Creating a List of Elite Football Clubs in a Nation
The editor of a sports magazine must compile a list of elite football clubs in a specific nation. This requires sending HTTP GET requests to access a football club database via the following URL:
API URL:
https://jsonmock.hackerrank.com/api/football_teams?nation={nation_name}
The database provides the estimated monetary value of each club, referred to as its valuation. Since the query result is paginated, additional pages can be accessed using the query parameter &page={num}
, where num
represents the page number.
API Response Fields
The API response contains the following fields:
- page: The current page number.
- per_page: The maximum number of results per page.
- total: The total number of records.
- total_pages: The total number of pages for the query.
- data: An array of JSON objects, each containing football club details.
Data Fields in Each Club Object
Each JSON object within the data
array includes the following details:
- name: The name of the club.
- nation: The nation in which the club plays.
- estimated_value_numeric: The club's monetary valuation.
- number_of_league_titles_won: The number of league titles won by the club.
- Other details: Additional fields that are not relevant to this question.
Determining Presence of Values in a Binary Search Tree
Description
In a binary search tree (BST), each node contains a value and references to up to two child nodes: left and right. The root node has no ancestors. The left and right children represent subtrees. The BST property dictates that:
- Values in the left subtree of a node must be less than the node's value.
- Values in the right subtree of a node must be greater than or equal to the node's value.
This property enables efficient searching in the BST.
Function Description
Complete the function:
isPresent
This function takes the following parameters:
BSTreeNode root
: A reference to the root node of an integer BST.int val[q]
: An array of integers to search for in the BST.
Returns:
int[q]
: An integer array where each value at indexi
is:1
ifval[i]
is present in the BST.0
otherwise.
Constraints:
- 1≤n,q≤1051 \leq n, q \leq 10^5
- 1≤val[i]≤5×1041 \leq val[i] \leq 5 \times 10^4
Code Snippet for Reference (Java)
import java.io.*;
private static int isPresent(Node root, int val) {
// For your reference
class Node {
Node left, right;
int data;
Node(int newData) {
left = right = null;
data = newData;
}
}
}
This function checks if a list of integer values exists in a given BST, returning a corresponding list of 1
s and 0
s.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
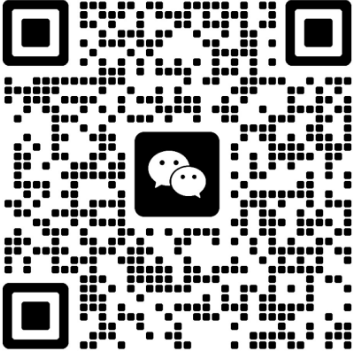