Google's technical interviews are known for their difficulty, in-depth assessments, and unexpected follow-up questions. Even experienced developers can struggle under pressure, losing clarity of thought. Today, we recreate a real-life Google interview experience and demonstrate how CSOAHELP’s live remote interview assistance helped a candidate tackle the problem seamlessly and present a perfect solution.
The candidate joined the Google Meet interview on time. After a brief exchange, the interviewer presented the question:
Question 1:
Given a company tree, calculate how many managers are paid less than the average salary of their direct and indirect employees.
For example, consider the following hierarchy:
A ($100)
|
+-- B ($100)
+-- C ($200)
|
+-- D ($60)
Here, there are two managers, A and C.
- A should be counted since the average salary of their employees is: (100+200+60)3=120\frac{(100 + 200 + 60)}{3} = 120 which is more than A’s salary ($100).
- C should NOT be counted because their salary is $200, which is more than the average salary of their employees ($60).
At first glance, this problem appears to be a simple tree traversal question. However, the candidate quickly realized that a naive approach could lead to exponential complexity if each manager required recalculating the salaries of all subordinates. After a brief pause to collect his thoughts, CSOAHELP provided a complete step-by-step solution on his secondary screen. He could simply follow this guide to structure his response clearly and maintain a smooth delivery.
CSOAHELP’s First Stage Assistance: Full Explanation and Code
Solution Approach:
This problem is best solved using Depth-First Search (DFS). Each node should recursively return:
- The total salary of all its subordinates (sum of salaries).
- The total number of employees under its management.
When returning from recursion, the manager's salary is compared with the average salary of their employees. If the manager is underpaid, they are counted.
Code Implementation:
class Employee:
def __init__(self, name, salary):
self.name = name
self.salary = salary
self.subordinates = []
def count_underpaid_managers(root):
underpaid_count = 0
def dfs(node):
nonlocal underpaid_count
if not node.subordinates:
return node.salary, 1
total_salary, total_count = 0, 0
for sub in node.subordinates:
sub_salary, sub_count = dfs(sub)
total_salary += sub_salary
total_count += sub_count
avg_salary = total_salary / total_count
if node.salary < avg_salary:
underpaid_count += 1
return total_salary + node.salary, total_count + 1
dfs(root)
return underpaid_count
The interviewer reviewed the code carefully and asked, “What is the time complexity of your solution?”
The candidate quickly analyzed the execution process and was able to confidently recite the structured response provided by CSOAHELP:
"My algorithm employs a depth-first search (DFS), visiting each employee node only once while aggregating salaries and employee counts during backtracking. Therefore, the time complexity is O(N), where N is the total number of employees."
The interviewer nodded in approval and then posed a more challenging follow-up question:
"If we need to adjust salaries to ensure that all managers are paid at least the average salary of their employees, what is the minimum amount we need to increase?"
This significantly increased the problem’s complexity. The candidate needed an optimal strategy to determine the required salary increments. At this moment, CSOAHELP promptly provided a complete breakdown and working code, allowing the candidate to quickly adapt and present a well-thought-out answer to the interviewer.
CSOAHELP’s Follow-up Assistance
Solution Approach:
This problem still follows a DFS approach, but an additional calculation is needed:
- Determine whether a manager’s salary meets the average employee salary requirement.
- If not, compute the minimum necessary salary adjustment.
To achieve this, the DFS function now returns an extra value representing the total salary increment required.
Code Implementation:
def min_extra_salary(root):
total_extra_salary = 0
def dfs(node):
nonlocal total_extra_salary
if not node.subordinates:
return node.salary, 1, 0
total_salary, total_count, extra_salary = 0, 0, 0
for sub in node.subordinates:
sub_salary, sub_count, sub_extra = dfs(sub)
total_salary += sub_salary
total_count += sub_count
extra_salary += sub_extra
avg_salary = total_salary / total_count
if node.salary < avg_salary:
extra_salary += (avg_salary - node.salary)
return total_salary + node.salary, total_count + 1, extra_salary
_, _, total_extra_salary = dfs(root)
return total_extra_salary
The candidate reiterated CSOAHELP’s explanation and highlighted key optimizations:
"In this approach, we continue using DFS, where each manager calculates the minimum salary increment needed. The overall time complexity remains O(N), avoiding redundant computations. Finally, by aggregating the returned values, we obtain the exact total salary adjustment required."
The interviewer smiled approvingly and engaged in a brief discussion on potential optimizations. The candidate successfully completed the interview round.
Why Does CSOAHELP’s Live Interview Assistance Significantly Improve Success Rates?
CSOAHELP provides complete solutions and detailed explanations during interviews, allowing candidates to quickly grasp concepts and deliver structured responses rather than struggling to piece together disjointed hints. Many candidates lose clarity under pressure, but CSOAHELP’s written guidance ensures they remain articulate and well-organized.
Google interviews often include follow-up questions designed to test a candidate’s adaptability. CSOAHELP prepares for multiple follow-up scenarios, helping candidates respond quickly and demonstrate strong engineering thinking.
If you're preparing for interviews with Google, Meta, Amazon, or other top-tier tech companies, don’t let nervousness ruin your chance. Choose CSOAHELP to ensure that every interview showcases your best performance and maximizes your chances of securing your dream offer!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
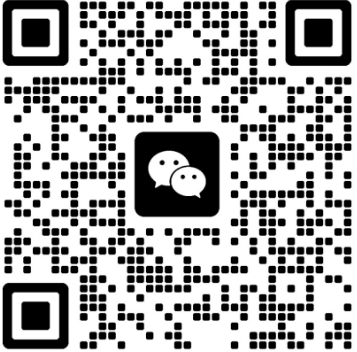