以 interval(如1秒一站)。建议将该逻辑从 BusTracker 主类中解耦出来,由单独模块处理。”
候选人看到提示后,迅速调整状态,说道:“我们可以让每辆 bus 保存一个初始站点和启动时间,再用当前时间计算出它已经前进了几站,通过索引加减得出当前站点。这个功能可以由一个辅助模块专门计算当前位置,而不是耦合在主类中。”
这段回答让面试官露出满意的神情,并跟进追问:“那如果某辆车行驶超过终点站,会如何处理?”
我们再次推送简明要点:“当计算出的站点索引超出 stationArray 长度,应返回 undefined 或最后一站作为默认值,同时在设计中预留越界保护逻辑。”
候选人顺利回应,并强调系统可加一层缓冲或容错策略来处理这种场景。
这场面试中,候选人完成了完整实现、结构化思维展示、多轮逻辑追问应对。其实他自身在表达和建模能力上还比较初级,但得益于 CSOAHELP 的全程辅助,他成功把控了节奏,并顺利进入下一轮面试。
这道题真正难的从来不是代码,而是如何在现场:拆解问题,提出结构清晰的方案,保持表达流畅,还能及时应对面试官的追问。
而这正是 CSOAHELP 能给你的:我们不仅帮你看懂题,还能帮你“说清楚、写出来、稳住场”。
你刷题,是为了准备面试。但在真正的面试中,CSOAHELP 是你背后的第二大脑、是你战术上的强力支援。
远程辅助,模拟实战,预测追问,生成应答逻辑。只要你能听懂并复述,我们就能帮你顺利通过。
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
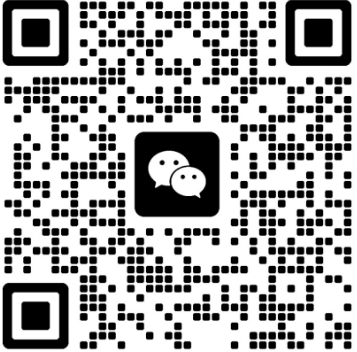