At Google, technical interviews assess more than just algorithmic skills. They evaluate problem-solving abilities, code quality, system design expertise, and how well candidates perform under pressure. Many candidates struggle with open-ended algorithmic questions due to nervousness or unclear thinking, which can lead to mistakes in coding and communication. Today, we’ll explore a real Google interview experience where a candidate successfully navigated their coding interview with the support of CSOAHELP Real-Time Interview Assistance, from problem understanding to code implementation, optimization, and system design.
The candidate, a software engineer with four years of Java experience, was applying for a Software Engineer (SDE) position at Google. He had prepared extensively, including practicing algorithmic problems, studying system design, and doing mock interviews. However, he was still concerned about the possibility of stumbling over a question due to anxiety or an unclear thought process. To ensure a smooth and confident performance, he opted for CSOAHELP Real-Time Interview Assistance, where expert professionals provided live textual guidance and complete code suggestions throughout the interview.
After a brief introduction, the interviewer presented the coding problem.
Amazon is releasing a feature called "Stuff Your Friends Are Buying."
Implement a function that returns items that a person’s friends are buying but that the person has not bought, ordered by popularity.
The interviewer also provided the following API signatures:
/**
* Returns a list of purchases of the person. If purchased multiple times, it will be in the list multiple times.
*/
List<Purchase> getPurchases(Person person);
/**
* Returns a list of friends of the person.
*/
List<Person> getFriends(Person person);
The candidate’s initial approach was to retrieve the user’s list of friends, count the purchases made by those friends, filter out any items already purchased by the user, and then sort the remaining items based on popularity. Since the interviewer did not specify the exact structure of the purchase records, the candidate hesitated momentarily. CSOAHELP Real-Time Assistance immediately provided a complete solution, enabling the candidate to confidently explain the approach and demonstrate a structured coding process.
public List<String> getRecommendedItems(Person person) {
Map<String, Integer> itemCount = new HashMap<>();
Set<String> purchasedItems = new HashSet<>();
// Retrieve the purchases of the current user
for (Purchase p : getPurchases(person)) {
purchasedItems.add(p.getItemName());
}
// Iterate over friends and count the items they have purchased
for (Person friend : getFriends(person)) {
for (Purchase p : getPurchases(friend)) {
String item = p.getItemName();
if (!purchasedItems.contains(item)) {
itemCount.put(item, itemCount.getOrDefault(item, 0) + 1);
}
}
}
// Sort items by purchase frequency
List<String> recommendedItems = new ArrayList<>(itemCount.keySet());
recommendedItems.sort((a, b) -> itemCount.get(b) - itemCount.get(a));
return recommendedItems;
}
With CSOAHELP, the candidate could clearly articulate this approach without unnecessary pauses, ensuring a smooth and structured explanation. The interviewer acknowledged the solution but then raised an additional challenge: "How does your code perform on large-scale data? If a person has a million friends and each friend buys thousands of items, will your code remain efficient?"
The candidate momentarily hesitated, unsure how to respond. CSOAHELP immediately provided a fully optimized solution, helping him articulate a performance-improved version of the code.
public List<String> getRecommendedItemsOptimized(Person person) {
Map<String, Integer> itemCount = new ConcurrentHashMap<>();
Set<String> purchasedItems = getPurchases(person).stream()
.map(Purchase::getItemName)
.collect(Collectors.toSet());
// Process friends’ purchase data in parallel
getFriends(person).parallelStream().forEach(friend -> {
getPurchases(friend).forEach(p -> {
String item = p.getItemName();
if (!purchasedItems.contains(item)) {
itemCount.merge(item, 1, Integer::sum);
}
});
});
return itemCount.entrySet().stream()
.sorted((a, b) -> b.getValue() - a.getValue())
.map(Map.Entry::getKey)
.collect(Collectors.toList());
}
With CSOAHELP’s guidance, the candidate smoothly described the optimization techniques, leveraging Java’s Stream API to improve efficiency. The interviewer was impressed with the improved approach.
At Google, interviews don’t just test coding implementation; they also assess how solutions can be scaled to large-scale system designs. The interviewer followed up with another challenge: "If Amazon has billions of users, how would you efficiently compute these recommendations in a distributed environment?"
The candidate had limited experience with distributed computing but CSOAHELP instantly provided a well-structured answer, allowing the candidate to successfully navigate this advanced discussion.
CSOAHELP’s suggested system design approach included:
- Using Kafka + Spark Streaming to process real-time purchase data
- Leveraging Redis for caching and reducing database queries
- Utilizing Hadoop/BigQuery for batch recommendation computation
- Deploying Elasticsearch for real-time querying and improved user experience
As the interviewer probed further, the candidate repeated and elaborated on these concepts, explaining how to ensure data consistency under high concurrency. His structured and insightful response earned positive feedback from the interviewer.
The candidate successfully advanced to the next round at Google, and CSOAHELP Real-Time Interview Assistance played a crucial role in this achievement. Complete code suggestions allowed the candidate to smoothly implement solutions without hesitation. Optimized techniques ensured he could confidently handle performance-related questions. Structured system design insights helped him navigate high-level discussions, demonstrating strong engineering thinking.
For software engineers aiming to secure offers from Google, Amazon, Meta, and other top tech companies, brute-force problem-solving is no longer enough. FAANG interviews focus on evaluating problem-solving skills, adaptability under pressure, and the ability to communicate effectively. If you’re looking for professional support to maximize your interview success, CSOAHELP’s Real-Time Interview Assistance ensures you’re fully prepared to tackle challenges, handle tough questions, and secure that coveted tech job offer. 🚀
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
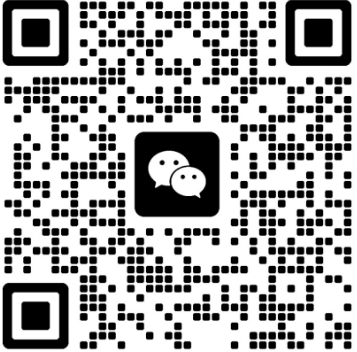