Amazon’s technical interviews are known for their high difficulty and pressure. Even experienced developers may struggle due to nerves or unclear thinking. Today, we will break down a real Amazon interview experience and show how CSOAHELP’s real-time remote assistance helped the candidate tackle algorithmic questions, system design problems, and behavioral interview challenges, ultimately leading to a successful offer.
This candidate had practiced numerous LeetCode problems and had solid development experience. However, he was still concerned about key aspects of the interview process. First, he feared mental blocks, as sometimes he understood problems but would freeze under pressure, making his solutions less clear. Second, he lacked confidence in his ability to express code fluently, worried that he might not be able to write a complete and correct solution efficiently. Finally, he felt underprepared for the behavioral interview, where Amazon follows the STAR (Situation, Task, Action, Result) method. He was concerned that his responses might not be structured and logical.
To address these concerns, he opted for CSOAHELP’s real-time remote assistance, ensuring that he would receive timely written prompts and code guidance to help him navigate the interview successfully.
The interviewer wasted no time and jumped straight into the first algorithmic question:
Q1
Given a sum of money compute the minimum number of bills and coins that equal that sum.
Assume you only have the following denominations:
- Bills: 20, 10, 5, 1
- Coins: 0.25, 0.1, 0.05, 0.01
For example, given 6.35, the solution would be One 5, One 1, One 0.25, One 0.1.
The candidate immediately recognized this as a greedy algorithm problem. He opened a virtual whiteboard and began explaining his approach.
CSOAHELP’s real-time assistance provided a complete response, allowing the candidate to articulate his thought process confidently:
“This problem can be solved using a greedy algorithm. The US currency system allows for a greedy strategy because always using the largest possible denomination first results in an optimal solution. My approach will be as follows:
- Start with the highest denomination and use as few bills and coins as possible.
- Subtract the used amount and move to the next lower denomination.
- Carefully handle floating-point precision issues to ensure accuracy.”
With this structured explanation, he proceeded to implement the solution using the complete Python code provided by CSOAHELP:
def make_change(amount):
bills = [20, 10, 5, 1]
coins = [0.25, 0.1, 0.05, 0.01]
all_denominations = bills + coins
all_denominations.sort(reverse=True)
result = {}
remaining = round(amount, 2)
for denom in all_denominations:
count = int(remaining / denom)
if count > 0:
result[denom] = count
remaining = round(remaining - count * denom, 2)
return result
The interviewer reviewed the code and asked, “What is the time complexity of your algorithm? How does it scale for large amounts?”
CSOAHELP immediately provided a full response that the candidate could repeat:
“The time complexity of this algorithm is O(n), where n is the number of available denominations. Since the number of bills and coins is fixed (8 types), the algorithm always runs in constant time, making it effectively O(1). Even for large amounts, the solution remains efficient.”
The interviewer nodded in approval, acknowledging that the solution was well-reasoned, and moved on to the next question.
Q2
You will now be given a cash drawer that defines how much of each bill and coin you have to make change with.
Now, given a sum of money and a cash drawer, compute the minimum number of bills and coins.
This problem introduced an additional challenge: limited availability of cash in the drawer. The candidate now had to ensure that his algorithm not only minimized the number of coins but also respected the actual stock of bills and coins available.
CSOAHELP provided a complete structured response that the candidate could confidently explain:
“This problem is more complex than the previous one because we need to incorporate inventory constraints into the greedy approach:
- Start with the highest available denomination, but do not exceed the quantity available in the cash drawer.
- If a denomination is insufficient, fall back on the next best option to make up the remaining amount.
- If it’s impossible to provide the exact change, return an appropriate message indicating that the cash drawer lacks the necessary funds.”
With this explanation in place, the candidate implemented the solution using CSOAHELP’s complete Python code:
def make_change_with_drawer(amount, drawer):
all_denominations = sorted(drawer.keys(), reverse=True)
result = {}
remaining = round(amount, 2)
for denom in all_denominations:
count = min(int(remaining / denom), drawer[denom])
if count > 0:
result[denom] = count
remaining = round(remaining - count * denom, 2)
return result if remaining == 0 else "Not enough cash in drawer"
The interviewer nodded in satisfaction and asked, “What happens if the cash drawer doesn’t have enough money to provide change?”
Following CSOAHELP’s guidance, the candidate replied:
“If the drawer doesn’t have enough money, I return an error message to inform the cashier that the transaction cannot be completed.”
Amazon’s interview process doesn’t just assess technical skills; communication and collaboration skills are equally important. The interviewer then transitioned into the behavioral interview, asking:
“Tell me about how you work with difficult people/stakeholders with unreasonable demands.”
CSOAHELP provided a full STAR response, allowing the candidate to articulate a well-structured answer:
Situation:
“In a previous role, I encountered a stakeholder who requested changes to the pricing algorithm’s priority. However, these changes would have negatively impacted trading operations in other regions.”
Task:
“My responsibility was to balance competing interests and ensure that business-critical functionalities were not disrupted.”
Action:
“I first gathered background information from the stakeholder to understand their objectives. I then engaged with affected teams to assess the broader impact of the requested change. After aligning with all stakeholders, I proposed a dynamic prioritization system that could adapt based on market conditions rather than manual overrides.”
Result:
“This approach eliminated conflicts between teams and streamlined decision-making by automating prioritization. It ensured all trading desks had fair and effective pricing mechanisms.”
The interviewer nodded in approval, acknowledging that the response was logical, well-articulated, and demonstrated effective problem-solving.
A few days later, the candidate received an Amazon job offer. He reflected:
“If not for CSOAHELP, I might have frozen on key questions or struggled to write clean code under pressure. The real-time assistance ensured that my thought process remained structured, my code was well-explained, and my behavioral answers met Amazon’s expectations.”
For anyone preparing for a high-stakes tech interview, CSOAHELP is your best companion for real-time guidance and structured responses. 🚀
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
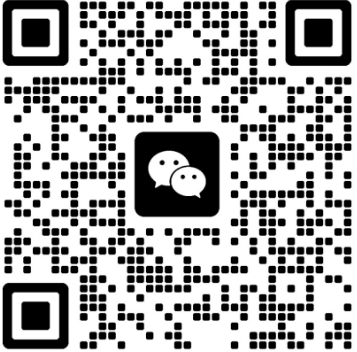