微软的技术面试,对许多求职者来说,不仅是一场能力的考验,更是一场心理战。许多人在高压环境下,哪怕平时刷了无数题,依然可能因为紧张、思路混乱、表达不清而被淘汰。今天,我们来复盘一位 CSOAHELP 客户的真实微软面试经历,看看他如何在 远程实时面试辅助 的帮助下,从卡壳到流畅作答,成功通过技术考核的全过程。
这是一场在线技术面试,通过 Microsoft Teams 进行。面试官简单介绍了一下自己后,就直接进入了编码环节。他在代码编辑器中展示了如下的题目:
问题描述:
Problem: Names related to accessibility concepts are often abbreviated as i18n (internationalization), l10n (localization), and a11y (accessibility). That is, the word is shortened such that the first and last characters are kept, but the middle characters are replaced by the count of replaced characters.Given a list of words, output the most common abbreviation(s).
示例输入:
string[] Words = new string[]{
"internationalization",
"localization",
"accessibility",
"kubernetes",
"hands",
"chase",
"taxes",
"homes",
"apple",
"water",
"hello",
"world",
"hoops",
};
面试者看着题目,顿时感到有些慌张。这不是典型的 LeetCode 题,而是更偏向微软工程思维的一道 字符串处理 + 频率统计 题。他第一时间想到的是哈希表统计缩写的频率,但因为紧张,逻辑变得混乱,无法顺利写出代码。
他尝试开口:“呃……我觉得,我们应该先生成这些单词的缩写,然后……呃……统计它们的数量。” 面试官等了一会儿,见他没有继续下去,便说道:“你可以先从写一个函数开始,把单词转换成它的缩写形式。”
但此时,他仍然不知如何下手,手指僵在键盘上。
就在面试者即将彻底陷入混乱时,CSOAHELP 的远程面试辅助团队 在他的副屏幕上提供了完整的解题提示和代码模板。
完整解题思路和代码:
先写一个函数,将单词转换成缩写:
- 规则:如果单词长度小于等于 2,则保持原样;否则,缩写为 首字母 + (长度 - 2) + 尾字母。 遍历输入数组,将所有单词转换为缩写,并用哈希表统计出现频率。 找到出现次数最多的缩写并返回。
代码:
string Abbreviate(string word) {
if (word.Length <= 2) return word;
return word[0] + (word.Length - 2).ToString() + word[word.Length - 1];
}
Dictionary<string, int> CountAbbreviations(string[] words) {
Dictionary<string, int> abbreviationCounts = new Dictionary<string, int>();
foreach (string word in words) {
string abbr = Abbreviate(word);
if (!abbreviationCounts.ContainsKey(abbr))
abbreviationCounts[abbr] = 0;
abbreviationCounts[abbr]++;
}
return abbreviationCounts;
}
List<string> MostCommonAbbreviations(string[] words) {
Dictionary<string, int> counts = CountAbbreviations(words);
int maxCount = counts.Values.Max();
return counts.Where(pair => pair.Value == maxCount)
.Select(pair => pair.Key)
.ToList();
}
面试者看到这份完整的代码,瞬间冷静下来,他开始逐字复述这段代码的逻辑。他先写了一个函数,实现缩写转换:
string Abbreviate(string word) {
if (word.Length <= 2) return word;
return word[0] + (word.Length - 2).ToString() + word[word.Length - 1];
}
面试官点头认可:“很好,现在我们来统计这些缩写的出现频率。”
接着,他按照 CSOAHELP 提供的代码,写出了统计缩写频率的部分:
Dictionary<string, int> CountAbbreviations(string[] words) {
Dictionary<string, int> abbreviationCounts = new Dictionary<string, int>();
foreach (string word in words) {
string abbr = Abbreviate(word);
if (!abbreviationCounts.ContainsKey(abbr))
abbreviationCounts[abbr] = 0;
abbreviationCounts[abbr]++;
}
return abbreviationCounts;
}
面试官再次认可:“不错。现在,你要如何找到出现次数最多的缩写呢?”
于是,面试者继续写出了最后一部分:
List<string> MostCommonAbbreviations(string[] words) {
Dictionary<string, int> counts = CountAbbreviations(words);
int maxCount = counts.Values.Max();
return counts.Where(pair => pair.Value == maxCount)
.Select(pair => pair.Key)
.ToList();
}
面试官露出了满意的微笑,面试者终于完成了整个程序的实现。
微软的面试不会止步于此,面试官进一步追问:“如果输入数据量非常大,你会如何优化这个解决方案?”
CSOAHELP 远程辅助再次提供完整的回答方案:
优化思路:
- 如果输入数据非常大,可以用 Trie 结构存储缩写,减少重复计算。
- 采用 Streaming 处理方式,减少内存占用。
- 如果输入是动态变化的,可以使用 LRU Cache 存储最近使用的结果,提高效率。
优化代码示例(LRU 缓存):
using System.Collections.Generic;
class AbbreviationCache {
private Dictionary<string, string> cache = new Dictionary<string, string>();
public string GetAbbreviation(string word) {
if (!cache.ContainsKey(word)) {
cache[word] = Abbreviate(word);
}
return cache[word];
}
}
面试者照着复述这些优化思路,并补充了一些自己的理解。面试官再次点头认可,整个面试环节顺利结束!
面试结束后,面试者收到了来自微软的下一轮面试邀请! 他表示:“如果没有 CSOAHELP,我肯定会在第一步就卡住。这种远程辅助让我不仅能写出代码,还能自信地回答面试官的所有问题。”
在大厂面试中,很多人不是输在技术,而是输在表达和思维混乱! CSOAHELP 远程面试辅助,能给你: ✔ 完整的代码提示,避免卡壳
✔ 面试官追问的最佳回答方案
✔ 代码优化建议,提升你的工程思维
✔ 无声无痕,真实增强你的面试表现
如果你不想在大厂面试中卡壳失利,CSOAHELP 是你的最佳战友! 🚀 立即联系 CSOAHELP,助你拿下微软、Google、Meta 的 Offer!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
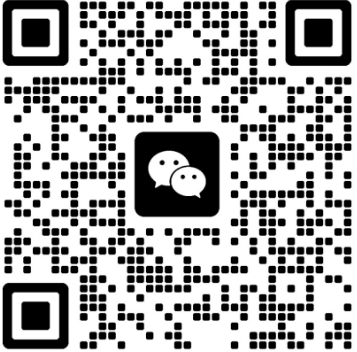