You have n chocolates with weights given in an array weights[n], where weights[i] represents the weight of the i-th chocolate. Each day, you can pick one chocolate, consume half of its weight (calculated as floor(weights[i] / 2)), and keep the remaining portion. Calculate the minimum possible total weight of the chocolates after d days. Note that you can eat from the same chocolate multiple times.
Example:
weights = [30, 20, 25]
d = 4
Day | Weight of the chocolate picked | Weight eaten | Remaining weight | Result |
---|---|---|---|---|
1 | 20 | 10 | 10 | [30, 10, 25] |
2 | 25 | 12 | 13 | [30, 10, 13] |
3 | 30 | 15 | 15 | [15, 10, 13] |
4 | 15 | 7 | 8 | [8, 10, 13] |
The total weight of chocolates on day 4 is 8 + 10 + 13, which is the minimum possible weight after 4 days.
Function Description
Complete the function findMinWeight in the editor below.
findMinWeight has the following parameters:
int weights[n]: an array of integers representing weights of chocolates, indexed 0 to n-1
int d: an integer representing the number of days
Returns
int: the minimum total weight of chocolates after d days.
Constraints
1 ≤ n ≤ 10⁵
1 ≤ weights[i] ≤ 10⁴ (where 0 ≤ i < n)
1 ≤ d ≤ 2 * 10⁵
Given a string composed of lowercase letters within the ASCII range 'a'-'z', determine the number of substrings that consist solely of vowels, where each vowel appears at least once. The vowels are ['a', 'e', 'i', 'o', 'u']. A substring is defined as a contiguous sequence of characters within the string.
Example
s = "aeioaxaeeiou"
There is a substring to the left that is made of vowels, "aeioae" which is followed by an 'x'. Since 'x' is not a vowel, it cannot be included in the substring, and this substring does not contain all of the vowels. It is not a qualifying substring. Moving to the right, there are four substrings that do qualify: "aeeiou", "aeeiuo", "aeeiou" and "aeeio".
Function Description
Complete the function vowelSubstring in the editor with the following parameter(s):
string s: a string
Returns
int: the number of substrings that consist of vowels only ('a', 'e', 'i', 'o', 'u') where every vowel appears at least once
Constraints
1 ≤ size_of(s) ≤ 10⁵
s[i] is in the range ascii['a'-'z'] (where 0 ≤ i < size_of(s))
Input Format For Custom Testing
The first line contains a string, s.
Sample Case 0
Sample Input For Custom Testing
STDIN Function
"aaeoiouxa" → s = "aaeoiouxa"
Sample Output
2
A test needs to be prepared on the HackerRank platform with questions from different sets of skills to assess candidates. Given an array, skills, of size n, where skills[i] denotes the skill type of the i-th question, select skills for the questions on the test. The skills should be grouped together as much as possible. The goal is to find the maximum length of a subsequence of skills such that there are no more than k unequal adjacent elements in the subsequence. Formally, find a subsequence of skills, call it x, of length m such that there are at most k indices where x[i] ≠ x[i+1] for all 0 ≤ i < m.
Note: A subsequence of an array is obtained by deleting several elements of the array (possibly zero or all) without changing the order of the remaining elements. For example, [1, 3, 4], [3] are subsequences of [1, 2, 3, 4], whereas [1, 5], [4, 3] are not.
Example
skills = [1, 1, 2, 3, 2, 1], k = 2.
The longest possible subsequence is x = [1, 1, 2, 2, 1]. There are only two indices where x[1] ≠ x[2] and x[3] ≠ x[4].
Return its length, 5.
Function Description
Complete the function findMaxLength in the editor below.
findMaxLength has the following parameters:
int skills[n]: the different skill types
int k: the maximum count of unequal adjacent elements
Returns
int: the maximum value of m
Constraints
1 ≤ n ≤ 2 * 10³
1 ≤ k ≤ n
1 ≤ skills[i] ≤ 2 * 10³
Input Format For Custom Testing
The first line contains an integer n, the size of the array.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
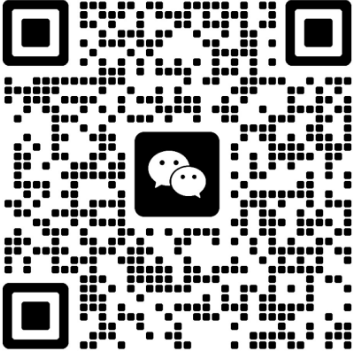