Waabi Truck Dependencies
Waabi's network of trucks contains num_trucks
number of trucks denoted by [1, 2, ..., num_trucks]. Many of these trucks depend on packages from a shipment arriving from a separate truck, before departing to make its own shipment. There are a total of m
dependencies in the truck network which are denoted by the 2d array truck_deps[][]
with shape m x 2. Each pair truck_deps[i][0], truck_deps[i][1]
denotes that the truck numbered truck_deps[i][0]
depends on the packages in the shipment from truck_deps[i][1]
. truck_deps[i][0]
must depart only after truck_deps[i][1]
has arrived. If a truck is delayed, all the trucks dependent on the previous truck and their corresponding dependencies are also delayed.
Given a list of k initially delayed trucks and the network as described, find the list of all delayed trucks. Return the list sorted in increasing order of truck numbers.
Example
Consider num_trucks = 4
, and the number of dependencies m = 2
.truck_deps = [[4, 1], [3, 2]]
The number of delayed trucks k = 2
, and delayed = [1, 3]
.
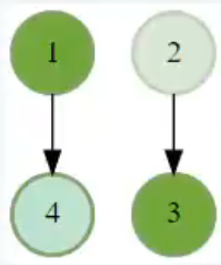
- Truck 1 is delayed.
- Truck 4 depends on truck 1, so truck 4 is delayed.
- Truck 3 is delayed.
- There are no trucks dependent on truck 3.
Return the sorted array of delayed trucks:
[1, 3, 4]
Function Description
Complete the function countDelayedTrucks
in the editor below.
countDelayedTrucks
has the following parameters:
int num_trucks
: the number of trucksint truck_deps[m][2]
: the details of the truck dependenciesint delayed[k]
: the trucks delayed initially
Returns
int[]
: the sorted list of all delayed trucks
Constraints
2 <= num_trucks <= 10^5
1 <= m <= min(num_trucks * (num_trucks - 1) / 2, 10^5)
Sample Case 0
Sample Input For Custom Testing
STDIN FUNCTION
----- --------
4 → num_trucks = 4
2 → m = 4 number of dependencies
(number of rows)
2 → this is always 2 (number of columns)
4 1 → [truck_deps[1][0], truck_deps[1][1]]
2 1
3 2
1 3
1 → delayed[] size k = 1
1 → delayed = [1]
Sample Output
1 2 3
Explanation
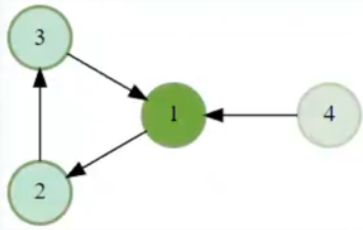
Truck 1 is delayed.
Since truck 2 depends on truck 1, it is delayed.
Since truck 3 depends on truck 2, it is delayed.
The sorted list of delayed trucks is [1, 2, 3]
.
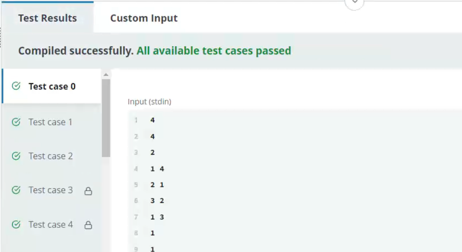
Waabi Truck Dependencies
Waabi's network of trucks contains num_trucks
number of trucks denoted by [1, 2, ..., num_trucks]. Many of these trucks depend on packages from a shipment arriving from a separate truck, before departing to make its own shipment. There are a total of m
dependencies in the truck network which are denoted by the 2d array truck_deps[][]
with shape m x 2. Each pair truck_deps[i][0], truck_deps[i][1]
denotes that the truck numbered truck_deps[i][0]
depends on the packages in the shipment from truck_deps[i][1]
. truck_deps[i][0]
must depart only after truck_deps[i][1]
has arrived. If a truck is delayed, all the trucks dependent on the previous truck and their corresponding dependencies are also delayed.
Given a list of k initially delayed trucks and the network as described, find the list of all delayed trucks. Return the list sorted in increasing order of truck numbers.
Example
Consider num_trucks = 4
, and the number of dependencies m = 2
.truck_deps = [[4, 1], [3, 2]]
The number of delayed trucks k = 2
, and delayed = [1, 3]
.
- Truck 1 is delayed.
- Truck 4 depends on truck 1, so truck 4 is delayed.
- Truck 3 is delayed.
- There are no trucks dependent on truck 3.
Return the sorted array of delayed trucks:
[1, 3, 4]
Function Description
Complete the function countDelayedTrucks
in the editor below.
countDelayedTrucks
has the following parameters:
int num_trucks
: the number of trucksint truck_deps[m][2]
: the details of the truck dependenciesint delayed[k]
: the trucks delayed initially
Returns
int[]
: the sorted list of all delayed trucks
Constraints
2 <= num_trucks <= 10^5
1 <= m <= min(num_trucks * (num_trucks - 1) / 2, 10^5)
Sample Case 0
Sample Input For Custom Testing
STDIN FUNCTION
----- --------
4 → num_trucks = 4
2 → m = 4 number of dependencies
(number of rows)
2 → this is always 2 (number of columns)
4 1 → [truck_deps[1][0], truck_deps[1][1]]
2 1
3 2
1 3
1 → delayed[] size k = 1
1 → delayed = [1]
Sample Output
1 2 3
Explanation
Truck 1 is delayed.
Since truck 2 depends on truck 1, it is delayed.
Since truck 3 depends on truck 2, it is delayed.
The sorted list of delayed trucks is [1, 2, 3]
.
Waabi Message Delivery System
In this task, a basic message delivery service is to be implemented that has a rate-limiting algorithm that drops any message that has already arrived in the last k seconds. If a message has arrived but isn't delivered due to rate limiting, it still counts as having arrived at the delivery service.
Given the integer k
, a list of messages as an array of n
strings, messages
, and a sorted integer array timestamps
representing the time at which the message arrived, for each message report the string "true"
if the message is delivered and "false"
otherwise.
Example
Suppose n = 6
, timestamps = [1, 4, 7, 10, 11, 14]
, messages = ["hello", "bye", "bye", "hello", "bye", "hello"]
, and k = 5
.
Timestamps | Message request | Last Same Message at the time | Delivered |
---|---|---|---|
1 | Hello | - | true |
4 | Bye | - | true |
7 | Bye | 4 | false |
10 | Hello | 1 | true |
11 | Bye | 7 | false |
14 | Hello | 10 | false |
Hence the answer is ["true", "true", "false", "true", "false", "false"]
.
Function Description
Complete the function getMessageStatus
in the editor below.
getMessageStatus
takes the following arguments:
int timestamp[n]
: the time at which messages arrivestring messages[n]
: the messages to be deliveredint k
: the minimum gap between same messages
Returns
string[n]
: the status of the messages
Constraints
1 <= n <= 10^5
1 <= |messages[i]| <= 15
1 <= timestamps[i] <= 10^6
- It is guaranteed that all the messages consist of lowercase English letters only.
Sample Case 0
Sample Input For Custom Testing
STDIN FUNCTION
----- --------
4 → timestamp[] size n = 4
1 1 1 11 → timestamp = [1, 1, 1, 11]
4 → messages[] size n = 4
message-2
message-2
message-3
message-2
5 → k = 5
Sample Output
true
false
true
true
Explanation
The second message is dropped as the last message it has the same id as the first and both arrive at the same time. All other messages can be delivered.
Sample Case 1
Sample Input For Custom Testing
STDIN FUNCTION
----- --------
1 → timestamp[] size n = 1
17 → timestamp = [17]
1 → messages[] size n = 1
message-3 → messages = ["message-3"]
Sample Output
true
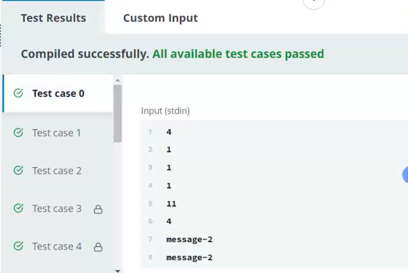
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
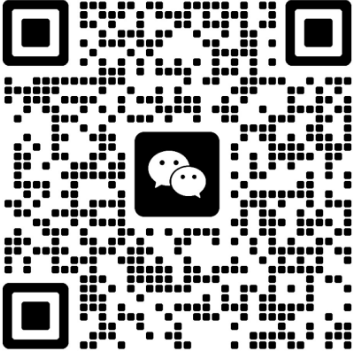