Have you ever experienced this during an interview? You’ve solved countless LeetCode problems, but when the real interview comes, your mind goes blank. You understand algorithms but struggle to articulate your thoughts clearly to the interviewer. You write the code, but the interviewer keeps pushing for optimizations, and the more you explain, the more confused you become.
Today, we’ll share the experience of a candidate who went through an Amazon interview and, with the help of CSOAHELP’s real-time interview assistance, transformed from being completely lost to confidently completing the interview and successfully moving on to the next round.
This candidate is a software engineer with three years of experience, mainly working with Python and Java. He had interviewed with several top tech companies before but kept getting rejected in the technical rounds. His main struggles were unclear logical articulation—he could think of solutions but found it difficult to explain his reasoning in a structured manner to the interviewer. His coding implementation was not well-structured—he could solve problems but didn’t follow best coding practices expected by top companies. Additionally, he lacked system design experience, often struggling when asked about databases and architectural optimizations.
This time, he finally secured an Amazon interview. However, he knew that relying solely on self-preparation might not be enough. To maximize his chances, he chose CSOAHELP’s real-time interview assistance, hoping to get precise guidance at critical moments and avoid being eliminated due to communication and coding issues.
On the interview day, he joined the Zoom meeting, exchanged brief introductions with the interviewer, and immediately moved on to the coding challenge.
Interview Question:
Create an employee directory structure that stores employee information for a company. Include manager direct reports.
Implement the following functions:
- AddEmployee
- Adds an employee to the company directory
- RemoveEmployee
- Removes an employee from the company directory
- GetDirectReports
- Returns a list of the direct reports for a given manager
- GetEmployee
- Returns the employee information
Upon seeing the problem, he suddenly panicked. He realized this was a data structure design question but wasn’t sure how to begin. Should he store employees in a list or a hash table? How should he efficiently manage the relationship between managers and direct reports? As he was trying to gather his thoughts, the interviewer asked, “Can you first describe your approach?” At this moment, he had no idea how to respond.
CSOAHELP’s real-time interview assistance team quickly provided him with a fully written response on his secondary screen, allowing him to repeat it confidently.
CSOAHELP’s provided response:
“I would use a hash table (dictionary) to store employee data, where the key is the employee_id and the value is an Employee object. Each Employee object contains employee_id, name, manager_id, and a direct_reports list to store the IDs of direct reports. This allows O(1) time complexity for looking up employee information and O(1) time complexity for retrieving a manager’s direct reports.”
He repeated this response verbatim, and the interviewer nodded, signaling him to start writing the code.
CSOAHELP provided a complete code template:
class Employee:
def __init__(self, employee_id, name, manager_id=None):
self.employee_id = employee_id
self.name = name
self.manager_id = manager_id
self.direct_reports = []
class EmployeeDirectory:
def __init__(self):
self.employees = {}
def AddEmployee(self, employee_id, name, manager_id=None):
employee = Employee(employee_id, name, manager_id)
self.employees[employee_id] = employee
if manager_id in self.employees:
self.employees[manager_id].direct_reports.append(employee_id)
def RemoveEmployee(self, employee_id):
if employee_id in self.employees:
employee = self.employees[employee_id]
if employee.manager_id and employee.manager_id in self.employees:
self.employees[employee.manager_id].direct_reports.remove(employee_id)
for report in employee.direct_reports:
self.employees[employee.manager_id].direct_reports.append(report)
del self.employees[employee_id]
def GetDirectReports(self, employee_id):
if employee_id in self.employees:
return [self.employees[emp_id].name for emp_id in self.employees[employee_id].direct_reports]
return []
def GetEmployee(self, employee_id):
return self.employees.get(employee_id, None)
He carefully copied the code provided by CSOAHELP, line by line, into the shared editor. The interviewer observed his implementation and didn’t express any dissatisfaction. Instead, they followed up with, “How would you handle the removal of a manager?”
CSOAHELP immediately provided the best response:
“When removing a manager, we should not delete their direct reports outright. Instead, we should reassign them to the next level manager, maintaining the organizational hierarchy. This prevents data loss and keeps the structure intact.”
He repeated this answer and modified the RemoveEmployee
function to ensure direct reports were properly reassigned.
The interviewer nodded again and then posed another challenge: “If this data were stored in a database instead of memory, how would you optimize queries for direct reports?”
This question caught him off guard, as he had little experience with database optimizations. But CSOAHELP promptly displayed a fully written response on his secondary screen:
CSOAHELP’s provided database optimization strategy:
“In a database setting, we can use indexing to speed up queries related to manager and direct reports relationships. Indexing employee_id and manager_id will reduce query time. Additionally, we can precompute direct reports relationships and store them in a cache (e.g., Redis) to reduce database load.”
He repeated the response confidently, and the interviewer acknowledged it with a nod before concluding the interview with, “Nice answer.”
When the Zoom meeting ended, he breathed a sigh of relief. The interviewer seemed satisfied with his performance, and he successfully advanced to the next round of the interview process.
Without CSOAHELP’s real-time interview assistance, he might have been stuck on the first question, struggling to come up with a structured response. Instead, CSOAHELP provided a complete problem-solving approach, coding implementation, and database optimization strategy, enabling him to deliver professional, well-structured answers and write code that met Amazon’s standards.
CSOAHELP transforms your interviews from stressful to confident. Real-time assistance ensures your answers are precise and professional. Full code templates allow you to simply repeat or copy them, avoiding errors due to stress. Optimized database and system design strategies help you stand out in big tech interviews.
If you want to secure an offer from Amazon, Google, Meta, Stripe, or other top companies without being hindered by nerves or lack of preparation, CSOAHELP is your best choice! Book now and let our professional team guide you to your Dream Offer!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
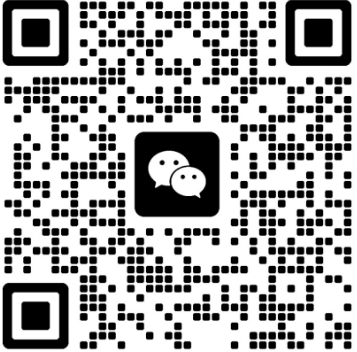