Goldman Sachs technical interviews still place a strong emphasis on algorithmic assessments. However, many candidates struggle with unfamiliar problems under pressure. Even after extensive LeetCode practice, nervousness can lead to unclear explanations, flawed implementations, and difficulties answering follow-up questions. This time, our candidate found himself in such a situation. He had never encountered this problem before and had only a basic understanding of dynamic programming. If left to tackle it alone, he would likely struggle with structuring his thoughts, implementing the code correctly, and optimizing his solution. However, with the help of CSOAHELP’s remote interview assistance, the candidate not only completed the problem successfully but also impressed the interviewer with his optimization approach.
The interviewer shared the following problem on Zoom:
Given an array of non-negative integers representing the elevations from the vertical cross-section of a range of hills, determine how many units of snow could be captured between the hills.
Example:[0, 1, 3, 0, 1, 2, 0, 4, 2, 0, 3, 0]
Solution: In this example, 13 units of snow (*) could be captured.
Implement computeSnowpack()
correctly.
The candidate hesitated for a moment, struggling to understand the problem clearly.
CSOAHELP, through a secondary screen, provided a comprehensive breakdown and solution, allowing the candidate to articulate his logic smoothly without getting lost in his thoughts. CSOAHELP offered the following structured explanation:
“We can solve this problem using a dynamic programming approach. First, we compute the maximum heights to the left and right of each position and store them in left_max
and right_max
arrays. Then, for each position, we calculate min(left_max[i], right_max[i]) - height[i]
. If this value is greater than 0, it means snow can accumulate at that position.”
The candidate repeated this explanation verbatim, and the interviewer nodded in approval.
Next, the interviewer asked him to implement the solution. Without CSOAHELP, the candidate might have overlooked edge cases (such as arrays with fewer than three elements), made logical errors (such as miscomputing left_max
and right_max
values), or spent too much time figuring out optimizations.
To help him swiftly pass this phase, CSOAHELP provided the full implementation, ensuring the candidate could type it in accurately without unnecessary mistakes.
def computeSnowpack(arr):
if not arr or len(arr) < 3:
return 0
n = len(arr)
left_max = [0] * n
right_max = [0] * n
left_max[0] = arr[0]
for i in range(1, n):
left_max[i] = max(left_max[i-1], arr[i])
right_max[n-1] = arr[n-1]
for i in range(n-2, -1, -1):
right_max[i] = max(right_max[i+1], arr[i])
total_snow = 0
for i in range(n):
total_snow += max(0, min(left_max[i], right_max[i]) - arr[i])
return total_snow
After entering the code, the interviewer asked the candidate to run some test cases. At this point, CSOAHELP continued assisting by supplying pre-written test cases, ensuring no crucial edge cases were missed.
def doTestsPass():
tests = [
[[0, 1, 3, 0, 1, 2, 0, 4, 2, 0, 3, 0], 13],
[[], 0],
[[5], 0],
[[3, 2], 0],
[[2, 0, 2], 2],
[[3, 0, 0, 2, 0, 4], 9],
[[5, 4, 3, 2, 1, 0], 0],
[[0, 1, 2, 3, 4, 5], 0],
[[5, 0, 0, 0, 0, 5], 20],
]
for test in tests:
result = computeSnowpack(test[0])
print(f"Input: {test[0]}, Expected: {test[1]}, Got: {result}")
doTestsPass()
The interviewer reviewed the solution and then asked, “What is the space complexity of your approach? Can you optimize it further?”
This was a potential stumbling block, but CSOAHELP immediately displayed a fully prepared response on the candidate’s second screen:
“The current solution uses left_max
and right_max
arrays, leading to an O(n) space complexity. We can optimize this to O(1) space by using two variables, left_max
and right_max
, instead of storing entire arrays. These variables can be updated dynamically while iterating through the array.”
The candidate repeated this explanation, and the interviewer seemed satisfied before continuing: “Can you implement this optimization?”
CSOAHELP instantly provided the optimized solution:
def computeSnowpackOptimized(arr):
if not arr or len(arr) < 3:
return 0
left, right = 0, len(arr) - 1
left_max, right_max = arr[left], arr[right]
total_snow = 0
while left < right:
if left_max < right_max:
left += 1
left_max = max(left_max, arr[left])
total_snow += max(0, left_max - arr[left])
else:
right -= 1
right_max = max(right_max, arr[right])
total_snow += max(0, right_max - arr[right])
return total_snow
With CSOAHELP’s guidance, the candidate not only answered all questions comprehensively but also successfully implemented the optimized approach, leaving a strong impression on the interviewer.
If he had gone into this interview alone, he might have:
❌ Struggled to articulate his thoughts clearly
❌ Written incorrect or inefficient code
❌ Been unable to handle follow-up questions effectively
However, with CSOAHELP, he was able to:
✅ Confidently explain the problem and solution
✅ Write error-free code with strong test cases
✅ Effectively respond to follow-up questions and demonstrate deeper understanding
Tech interviews at top companies are not just about coding skills; the real winners are those who can stay composed and perform at their best under pressure. If you want to avoid costly mistakes in your Goldman Sachs, Google, Meta, or Apple interviews, CSOAHELP is your best ally!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
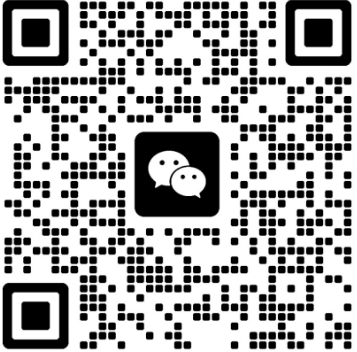