Not long ago, we helped a candidate successfully pass a real Amazon system design interview. The question wasn't the kind of "grind-LeetCode-hard" type, but rather a practical design challenge you'd likely encounter on the job. These kinds of questions are trickier—not because the algorithm is hard, but because a slip in communication, a moment of hesitation, or a missing detail can cost you dearly.
But this candidate nailed it. He not only designed the system, but also wrote clean code and explained it clearly enough to impress the interviewer.
His secret wasn't genius or luck. It was this: during the entire interview, he had CSOAHELP’s real-time silent interview support team on a second screen—guiding him with written suggestions and code snippets, helping him stay sharp and structured every step of the way.
Let me walk you through the actual interview process so you can see exactly how real-time support works, and how much of a game changer it is.
Here’s the question:
We are in charge of designing a system to install packages. We are required to support the installation of a package and all of its dependent packages.
Example Package Structure
A depends on B, C
B depends on D, E, F
C depends on F
F depends on G
Solution Design
Defining a Package
class Package {
// define what this looks like
}
Implementing the Installer
class Installer {
// solution
}
Right after the interviewer presented the question, the candidate quickly recognized it as a dependency resolution problem.
But knowing the core idea is one thing—expressing it clearly and impressively is another. So we pushed the first suggested response onto his second screen:
“I understand that this problem is fundamentally about dependency resolution. Each package may depend on other packages, so we need to ensure that dependencies are installed before installing the target package.”
Immediately after, we followed up with a second prompt to guide his algorithmic explanation:
“I plan to use depth-first search (DFS) to perform a topological sort, ensuring that each package is installed only after all its dependencies. Topological sort is the standard solution for problems modeled as a Directed Acyclic Graph (DAG).”
The candidate repeated these lines almost word for word. The logic was solid, and his confidence was obvious. The interviewer nodded and moved on: “How would you define your Package and Installer classes?”
We sensed the shift to class modeling and exception handling. So we pushed another well-crafted message:
“I would define a Package class with fields for name, dependencies, and installation status. The Installer class would register packages, manage the installation flow, and handle dependency resolution, including cycle detection.”
We also provided a code snippet that he could copy or explain:
class Package:
def __init__(self, name, dependencies=None):
self.name = name
self.dependencies = dependencies if dependencies else []
self.is_installed = False
The candidate walked through this model confidently. Then, prompted by us, he added: “I’ll include an installation status flag to avoid reinstalling packages that are already handled.”
The interviewer threw in a classic follow-up: “What if a dependency isn’t registered in the system?”
We pushed the next line instantly:
“I’ll validate dependencies before installation. If any are missing, I’ll raise an error to prevent a broken installation flow. This adds robustness to the system.”
He relayed this naturally and fluently. Exactly the kind of defensive thinking big tech companies want to see.
Then came the main implementation part—designing the actual dependency resolution logic. This is where many people stumble. You might know how it works, but in the moment, it’s easy to forget one detail or get stuck in recursion.
We knew this was the make-or-break moment, so we pushed the full strategy in clear, spoken-style English:
“I’ll use DFS within the Installer to walk through dependencies recursively. I’ll use two sets: visited
to mark completed packages, and temp_mark
to track the current DFS path and detect cycles. If a package appears in temp_mark
again, it means there’s a cycle, and I’ll raise an error.”
And we dropped in the code block that he could recite or type out as-is:
def _visit(self, package_name):
if package_name in self.visited:
return
if package_name in self.temp_mark:
raise ValueError(f"Circular dependency detected involving {package_name}")
self.temp_mark.add(package_name)
for dep in self.packages[package_name].dependencies:
if dep not in self.packages:
raise ValueError(f"Dependency {dep} not found")
self._visit(dep)
self.temp_mark.remove(package_name)
self.visited.add(package_name)
self.installation_order.append(package_name)
He explained the logic while coding it out, referencing the flow and error handling. The interviewer was clearly impressed.
Finally, the interviewer asked the inevitable system-level question: “How would you scale this to support large installation scenarios?”
Without missing a beat, we pushed the final suggestion:
“To scale this, I’d use memoization to avoid redundant dependency checks, and persist installation status using a local DB or Redis for fast access. We could also move actual installations to a background job queue for concurrency.”
The candidate elaborated on caching, persistence, asynchronous task handling, and optimized lookup. The interviewer didn’t even challenge it further—just said, “Thanks, that’s very comprehensive.”
This candidate passed because, at every critical moment, we provided: clear verbal explanation suggestions he could repeat word-for-word, code snippets he could read or modify on the fly, and guidance to align his answers with what the interviewer was truly assessing. All he had to do was speak clearly, follow the flow, and avoid breaking focus. And the interviewer had no idea he was being supported in real time.
What we offer isn’t interview cheating. It’s structured, ethical, and silent support that helps you speak like the engineer you already are.
CSOAHELP’s real-time interview assistance works through a second device. While you’re live on Zoom, Meet, or Teams with your interviewer, we quietly observe and send short written prompts—key phrases, error fixes, reminders, and code examples—to help you stay composed, thorough, and impressive.
Whether you’re interviewing with Amazon, Google, Apple, Stripe, ByteDance, or any other competitive company, we can provide tailored support for system design, algorithm, and behavioral interviews. You’ll never be caught off-guard again.
The candidate we supported made it to the next round at Amazon. Afterward, he told us, “I know I can write that DFS myself. But in a real interview, it’s hard to say all those things clearly and remember every little piece. If it weren’t for you, I probably would’ve fumbled the recursion part.”
That’s the reality for most people: you know the solution, but you forget how to explain it. You understand the concepts, but can't structure your thoughts fast enough. You could write the code—but under pressure, it slips.
That’s why we’re here.
Are you ready for your Amazon interview? Wouldn't it be nice to have someone quietly give you that one hint, that one line, that one piece of structure—just when you need it most?
Don’t take your interviews alone. Let us be your quiet backup.
CSOAHELP Real-Time Interview Support — when you sound good, it’s not just you. It’s because someone’s got your back.
Message us to learn more. Let’s get started today. The next round could be yours.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
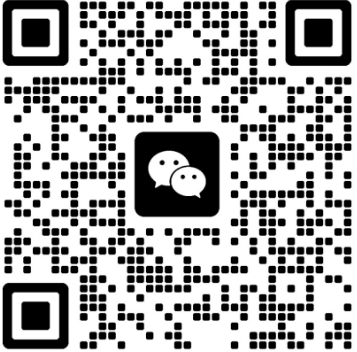