Ellen would like to assign this task to her subordinate workers
Each worker should get a distinct interval of adjacent shoes, such that the number of left shoes is equal to the number of right shoes. Each shoe must be assigned to exactly one worker.
What is the maximum number of workers that Ellen can assign to this task?
Write a function:
class Solution { public int solution(String S); }
that, given a string S
of letters "L" and "R", denoting the types of shoes in line (left or right), returns the maximum number of intervals such that each interval contains an equal number of left and right shoes.
For example, givenS = "RLRRLLRLRRLL"
the function should return 4
, because S
can be split into intervals: "RL"
, "RRLL"
, "RL"
and "RRLL"
.
Note that the intervals do not have to be of the same size.
GivenS = "RLLLRRRLLR"
the function should return 4
, because S
can be split into intervals: "RL"
, "LLRR"
, "RL"
and "LR"
.
GivenS = "LLRLRLRLRLLR"
the function should return 1
.
Write an efficient algorithm for the following assumptions:
N
is an integer within the range[2..100,000]
;- String
S
is made only of the characters'R'
and/or'L'
; - The number of letters
"L"
and"R"
in stringS
is the same.
A Caesar cipher encrypts a word by replacing each letter with another letter that is a fixed number of positions after it in the alphabet.
For example, for a cipher that replaces a letter with one that is four letters after it in the alphabet, letter A is replaced by E, letter B is replaced by F, ..., letter Y is replaced by C and letter Z is replaced by D. In other words, each letter in the alphabet is rotated to the right by four positions. For example:
PINEAPPLE is encrypted as TMRIETTPI.
The table below shows the mapping of letters when using a Caesar cipher with a rotation of 4.
A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W | X | Y |
Write a function:
class Solution { public String encrypt(String text); }
that, given a string text
, returns the string encrypted using a Caesar cipher with a rotation of 4.
Examples:
- Given text =
"PINEAPPLE"
, the function should return"TMRIETTPI"
. - Given text =
"ZEBRA"
, the function should return"DIFVE"
. - Given text =
"NETWORK"
, the function should return"RIXASVO"
.
Assume that:
- The length of string
text
is within the range [1..100]; String
text is made only of uppercase letters (A–Z).
In your solution, focus on correctness. The performance of your solution will not be the focus of the assessment.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
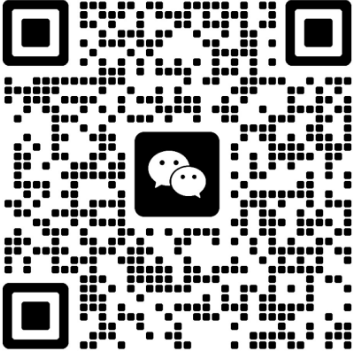