1. Counting Unique IP Addresses in Server Logs
Problem Statement
An analytics system analyzes large server log files to find the count of each unique IP address. The process should support fast lookups and insertions.
Option 1: Using an array
counts = []
for log_entry in log:
counts[log_entry.ip] += 1 # Increment count at array index of IP address
Option 2: Using a binary search tree
ip_counts = BST()
for log_entry in log:
if ip_counts.search(log_entry.ip):
ip_counts.increment(log_entry.ip)
else:
ip_counts.insert(log_entry.ip, 1)
Option 3: Using a hash map (optimal solution)
ip_counts = {}
for log_entry in log:
if log_entry.ip in ip_counts:
ip_counts[log_entry.ip] += 1
else:
ip_counts[log_entry.ip] = 1
Option 4: Using a linked list
ip_counts = LinkedList()
for log_entry in log:
node = ip_counts.search(log_entry.ip)
if node:
node.count += 1
else:
ip_counts.insert(log_entry.ip, 1)
2. Maintaining Top K Largest Elements in a Data Stream
Problem Statement
A real-time data analytics platform processes a continuous stream of numbers and must maintain the top K largest elements efficiently.
Option 1: Using an array
top_k = []
for num in stream:
top_k.append(num)
top_k.sort() # Sort array
if len(top_k) > K:
top_k.pop(0) # Remove smallest
Option 2: Using a min-heap (optimal solution)
import heapq
top_k = []
for num in stream:
if len(top_k) < K:
heapq.heappush(top_k, num)
elif num > top_k[0]:
heapq.heappushpop(top_k, num)
Option 3: Using a linked list
top_k = LinkedList()
for num in stream:
top_k.insert_sorted(num) # Insert in sorted order
if len(top_k) > K:
top_k.remove_min() # Remove smallest
Option 4: Using a stack
top_k = []
for num in stream:
top_k.append(num)
if len(top_k) > K:
top_k.pop() # Remove smallest
3. Email Read/Unread Status Tracking
Problem Statement
An email service tracks messages in a way that enables toggling between read and unread status. It primarily toggles the read/unread status of the newest messages, while older messages are rarely accessed or toggled.
Option 1: Using a stack (optimal for newest messages)
emails = []
for message in messages:
emails.append(message) # Push to stack
def toggle_latest():
emails[-1].toggle_status() # Toggle latest message
Option 2: Using a linked list
emails = LinkedList()
for message in messages:
emails.insert_at_head(message) # Insert at head
def toggle_status(msg_id):
email = emails.search(msg_id)
if email:
email.toggle_status() # Toggle status
Option 3: Using a hash map
emails = {}
for message in messages:
emails[message.id] = message # Map ID to message details
def toggle_status(msg_id):
if msg_id in emails:
emails[msg_id].toggle_status() # Toggle status in map
Option 4: Using an array
emails = []
for message in messages:
emails.append(message) # Add to array
def toggle_status():
emails.sort(key=lambda x: x.status) # Sort by read/unread
4. Remove Duplicates from Array
Write a function removeDuplicates
that removes duplicate values from an array arr
. Choose the correct implementation.
function removeDuplicates(arr) {
var unique = [];
for (num in arr) {
if (unique.indexOf(num) == -1) {
unique.push(num);
}
}
return unique;
}
Pick ONE option
if(num in arr)
if(num in unique)
if(unique.contains(num))
if(unique.index0f(num)==-1)
5. Find Missing Number in Sequence
Given an array arr
of numbers from 1 to n
with one number missing, write a function findMissingNumber
that returns the missing number. Select the correct implementation.
function findMissingNumber(arr, n) {
var total = n * (n + 1) / 2;
var sum = 0;
for (num in arr) {
sum += num;
}
return total - sum;
}
Pick ONE option
return total - sum
return sum- total
return total + sum
6.Video Decompression
TikTok is implementing a new technique for compression to efficiently store a large number of videos.
Any video can be represented as a binary string consisting only of 0
's and 1
's. The algorithm compresses a video using three numbers: x
, y
, and k
.
x
is the number of0
's in the video.y
is the number of1
's in the video.k
specifies that the video is the k-th lexicographically smallest among all videos using exactlyx
0
's andy
1
's.
Your task is to reconstruct the video corresponding to the given values of x
, y
, and k
.
Note:
A video s
is lexicographically smaller than another video t
if, at the first position where they differ, s
contains 0
while t
contains 1
.
Example
Suppose x = 3
, y = 4
, and k = 2
.
The videos formed from the given configuration include the smallest:
"0001111"
"0010111"
"0011011"
- and so on up to
"1111000"
.
Return the 2nd
lexicographically smallest video, i.e., "0010111"
.
Function Description
Complete the function decompressVideo
in the editor below.
Function Signature:
def decompressVideo(x: int, y: int, k: int) -> str:
Parameters:
int x
: The number of0
's.int y
: The number of1
's.long int k
: The lexicographic rank of the video.
Returns:
string
: Thek
th lexicographically smallest video consisting ofx
0
's andy
1
's.
Constraints:
1 ≤ x ≤ 5 * 10^3
1 ≤ y ≤ 5 * 10^3
1 ≤ k ≤ 10^18
- It is guaranteed that
k
is less than or equal to the total number of possible videos withx
0
's andy
1
's.
Sample Input for Custom Testing:
STDIN FUNCTION
----- --------
2 → x = 2
3 → y = 3
3 → k = 3
Sample Output:
01110
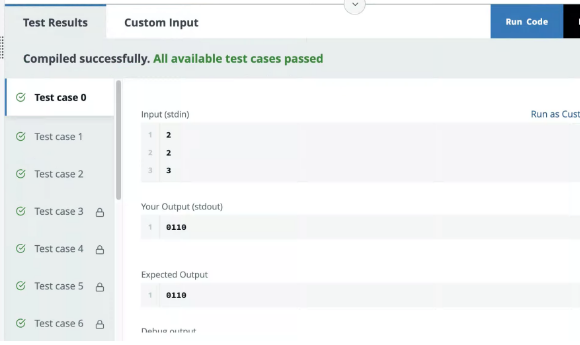
7.Risk-Aware Partition Optimization
Given n
videos posted on TikTok's platform, their risk scores (indicating potential issues like policy violations) are represented by an array videoRiskScores
. TikTok’s content moderation system needs to partition the videos into groups for review. The groups are formed by dividing the sequence of videos into subarrays, where each subarray’s size is at most k
.
The cost of evaluating risk for a group is given by the maximum risk score of any video in that group. The task is to divide the videos into groups such that the total cost of evaluating risk for all the groups is minimized, and return this minimum total cost.
Note:
A subarray is any contiguous segment of an array.
Example
Given n = 4
, videoRiskScores = [1, 2, 3, 4]
, k = 3
It is optimal to divide the entire set of videos into two groups, the first subarray is [1]
and the other one is [2, 3, 4]
.
The maximum risk scores in the partitions are 1
and 4
respectively, and therefore, the total cost for evaluating risk for both the groups is given by 5
.
Function Description
Complete the function findTotalCost
in the editor below.
Function Signature:
def findTotalCost(videoRiskScores: List[int], k: int) -> int:
Parameters:
int videoRiskScores[n]
: the risk scores of videos on TikTok platformint k
: the maximum possible length of a group
Returns:
int
: the minimum total cost for evaluating risks of videos
Constraints:
2 ≤ n ≤ 10^3
1 ≤ k ≤ 10^3
1 ≤ videoRiskScores[i] ≤ 10^3
Sample Input for Custom Testing:
Sample Case 0
STDIN FUNCTION
----- --------
6 → videoRiskScores[] size = 6
1 → videoRiskScores = [1, 3, 4, 5, 2, 6]
3 → k = 3
Sample Output:
10
Explanation:
It is optimal to divide the array into two subarrays of length 3
each.
The maximum of the first subarray is 4
, and the maximum of the second subarray is 6
.
Hence, the answer is 10
.
Sample Case 1
STDIN FUNCTION
----- --------
4 → videoRiskScores[] size = 4
1 → videoRiskScores = [1, 2, 3, 4]
1 → k = 1
Sample Output:
10
Explanation:
Since k = 1
, that is the maximum size of a group is 1
.
Therefore, risk for each video in the sequence is calculated separately and the answer is the sum of all the values of the array, videoRiskScores
, i.e. 1 + 2 + 3 + 4 = 10
.
Hence, 10
is returned as the answer.
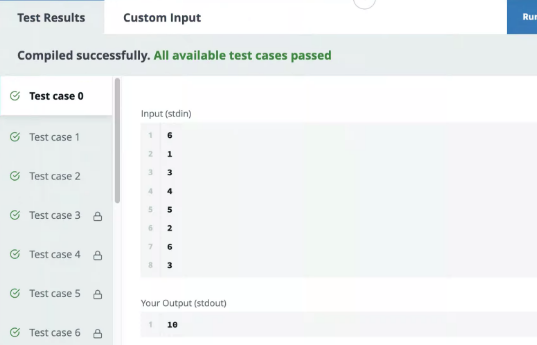
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
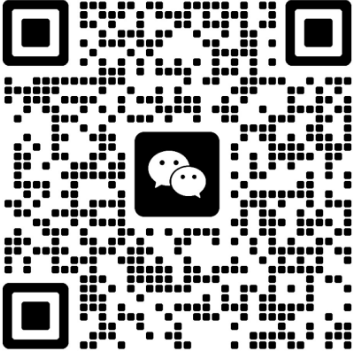