In Samsara’s technical interview, a complex Markdown parsing problem caught many candidates off guard. It tested not only basic string manipulation skills but also paragraph parsing, line break rules, blockquote nesting, and strikethrough formatting. Unlike a simple algorithm problem, this challenge assessed the candidate’s engineering mindset, requiring a balance between code readability, scalability, and efficiency.
Faced with this problem, the candidate quickly realized that this wasn’t just a straightforward string replacement task but required a structured parsing process. However, under high-pressure conditions, maintaining a clear train of thought and articulating solutions effectively became crucial. To ensure he could stay composed and methodical throughout the interview, he opted for CSOAHELP's real-time interview assistance, a service that provides live guidance during interviews. With CSOAHELP’s support, he accurately answered the interviewer’s questions and produced a high-quality implementation, allowing him to advance to the next round.
The interviewer first introduced the company’s tech stack before diving straight into the coding task: "You need to parse the following Markdown tags: <p/>
, <br/>
, <blockquote/>
, and <del/>
." The problem came with specific formatting rules: two or more consecutive newlines denote paragraph breaks, a single newline represents a soft line break (<br/>
), lines beginning with >
are blockquotes, and strikethrough text is marked by ~~
. The interviewer provided example input and expected output, emphasizing that while exact formatting wasn't required, the output should be valid HTML.
After reading the problem, the candidate grasped the general requirements, but the multi-layered nature of Markdown formatting made him hesitant. At this moment, CSOAHELP provided real-time guidance, offering a structured approach to solving the problem, helping him quickly establish a framework: iterate through the input text line by line, maintain a current_paragraph
variable to track paragraph content, wrap detected paragraphs in <p>
tags upon encountering an empty line, use a flag to determine whether a blockquote is active, replace ~~strikethrough~~
with <del>strikethrough</del>
using regular expressions, and properly insert <br/>
for soft line breaks.
After explaining his approach, the interviewer instructed him to proceed with coding. Although the candidate had a solid understanding of the solution, he was uncertain about how to efficiently implement the Markdown-to-HTML conversion. CSOAHELP immediately provided a complete code template, ensuring he could follow the structure and make necessary adjustments based on his understanding.
CSOAHELP’s Code Prompt (Candidate Could Recite or Type Directly):
import re
def markdown_to_html(markdown_text):
lines = markdown_text.split("\n")
html = []
current_paragraph = []
in_blockquote = False
def process_strikethrough(text):
return re.sub(r'~~(.*?)~~', r'<del>\1</del>', text)
for line in lines:
line = line.strip()
if line == "":
if current_paragraph:
html.append(f"<p>{''.join(current_paragraph)}</p>")
current_paragraph = []
if in_blockquote:
html.append("</blockquote>")
in_blockquote = False
continue
if line.startswith("> "):
if not in_blockquote:
if current_paragraph:
html.append(f"<p>{''.join(current_paragraph)}</p>")
current_paragraph = []
html.append("<blockquote>")
in_blockquote = True
line_content = process_strikethrough(line[2:])
if html[-1] != "<blockquote>":
html.append("<br/>")
html.append(line_content)
continue
line = process_strikethrough(line)
if current_paragraph:
current_paragraph.append("<br/>")
current_paragraph.append(line)
if current_paragraph:
html.append(f"<p>{''.join(current_paragraph)}</p>")
if in_blockquote:
html.append("</blockquote>")
return "\n".join(html)
# Test code
markdown_input = """This is a paragraph with a soft
line break.
This is another paragraph that has
> Some text that
> is in a
> block quote.
This is another paragraph with a ~~strikethrough~~ word."""
html_output = markdown_to_html(markdown_input)
print(html_output)
As the candidate entered the code, he followed CSOAHELP’s structured framework, ensuring that he fully understood the implementation. He used current_paragraph
to track ongoing text, managed blockquote sections with an in_blockquote
flag to determine when <blockquote>
should be opened or closed, and applied regular expressions to convert ~~text~~
into <del>text</del>
. The logic was clear, and the implementation met the interviewer's expectations.
Once the code ran successfully, the interviewer asked him to explain the key parts, particularly how he ensured efficiency for large-scale inputs. CSOAHELP provided a response strategy in real time, allowing him to confidently answer: using list.append()
instead of direct string concatenation to optimize performance and avoid O(n^2)
complexity, parsing Markdown line by line to minimize memory overhead, and ensuring that regular expression lookups and replacements were O(n)
, keeping operations efficient for real-world applications.
This interview wasn’t just about writing functional code but also about quickly structuring thoughts, explaining solutions effectively, and implementing clean, efficient logic under pressure. Without CSOAHELP’s real-time assistance, the candidate might have struggled with logical organization or minor implementation mistakes that could have led to failure. CSOAHELP not only provided a problem-solving framework and a working code template but also guided him in articulating his thought process and answering questions effectively, ensuring that every stage of the interview was executed flawlessly.
As tech interviews increasingly emphasize engineering thinking and problem decomposition, simply grinding LeetCode is no longer enough. The ability to remain calm under pressure, write efficient code, and clearly communicate solutions is now the determining factor in passing. CSOAHELP's real-time assistance eliminates the risk of freezing up or losing track of ideas, allowing you to systematically tackle any problem thrown at you. If you're preparing for a technical interview and don’t want stress or mental blocks to cost you an opportunity, CSOAHELP is your best choice to ensure success.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
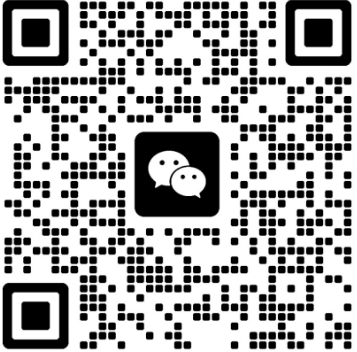