This is the story of a candidate applying for a systems engineering position at Meta. From the first contact to receiving the offer, the entire process took just three weeks. What made the difference wasn’t endless LeetCode practice, but the real-time remote support provided by CSOAHELP during the technical interview.
Many assume that "interview assistance" sounds shady or unreliable, but this story may change your mind. We’ll walk you through the entire Meta technical interview and show how the candidate received discreet, real-time guidance—text prompts, algorithm breakdowns, expression suggestions, even full code snippets for reproduction—all without the interviewer noticing a thing.
Before the interview, the candidate admitted he had decent technical foundations but struggled under pressure, especially when faced with layered follow-ups. After assessing his background, we scheduled a mock interview to pinpoint logic gaps, expression habits, and common failure points. On the day of the real interview, we set up a dual-device system: his main device connected to Zoom with the interviewer, while a secondary device used ToDesk to link with our prompt terminal, where we pushed structured and timely suggestions onto the screen’s side panel.
The interview officially began. The interviewer greeted him briefly and immediately shared the first problem:
# Given a list of integers and a window size, return a list of the moving averages.
#
# Example:
# Input: [1,2,3,4,5,6,7,8,9], window size: 7
# Output: [4.0, 5.0, 6.0]
We immediately sent over a prompt to clarify the requirement:
"Hi, I just want to confirm: for an array of length n and a window size k, we are expected to compute the average of each valid window of size k, resulting in (n-k+1) averages. Is that correct?"
The candidate repeated this confidently and received positive feedback. Then we pushed the step-by-step breakdown:
Approach: Use a sliding window + cumulative sum variable. Initialize the sum with the first k elements. Append the first average to the result list. Slide the window: add the new number, remove the old one. Calculate and append each new average.
We also provided a complete code snippet for seamless reproduction:
def moving_average(arr, k):
if len(arr) < k:
return []
res = []
window_sum = sum(arr[:k])
res.append(window_sum / k)
for i in range(k, len(arr)):
window_sum += arr[i] - arr[i-k]
res.append(window_sum / k)
return res
The interviewer wasn’t done yet. He followed up: "What’s the space complexity of your solution? Can it be optimized further?"
We quickly delivered the response:
"Apart from the output array, this approach only uses a constant variable to maintain the sum, so the space complexity is O(1). The time complexity is O(n). Unless we discard the result list, it’s already optimal."
The candidate repeated this smoothly and earned the interviewer’s approval. The next challenge followed: "What if the data is a real-time stream? Would your solution still work?"
We prompted:
"You could use a deque to maintain a fixed-size sliding window or design a class that handles real-time moving average calculation using cumulative sum and count."
The candidate constructed a rough structure and explained the stream-based approach. Though slightly nervous, he stayed on track and cleared the round.
The interviewer then moved on to the second question:
# Given the root of a binary tree of integers, print the columns of the
# tree in order with the nodes in each columns printed top-to-bottom.
He also showed a sketch of the tree:
6
/ \
3 4
/ \
5 1
/
2
/ \
9 7
Expected output: 5 9 3 2 6 1 7 4
Immediately, we pushed a structural breakdown: this is essentially a vertical order traversal. We recommended using BFS to preserve top-to-bottom ordering within columns.
Key ideas: Use a queue for BFS traversal, tagging each node with a column index (root=0, left=col-1, right=col+1). Use defaultdict(list) to store column → node mappings. Sort column keys and output values accordingly.
We provided the Python scaffold for direct reproduction:
from collections import defaultdict, deque
def vertical_order(root):
if not root:
return []
col_table = defaultdict(list)
queue = deque([(root, 0)])
while queue:
node, col = queue.popleft()
col_table[col].append(node.val)
if node.left:
queue.append((node.left, col - 1))
if node.right:
queue.append((node.right, col + 1))
result = []
for col in sorted(col_table.keys()):
result.extend(col_table[col])
return result
The candidate explained while coding, and we prepped him for follow-up questions.
When asked, "Why not use DFS instead?" we prompted:
"BFS naturally preserves top-to-bottom order. DFS could return deeper nodes before higher ones in the same column."
Asked about time complexity, the candidate answered as we suggested:
"Time complexity is O(n) since each node is visited once. Space complexity is also O(n) due to the queue and hashmap."
Final follow-up: "How would you extend this to support node attributes like coordinates, color, etc.?"
We prompted:
"You could extend the node structure and maintain additional metadata during BFS—for example, using a priority queue to order by color or adding level markers for same-column sorting."
The candidate handled it well. The interviewer ended with, "Your answers were very complete."
In the debrief afterward, the candidate said:
"I thought I was ready, but the moment the interviewer followed up, I blanked. You gave me full logic and phrasing before I even processed the question. It really felt like a cheat code."
So what exactly is real-time remote interview assistance? CSOAHELP doesn’t take the interview for you or lie about your experience. What we do is simple yet powerful: when your mind goes blank or your explanation falters, we step in with fast, clear guidance, complete structures, even clean code—so you can repeat or paraphrase naturally, as if you had prepped it all yourself.
You’re still being authentic, but you're showing your best self under pressure.
Technical skills get your foot in the door. Clear thinking and poise are what get you through. Meta, Google, Stripe, Apple—they increasingly value systems thinking, communication, and logic—not just whether your code compiles. If you’ve been grinding LeetCode for three months and still stumble under pressure, CSOAHELP might just be your Plan A.
We’ve seen too many strong candidates fail due to nerves or disorganized thinking. Our goal is to help you present the best version of your real ability—stay calm, deliver every answer smoothly, and earn the offer you deserve.
Curious about how it works? Message us anytime. We’ll tailor a strategy just for your role and target company.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
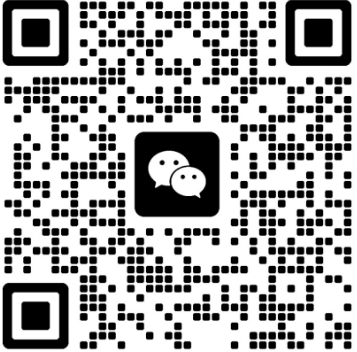