1. Minimum Total Weight
You have n chocolates with weights given in an array weights[n]
, where weights[i]
represents the weight of the iᵗʰ chocolate. Each day, you can pick one chocolate, consume half of its weight (calculated as floor(weights[i] / 2)
), and keep the remaining portion. Calculate the minimum possible total weight of the chocolates after d days. Note that you can eat from the same chocolate multiple times.
Example:
weights = [30, 20, 25]
d = 4
Day | Weight of the chocolate picked | Weight eaten | Remaining weight | Result |
---|---|---|---|---|
1 | 20 | 10 | 10 | [30, 10, 25] |
2 | 25 | 12 | 13 | [30, 10, 13] |
3 | 30 | 15 | 15 | [15, 10, 13] |
4 | 15 | 7 | 8 | [8, 10, 13] |
The total weight of chocolates on day 4 is 8 + 10 + 13 = 31
, which is the minimum possible weight after 4 days.
Function Description
Complete the function findMinWeight
in the editor below.
Function Signature:
int findMinWeight(int weights[], int d)
Parameters:
int weights[n]
: an array of integers representing weights of chocolates, indexed0
ton-1
int d
: an integer representing the number of days
Returns:
int
: the minimum total weight of chocolates afterd
days.
Constraints
1 ≤ n ≤ 10⁵
1 ≤ weights[i] ≤ 10⁴
(where0 ≤ i < n
)1 ≤ d ≤ 2 * 10⁶
2. Paint the Ceiling
You want to build yourself a house. The building company you hired can only build houses with sides from their specific set s. That means you can build a square house or a rectangular one, but only if its length and width belong to the set s.
This month, they have a special promotion: they will paint the ceiling of a new house for free, but only if its area is not more than a. You want them to do it for free, but you also want to be sure that the house will be comfortable and not too small. How many possible configurations can you create to have the ceiling painted for free given the side lengths offered?
Generating the Side Lengths
There is a method to determine what lengths of sides are available. To determine n lengths of wall segments, they start with a seed value s₀, and use variables k, b, m to generate all other side lengths s[i] using the formula:
s[i] = ((k * s[i - 1] + b) mod m) + s[i - 1], for 1 ≤ i < n
For example, given s₀ = 2, and n = 3, using k = 3, b = 3, m = 2, we calculate:
s | i | Calculation | Result |
---|---|---|---|
[2] | 1 | - | 2 |
[2, 4] | 2 | ((3 * 2 + 3) % 2) + 2 | 4 |
[2, 4, 6] | 3 | ((3 * 4 + 3) % 2) + 4 | 6 |
Now that we have the set of available lengths, we need to determine how many valid house configurations exist.
Finding Valid House Configurations
If we assume a = 15, we can brute force possible house configurations:
s₁ | s₂ | s₁ * s₂ | s₁ * s₂ ≤ a |
---|---|---|---|
2 | 2 | 4 | True |
2 | 4 | 8 | True |
2 | 6 | 12 | True |
4 | 2 | 8 | True |
4 | 4 | 16 | False |
4 | 6 | 24 | False |
6 | 2 | 12 | True |
6 | 4 | 24 | False |
6 | 6 | 36 | False |
There are 5 valid configurations that result in a free paint job.
Function Description
Complete the function variantsCount
in the editor below. The function must return an integer that denotes the number of valid variants that allow you to use the promotion.
Function Signature:
int variantsCount(int n, int s0, int k, int b, int m, long a)
Parameters:
n
: an integer, the number of wall lengths offereds0
: an integer, the length of the shortest wallk, b, m
: three arbitrary integersa
: a long integer, the largest area that will be painted for free
Constraints
1 ≤ n ≤ 2 * 10⁷
1 ≤ s[i] ≤ 10⁹
for0 ≤ i < n
1 ≤ k, b, m ≤ 10⁹
1 ≤ a ≤ 10¹⁸
Sample Case
Sample Input:
3
1
1
1
2
4
Sample Output:
6
Explanation:
With n = 3
, s0 = 1
, k = 1
, b = 1
, m = 2
, and a = 4
, we generate:
i | s[i] Calculation | s[i] |
---|---|---|
0 | Given: s₀ | 1 |
1 | ((1 * 1 + 1) % 2) + 1 | 2 |
2 | ((1 * 2 + 1) % 2) + 2 | 4 |
Possible configurations:
s₁ | s₂ | Area (s₁ * s₂) | ≤ a |
---|---|---|---|
1 | 1 | 1 | ✅ |
1 | 2 | 2 | ✅ |
1 | 4 | 4 | ✅ |
2 | 2 | 4 | ✅ |
2 | 4 | 8 | ❌ |
4 | 4 | 16 | ❌ |
A total of 6 valid configurations exist where the area is ≤ 4.
3. Maximum Order Volume
During the day, a supermarket will receive calls from customers who want to place orders. The supermarket manager knows in advance the number of calls that will be attempted, the start time, duration, and order volume for each call. Only one call can be in progress at any one time, and if a call is not answered, the caller will not call back. The manager must choose which calls to service in order to maximize order volume. Determine the maximum order volume.
Example
start = [10, 5, 15, 18, 30]
duration = [30, 12, 20, 35, 35]
volume = [50, 51, 20, 25, 10]
The above data as a table:
Caller | Start time | Duration | Order volume |
---|---|---|---|
1 | 10 | 30 | 50 |
2 | 5 | 12 | 51 |
3 | 15 | 20 | 20 |
4 | 18 | 35 | 25 |
5 | 30 | 35 | 10 |
- The first call will start at time 10 and last until 40.
- The second call will start at time 5 and last until 17.
- The third call will start at time 15 and last until 35.
- The fourth call will start at time 18 and last until 53.
- The fifth call will start at time 30 and last until 65.
The first call completely overlaps the second and third calls and partially overlaps the fourth and fifth calls. Choosing calls that do not overlap and answering the 2nd and 4th calls leads to the maximum total order volume of:
51 + 25 = 76
Function Description
Complete the function phoneCalls
in the editor below.
Function Signature:
int phoneCalls(int start[], int duration[], int volume[], int n)
Parameters:
start[n]
: an array of integers representing the start times of each callduration[n]
: an array of integers representing the durations of each callvolume[n]
: an array of integers representing the volumes of each ordern
: an integer, the number of calls
Constraints
1 ≤ n ≤ 10⁵
1 ≤ start[i] ≤ 10⁹
1 ≤ duration[i] ≤ 10⁹
1 ≤ volume[i] ≤ 10⁹
Sample Case 1
Sample Input:
3
start = [1, 2, 4]
duration = [2, 2, 1]
volume = [1, 2, 3]
Sample Output:
4
Explanation:
The calls happen in the following intervals:
Call | Interval |
---|---|
1 | [1, 3] |
2 | [2, 4] |
3 | [4, 5] |
- The first and third calls together make up the order volume 4, and their intervals do not intersect.
- The first and second calls intersect, as do the second and third calls.
- Only one call from either of these pairs can be serviced.
- The most efficient calls to answer are the first and third, with a total volume of 4.
Sample Case 2
Sample Input:
3
start = [1, 10, 100]
duration = [1, 10, 100]
volume = [1, 10, 100]
Sample Output:
111
Explanation:
The calls happen in the following intervals:
Call | Interval |
---|---|
1 | [1, 2] |
2 | [10, 20] |
3 | [100, 200] |
- All three calls can be attended, so the total volume is:
1 + 10 + 100 = 111
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
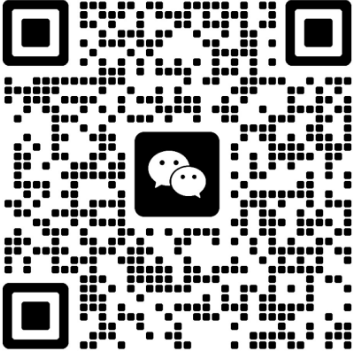