Have you ever experienced that awkward interview moment—when you fully understand the question, even have a rough idea of the solution, but the moment you start speaking, you freeze? Or worse, you try a complicated approach only to be bombarded with follow-up questions and find yourself unable to respond?
Let me share a real story with you. One of our clients was recently invited to a Microsoft technical interview. He wasn't a strong algorithmic thinker and struggled to explain his ideas in English. If he had faced the interview alone, he most likely would have failed. But he made one critical decision—he used CSOAHELP's real-time remote interview assistance service.
The interview problem was one that many candidates have seen on LeetCode or in prep books. Here’s the question:
"Given a list of meetings, where a meeting has a start and an end time, determine the minimum number of rooms required to schedule all the meetings without any conflicts. A person can also attend a meeting if it's starting time is the same as the previous meeting's ending time."
At first glance, it looks like a typical meeting room allocation problem. Many candidates immediately think of using heaps or the two-array sort and two-pointer technique. But in a real Microsoft interview, the challenge isn’t just solving the problem—it’s about clearly communicating your thought process, handling edge cases, and showing how your solution fits real-world use.
This candidate wasn't strong in algorithms and wasn’t confident in English. But with CSOAHELP's remote support, he delivered a clear, confident, and complete explanation that impressed the interviewer and made him look like a seasoned engineer.
When the interview started and the candidate heard the question, he paused, clearly nervous. We quickly pushed a prewritten clarification sentence to his secondary screen:
“I just want to confirm: if we have two meetings [1, 2] and [2, 3], can they share the same room? That is, is the start time allowed to be equal to the end time of a previous meeting?”
He read it naturally, which immediately calmed his nerves and showed the interviewer his attention to detail. The interviewer confirmed, “Yes, they can share a room,” and we moved to the next phase.
The candidate wasn’t confident explaining complex logic, so instead of offering keyword hints, we provided a fully written explanation he could repeat word-for-word. He delivered it smoothly, as if rehearsed:
“We can separate all the start times and end times into two arrays and sort both arrays individually. Then, we use two pointers to traverse the arrays. Whenever the current start time is less than the current end time, it means a new meeting starts before the previous one ends, so we need to allocate an additional room. If the start time is greater than or equal to the end time, that means a previous meeting has ended, and we can release a room. During this process, we keep track of the maximum number of rooms used, which is the final answer.”
It sounded professional, well-structured, and natural—nothing like someone who wasn’t confident just hours earlier. The interviewer nodded and asked him to write the code.
Now came the make-or-break moment. The candidate wasn’t fluent in Java syntax and might’ve fumbled this part if left on his own. But we had already prepared and pushed a clean, well-commented, complete code template to his screen. He typed it out line by line while verbally explaining what each section did.
Here’s the code he delivered:
public static int minMeetingRooms(int[][] meetings) {
int n = meetings.length;
if (n == 0) return 0;
int[] startTimes = new int[n];
int[] endTimes = new int[n];
for (int i = 0; i < n; i++) {
startTimes[i] = meetings[i][0];
endTimes[i] = meetings[i][1];
}
Arrays.sort(startTimes);
Arrays.sort(endTimes);
int currentRooms = 0;
int maxRooms = 0;
int startPtr = 0;
int endPtr = 0;
while (startPtr < n) {
if (startTimes[startPtr] < endTimes[endPtr]) {
currentRooms++;
startPtr++;
} else {
currentRooms--;
endPtr++;
}
maxRooms = Math.max(maxRooms, currentRooms);
}
return maxRooms;
}
While typing, he repeated the explanation we provided, such as: “We check if a new meeting starts before the current one ends. If so, we need a new room. If not, we can free up a room. maxRooms stores the peak number of concurrent meetings.”
After the code passed, the interviewer asked a follow-up: “What if I wanted to track which meetings are overlapping? What would you change?”
We immediately pushed a full answer for him to repeat:
“We can assign a unique ID to each meeting. Then, we use a HashSet to track ongoing meetings. For each start time, we add the meeting ID to the set. For each end time, we remove the corresponding ID. At any given moment, the set tells us which meetings are currently overlapping. We could also enrich this data with host names or meeting topics for further analysis.”
He repeated the explanation naturally and added, “This would make the system more flexible if we need to analyze meeting loads or optimize scheduling based on real-time overlap.” The interviewer responded very positively.
Then came the behavioral portion. This candidate normally struggles to answer these questions clearly. So we prepared a STAR-format story, customized from his past project experience. With that, he was able to tell a compelling story by just following the script:
“In one of my previous projects, we faced a major issue with unclear dependency order during a large-scale system migration. My task was to identify all module dependencies and establish a clear migration sequence. I organized a cross-team meeting and used a graph visualization to illustrate dependencies. Then, I created a staging environment to run phased migration tests. As a result, we completed the migration without any downtime, reducing deployment time by nearly 30%. This experience taught me how to manage complexity and lead cross-functional collaboration.”
The interviewer was impressed and asked follow-up questions, which gave the candidate more opportunities to showcase his strengths.
After the interview, the candidate told us, “Without you, I would’ve lost my rhythm right at the clarification step. I wouldn’t have made it through that calmly.” Days later, he received an invitation for the second round at Microsoft.
Most candidates treat interviews as a solo battle. They grind LeetCode, review systems design, and rehearse answers alone. But that process is stressful and often inefficient. What most don’t realize is—you don’t have to do it alone.
With CSOAHELP's real-time interview assistance, you get more than a technical hint. We help you build the complete structure of what to say, how to say it, and how to think through follow-ups. Whether it’s a full explanation script, clean working code, or STAR-format behavioral answers, we are there to back you up—live and silent, but effective.
We don’t replace your skills—we amplify them at the most critical moment.
If you’re preparing for big tech interviews and want to maximize your chances, don’t let language gaps, nerves, or structure kill your effort. Let us be your silent teammate.
You don’t have to face your next high-pressure interview alone.
Just focus on being your best.
Leave the rest to us.
CSOAHELP — real-time interview support that truly makes a difference.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
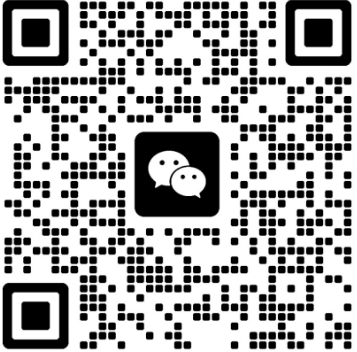