In a real DoorDash technical interview, a candidate who had limited time to prepare and wasn’t confident in their algorithm skills was presented with what many would consider a “basic” question:
/*
A
/ \
B C
/ \ / \
D (E) F G
/ \
(H) I
Node -> left, right, parents. A -> value
Input: H, E
Output: B
*/
The problem sounds simple enough:
You’re given a tree where each node not only has left and right child pointers but also a parent
pointer. Now, given two nodes (H and E in this example), return their lowest common ancestor (LCA).
Most people who’ve spent time on LeetCode might shrug and say: “I know this one!”
But in reality, this candidate didn’t.
What he did do was book a CSOAHELP Live Interview Assistance session in advance.
Throughout the entire interview, his responses impressed the interviewer. But all he did was follow our real-time cues and repeat the structured thoughts and code we provided behind the scenes.
The interviewer started: “Let’s implement a function to find the lowest common ancestor of two nodes. Each node has a parent pointer.”
The candidate froze momentarily. We jumped in via his second screen and pushed a quick prompt:
“You should first clarify if you can define a TreeNode class, and whether a node can be its own ancestor—for example, if B and E are the inputs, should the answer be B?”
The candidate followed through: “Can I define my own TreeNode class? Also, if one node is an ancestor of the other, like B and E, should I return B?”
The interviewer replied: “Yes.”
The tension eased. We nudged the next thought:
“You can use a simple approach: traverse upward from both nodes to collect their ancestors, then compare their paths from the end to find the last matching node.”
The candidate repeated smoothly: “I’ll traverse up from both nodes using the parent pointers, collect the paths, then compare from the end backwards to find the last match—that would be the LCA.”
The interviewer asked: “What’s the time and space complexity of this approach?”
We quickly pushed a complete answer:
Time complexity is O(h), where h is the tree height. Worst case O(n), average O(logn). Space complexity is also O(h) since we store both paths.
The candidate said: “Time complexity is O(h), h being the height of the tree. Worst case is O(n) if the tree is skewed, average is O(logn) for balanced trees. Space is also O(h) to store the ancestor paths.”
The interviewer nodded but continued: “What if one node is the ancestor of the other? Will your solution still work?”
We pushed a concrete example to help him visualize:
For nodes B and E, where B is the ancestor, the paths would be [B, A] and [E, B, A]. Comparing from the end still finds B as the lowest common ancestor, so the logic holds.
The candidate echoed: “Let’s say the inputs are B and E—B’s path is [B, A], and E’s is [E, B, A]. Comparing from the end, B is still correctly identified as the common ancestor.”
“Great,” the interviewer said. “Now let’s write some code.”
The candidate hesitated—this was the hardest part for him. We instantly pushed a full code skeleton, well-structured, ready to be copied line-by-line:
public static TreeNode findLowestCommonAncestor(TreeNode node1, TreeNode node2) {
if (node1 == null || node2 == null) return null;
List<TreeNode> ancestors1 = findAncestors(node1);
List<TreeNode> ancestors2 = findAncestors(node2);
int i = ancestors1.size() - 1;
int j = ancestors2.size() - 1;
TreeNode commonAncestor = null;
while (i >= 0 && j >= 0 && ancestors1.get(i) == ancestors2.get(j)) {
commonAncestor = ancestors1.get(i);
i--;
j--;
}
return commonAncestor;
}
private static List<TreeNode> findAncestors(TreeNode node) {
List<TreeNode> ancestors = new ArrayList<>();
while (node != null) {
ancestors.add(node);
node = node.parent;
}
return ancestors;
}
The candidate read it aloud as he typed it out. Everything from variable names to logic flow looked professional.
The interviewer nodded approvingly. “Good, clean structure.”
Next came a deeper test: “Try running this with H and E. What are their ancestor lists, and what’s the result?”
We had anticipated this and pushed a full dry-run breakdown:
H’s path: [H, D, B, A]
E’s path: [E, B, A]
Compare from the end: A == A, B == B, D ≠ E → return B
The candidate responded: “H’s path is [H, D, B, A], E’s is [E, B, A]. From the end: A matches, then B, then D and E don’t—so B is the LCA.”
“Good,” said the interviewer. “Any way to optimize your approach, say, in terms of space?”
The candidate paused. We immediately pushed an alternative method:
Use a HashSet to store all ancestors of one node. Then walk up the second node’s path until you find the first node present in the set.
This way, space usage drops from O(h + h) to just O(h).
We sent over a lightweight version of the optimized code:
Set<TreeNode> visited = new HashSet<>();
while (node1 != null) {
visited.add(node1);
node1 = node1.parent;
}
while (node2 != null) {
if (visited.contains(node2)) return node2;
node2 = node2.parent;
}
The candidate copied the logic and explained: “I’d store all of node1’s ancestors in a HashSet, then walk up from node2 until I find a match—that’s the LCA.”
“Nice optimization,” the interviewer said.
At the end of the interview, this candidate—by all means a mid-level one—managed to answer the question, improve it, and walk through edge cases. What he really did was follow our live, moment-to-moment coaching. He passed this DoorDash round smoothly.
He wasn’t the most technical candidate. His algorithms weren’t perfect, and his thinking wasn’t fast. But because of CSOAHELP’s live assistance, he was able to sound composed, think clearly, and build answers that worked.
This wasn’t cheating. This was structured real-time coaching that let him present what he was capable of, without getting lost under pressure. Here’s how it worked:
We listened in quietly and carefully during the interview (on a secondary device). We pushed timely prompts, explanation templates, and even full code outlines. We made sure that no matter what curveball the interviewer threw—edge cases, time/space trade-offs, optimizations—he had something confident to say.
The truth is, interviews at companies like DoorDash aren’t just about solving the problem. They’re about explaining your thought process, adapting to feedback, staying calm under pressure, and communicating clearly.
CSOAHELP becomes your silent, invisible partner. We help you say the right things, write the right code, and follow the right structure—live, as it happens.
Want to know how we helped another candidate ace a Stripe system design interview by building a “global payments architecture” on the spot? Stay tuned for the next write-up.
You don’t have to face interviews alone. With CSOAHELP, you perform like the best version of yourself.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
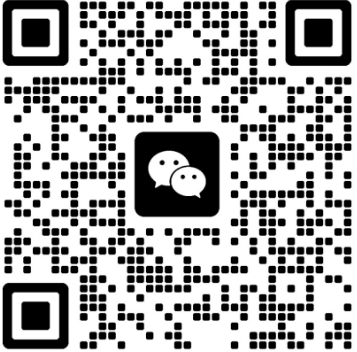