"I thought it would be a standard algorithm problem, but it turned into a technical thriller worth writing into a novel." That’s the feedback from one of our clients who recently passed a high-stakes technical interview at Meta (formerly Facebook). From anxious and disoriented to composed and focused, the candidate’s transformation was powered by CSOAHELP’s real-time remote interview assistance. This article shares the detailed journey of how our service helped him stand out and ultimately succeed.
The candidate had two years of development experience and was actively seeking a new role. After identifying Meta as his top target, he contacted us and opted for our remote support package. We ran a pre-interview tech check to ensure his devices, software, and platform—Google Meet—could seamlessly integrate with our observation and prompt system.
At 10:00 AM on the day of the interview, the video call began. Without much small talk, the interviewer immediately introduced the first problem:
“Given a binary tree, imagine yourself standing on the left side of it, return the values of the nodes you can see ordered from bottom to top, then switch to right side of the tree, and return the values of the nodes you can see ordered from top to bottom.”
The candidate was momentarily thrown off—it didn’t look like a typical LeetCode-style problem. Instead, it was a layered custom problem combining two perspectives. The real challenge here was not just writing correct code, but demonstrating practical understanding of structural traversal and dealing with follow-up complexity. At Meta, the interviewer will continue to probe—asking about your reasoning, time and space complexity, and may even present edge cases live for debugging.
Immediately after the question, CSOAHELP pushed a full-text prompt into the candidate’s side display: “Start with BFS to perform a level-order traversal of the tree, storing nodes by level. Then extract the left view from bottom to top (leftmost node of each level), and the right view from top to bottom (rightmost node of each level). Merge the two lists, removing the duplicate root node.”
Following the guide, he implemented the level_order structure. We continued providing structure for the view logic: use reversed traversal to get the left view (first element in each level), use regular order for the right view (last element in each level), and merge them carefully.
The interviewer began follow-ups: “What happens if the tree is very deep? Can your algorithm handle tens of thousands of nodes?”, “What if node values are not unique? Can your logic still work using node references instead of values?”, “What’s the space complexity here?”
Before each question was fully spoken, we had already pushed concise points: “Space complexity of BFS is O(n); you can optimize with queue management,” and “Duplicate values don’t matter here—we use position, not value.”
Next came the second problem:
“Given an array of integers (0 to 9) rearrange the positions of the array elements to represent a number which is the second largest possible.”
At first glance, this seemed like a simple permutation/sorting problem, but the Meta interviewer didn’t stop there. He followed up with: “Can your solution handle duplicates?”, “What if the array length is in the thousands—would brute force still work?”, “Can you do it in a single pass?”
We had already prepped the candidate with a simplified explanation of the next permutation algorithm. In the prompt, we advised: “Start by stating your understanding—‘find the second largest permutation.’ Suggest brute force as a starting point, then explain its limitations for large inputs, and smoothly transition to next permutation. Walk through: find the first decreasing point from the end, find the next larger element, swap, then reverse the tail. This gives O(n) time, suitable for production.”
We also provided a brute-force template for fallback:
def second_largest_permutation(arr):
arr = sorted(arr, reverse=True)
from itertools import permutations
seen = set()
count = 0
for p in permutations(arr):
num = tuple(p)
if num not in seen:
seen.add(num)
count += 1
if count == 2:
return list(num)
return []
The candidate reproduced the logic clearly, then transitioned to the optimized version. The interviewer followed up: “Can you do this without using Python’s built-in libraries?” We pushed a handwritten next-permutation breakdown. The candidate copied and explained it fluently.
Finally, the interviewer asked behavioral questions like, “Do you prefer working independently or collaborating closely?”, “Have you handled urgent projects before?” We immediately displayed a STAR framework reminder: describe the Situation, Task, Action, and Result. We even included a project keyword based on his resume—about refactoring a payment system. He narrated his story smoothly. The interviewer was visibly satisfied.
The interview lasted nearly an hour. From shaky and stuck in the beginning, to composed and articulate in the end, the transformation was powered quietly and consistently by CSOAHELP. Each follow-up question was anticipated with a text prompt. Every tricky logic point was supported. Every code structure was reinforced.
Interviews at Meta are not about brute-forcing through LeetCode questions. They are mind games and stress tests. You must not only solve the problem, but explain why you chose that path. You must demonstrate not only coding ability but strategic communication. CSOAHELP’s real-time assistance ensures that you’re never alone in the moment that matters.
We don’t speak for you. We don’t cheat. We use a secondary silent display to offer timely textual support. No interference. Just the right insight when you need it most. We help you unleash your full potential—without taking over.
If you’re preparing for Meta, Google, Apple, Stripe or any other top-tier tech interview, CSOAHELP is your secret advantage. We offer not just live support, but also mock interviews, thinking frameworks, code reviews, and communication coaching. To crack the FAANG code, it’s not about how many problems you’ve solved—it’s about who’s by your side when the pressure peaks.
Think you can survive until the last round at Meta? Leave a comment—we’ll evaluate your potential for free.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
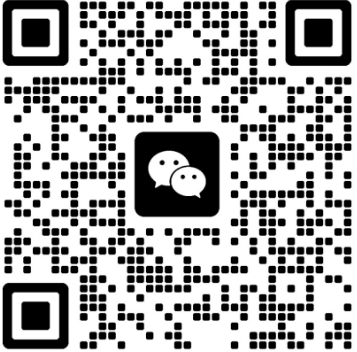