1. String Patterns
Given the length of a word (wordLen
) and the maximum number of consecutive vowels that can be used (maxVowels
), determine how many unique words can be generated.
Words will consist of English alphabetic letters a through z only.
Vowels are {a, e, i, o, u}; consonants are the remaining 21 letters.
In the explanations, v and c represent vowels and consonants.
wordLen = 1
maxVowels = 1
Patterns: {v, c}
That means there are 26 possibilities, one for each letter in the alphabet.
V or C
5 21
wordLen = 4
maxVowels = 1
Patterns: {cccc, vccc, cvcc, ccvc, cccv, vcvc, cvcv, cvvc, vccv}
There are 412,776 possibilities – see below:
C C C C → 21 * 21 * 21 * 21 = 194481
V C C C → 5 * 21 * 21 * 21 = 46305
C V C C → 21 * 5 * 21 * 21 = 46305
C C V C → 21 * 21 * 5 * 21 = 46305
C C C V → 21 * 21 * 21 * 5 = 46305
→ 4 * 46305 = 185220
V C V C → (5 * 21 * 5 * 21) = 11025
C V C V → (21 * 5 * 21 * 5) = 11025
C V V C → (21 * 5 * 5 * 21) = 11025
V C C V → (5 * 21 * 21 * 5) = 11025
→ 4 * 11025 = 33075
Total: 194481 + 185220 + 33075 = 412776
wordLen = 4
maxVowels = 2
In this case, all of the combinations from the previous example are still valid.
- There are 5 additional patterns to consider, three with 2 vowels (
vvcc
,cvvc
,ccvv
) and two with 3 vowels (vvvc
andvccv
) - Their counts are:
3 * (5 * 5 * 21 * 21) = 3 * 11025 = 33075
2 * (5 * 5 * 5 * 21) = 2 * 2625 = 5250
- The total number of combinations then is:
412776 + 33075 + 5250 = 451101
The result may be a very large number, so return the answer modulo (10^9 + 7).
Note
While the answers will be within the limit of a 32-bit integer, interim values may exceed that limit. Within the function, you may need to use a 64-bit integer type to store them.
2. Work Schedule
An employee has to work exactly as many hours as they are told to each week, scheduling no more than a given daily maximum number of hours. On some days, the number of hours worked will be given. The employee gets to choose the remainder of their schedule, within the given limits.
A completed schedule consists of exactly 7 digits in the range 0 to 8 that represent a day's work hours. A pattern string similar to the schedule is given, but some of its digits are replaced by a question mark, ?
(ascii 63 decimal). Given a maximum number of hours that can be worked in a day, replace the question marks with digits so that the sum of scheduled hours is exactly the hours that must be worked in a week.
Determine all possible work schedules that meet the requirements and return them as a list of strings, sorted ascending.
Example
pattern = '08??840'
work_hours = 24
day_hours = 4
There are two days on which they must work24 - 20 = 4
more hours for the week.
All of the possible schedules are listed below:
0804840
0813840
0822840
0831840
0840840
Function Description
Complete the function findSchedules
in the editor below.
findSchedules
has the following parameter(s):
int work_hours
: the hours that must be worked in the weekint day_hours
: the maximum hours that may be worked in a dayint pattern
: the partially completed schedule
Returns
string arr[]
: represents all possible valid schedules (must be ordered ascending)
Constraints
1 <= work_hours <= 56
1 <= day_hours <= 8
|pattern| = 7
- Each character of
pattern
∈ {0, ..., 8} - There is at least one correct schedule.
Sample Input
STDIN Function
----- --------
3 → work_hours = 3
2 → day_hours = 2
??? ??0 0 → pattern = '?????00'
Sample Output
0020100
0021000
0120000
1020000
Explanation
work_hours = 3
day_hours = 2
pattern = '?????00'
They only need to schedule 1 more hour for the week, and it can be on any one of the days in question.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
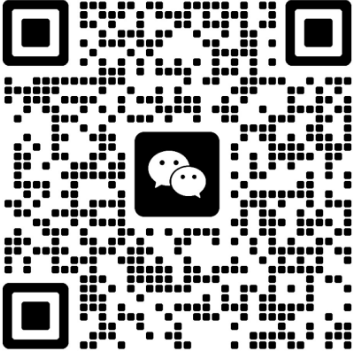