Problem:
You are given an array forest
and the bird’s starting position bird
.
forest[i]
represents the length of the stick at positioni
.- If
forest[i] == 0
, it means there is no stick at positioni
. - The bird’s starting position
bird
will always be an index where there is no stick (forest[bird] == 0
).
The bird follows this process:
- Starting from the initial position, the bird flies right until it finds the first stick.
- It picks up the stick and brings it back to the starting position.
- Next, the bird flies left until it finds the next stick.
- It picks up that stick and brings it back to the starting position.
- The bird repeats this process: alternating right and left until it collects sticks with a total length of at least 100.
You need to output the indices of the sticks that the bird picks up, in the order they are collected.
✅ Note:
- There will always be a stick available in the current flying direction.
- No edge cases like "flying right and finding no sticks" will occur.
Example:
forest = [0, 50, 0, 30, 0, 25]
bird = 2
The bird’s collection order would be:
[3, 1, 5]
✅ Explanation:
- First, the bird flies right to index 3 (stick of length 30).
- Then left to index 1 (stick of length 50).
- Then right again to index 5 (stick of length 25).
- Total length = 30 + 50 + 25 = 105 (which is >= 100), so the process stops.
Bubble Popping Game:
You are given a 2D array grid
, where grid[i][j]
represents the color of the bubble at position (i, j)
. You are also given an operations
array, which is a sequence of coordinates indicating where to pop bubbles.
Rules:
- If you pop a bubble at a position with value
0
(meaning no bubble), nothing happens. - If you pop a bubble, check its top-left, bottom-left, top-right, and bottom-right diagonal neighbors:
- If the neighbor is within bounds, has a bubble (non-zero), and the color is the same as the popped bubble, that neighbor also pops.
- After popping, gravity applies: bubbles above empty cells fall down to fill the empty spaces directly below them.
- Perform all operations in order and return the final grid.
Example:
grid = [
[1, 2, 3],
[6, 1, 2],
[1, 2, 5]
]
operations = [(1, 1), (1, 2)] # Note: 0-based indexing assumed
Step 1: Pop bubble at (1, 1)
- Pops the bubble itself
- Pops diagonally connected bubble
(0, 0)
because it has the same color1
Apply gravity:
[
[0, 0, 3],
[0, 2, 2],
[6, 2, 5]
]
Step 2: Pop bubble at (1, 2)
- Pops the bubble itself
- Pops diagonal
(0, 1)
because it’s2
- Pops diagonal
(2, 1)
because it’s also2
Apply gravity:
[
[0, 0, 0],
[0, 0, 3],
[6, 2, 5]
]
Final Output:
[
[0, 0, 0],
[0, 0, 3],
[6, 2, 5]
]
Balloon Color Pairs Problem:
You are given an integer length
representing the length of a balloon queue (indexed from 0
to length - 1
) and an array queries
.
Initially, the balloon queue is empty (all positions are uncolored or considered empty).
Each query[i] = (index, color)
means you color the balloon at position index
with the given color
.
After processing each query, you need to count the number of adjacent pairs of balloons that have the same color and record that number.
At the end, you should return an array containing the count after each query — the length of the result array is the same as the number of queries.
Example:
length = 5
queries = [(0, 1), (1, 1), (2, 1), (1, 3)]
Initial state:
_ _ _ _ _ (empty)
Process each query:
(0, 1)
→1 _ _ _ _
→ 0 adjacent same-color pairs(1, 1)
→1 1 _ _ _
→ 1 pair (positions 0 and 1)(2, 1)
→1 1 1 _ _
→ 2 pairs (0–1 and 1–2)(1, 3)
→1 3 1 _ _
→ 0 pairs
Final Output:
[0, 1, 2, 0]
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
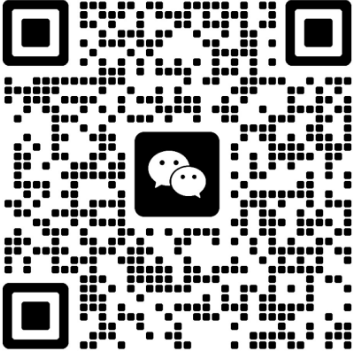