Many people believe that Amazon's interviews are no longer as difficult as they used to be, especially since their algorithmic questions seem more "friendly" compared to other FAANG companies. But does that really mean Amazon interviews have become easier? If you've ever gone through a real Amazon interview, you'll quickly realize that interviewers are not just assessing your algorithmic skills but also your problem decomposition ability, logical reasoning, code quality, communication skills, and even your psychological resilience.
Today, we will walk through a real Amazon interview experience and see how CSOAHELP remote interview assistance helped a candidate successfully navigate the process.
The Amazon interviewer opened a shared document on Zoom and presented the algorithm question.
"There are N products, and we don’t know exactly how many categories there are and which product belongs to which category. We are, however, aware that certain pairs of products belong in the same category. So, we are given a list of such product ID pairs that identify products belonging to a same category.
For example: (1, 5), (7, 2), (3, 4), (4, 8), (6, 3), (5, 2). When we analyze that list, we can see that we only have two categories with 4 products each: (1, 2, 5, 7), (3, 4, 6, 8).
The questions are: How many categories we have? How many products in each category?"
The problem essentially provides a set of product ID pairs that indicate which products belong to the same category. The goal is to determine the number of product groups and the number of products in each group.
The candidate read through the problem and started thinking about a suitable data structure. However, since the problem does not specify the total number of products and only provides a set of relationships, they were initially unsure how to initialize the data storage structure. CSOAHELP remote assistance immediately provided a detailed text prompt on their second screen: "Model this as a graph connected components problem. Each product is a node, and each given pair represents an edge. You need to find all connected components."
The candidate quickly realized that Depth-First Search (DFS) or Breadth-First Search (BFS) could be used to traverse the entire graph and identify connected product categories. The interviewer waited a moment before asking, "How do you plan to solve this problem?"
CSOAHELP instantly provided a complete response template, which the candidate repeated: "I will use defaultdict
to construct an undirected graph, treating each product ID as a node and the given product pairs as edges. Then, I will recursively traverse the graph using DFS to identify all connected components and count the number of products in each category."
The interviewer signaled for the candidate to begin coding.
Without CSOAHELP, the candidate might have struggled with:
- Confusion about how to structure the data
- Forgetting to handle the possibility of non-sequential product IDs
- Realizing midway through coding that their approach was unclear, requiring major revisions
But with CSOAHELP's guidance, the candidate received a fully structured code template, ensuring a clear and effective implementation:
from collections import defaultdict
def findCategories(pairs):
graph = defaultdict(set)
for a, b in pairs:
graph[a].add(b)
graph[b].add(a)
visited = set()
categories = []
def dfs(node, category):
if node in visited:
return
visited.add(node)
category.append(node)
for neighbor in graph[node]:
dfs(neighbor, category)
for product in graph:
if product not in visited:
category = []
dfs(product, category)
categories.append(category)
return categories
pairs = [(1, 5), (7, 2), (3, 4), (4, 8), (6, 3), (5, 2)]
categories = findCategories(pairs)
print(f"Total categories: {len(categories)}")
for i, category in enumerate(categories, 1):
print(f"Category {i}: {category}, Size: {len(category)}")
The interviewer asked the candidate to run the code, and it correctly output two categories, each containing four products. The interviewer then followed up with, "What happens if the dataset is very large, say with hundreds of thousands of products? How does your code perform?"
CSOAHELP quickly provided a detailed explanation, allowing the candidate to confidently respond: "The time complexity of this code is O(N + E), where N is the number of products and E is the number of given pairs (edges). Constructing the graph takes O(E), and DFS traversal requires O(N) since each node is visited only once. Thus, the overall complexity is O(N + E), making it efficient for large datasets."
The interviewer nodded in approval before asking, "If these product relationships change dynamically, meaning new products can be added or removed during runtime, how would you optimize your solution?"
The candidate hesitated for a moment, but CSOAHELP immediately provided a possible solution, displaying a full explanation on their second screen: "To support dynamic updates, we can use the Union-Find (Disjoint Set) data structure instead of DFS. This will allow us to efficiently merge new product categories using path compression, achieving O(1) find operations."
Following CSOAHELP's suggestion, the candidate explained how Union-Find would be a better choice for handling dynamic updates and gave real-world examples, such as merging social network friend groups or classifying similar items in recommendation systems. The interviewer seemed intrigued and asked, "If you were to implement this using Union-Find, how would you modify your code?"
CSOAHELP instantly provided the Union-Find version of the solution, and the candidate copied it while explaining its logic:
class UnionFind:
def __init__(self):
self.parent = {}
def find(self, node):
if self.parent[node] != node:
self.parent[node] = self.find(self.parent[node]) # Path compression
return self.parent[node]
def union(self, a, b):
rootA = self.find(a)
rootB = self.find(b)
if rootA != rootB:
self.parent[rootB] = rootA # Merge sets
def findCategories(pairs):
uf = UnionFind()
for a, b in pairs:
if a not in uf.parent:
uf.parent[a] = a
if b not in uf.parent:
uf.parent[b] = b
uf.union(a, b)
categories = {}
for node in uf.parent:
root = uf.find(node)
if root not in categories:
categories[root] = []
categories[root].append(node)
return list(categories.values())
pairs = [(1, 5), (7, 2), (3, 4), (4, 8), (6, 3), (5, 2)]
print(findCategories(pairs))
The interviewer was satisfied with the optimized approach and asked a final question: "How do you think you performed in this interview?"
The candidate smiled and said, "I was a bit nervous, but I think my thought process was clear and my solutions were reasonable."
Without CSOAHELP, the candidate might have struggled with code optimization, performance analysis, and responding to follow-up questions. But with CSOAHELP's real-time remote assistance, they not only completed the basic solution but also provided optimized approaches under pressure, successfully impressing the interviewer.
Has the Amazon interview really become easier? No, it has just become more subtle. CSOAHELP remote interview assistance can help you stay clear-headed in crucial moments, allowing you to excel in Amazon’s high-pressure interview process.
🚀 Ready to ace your next Amazon interview? Book CSOAHELP now and take the stress out of technical interviews!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
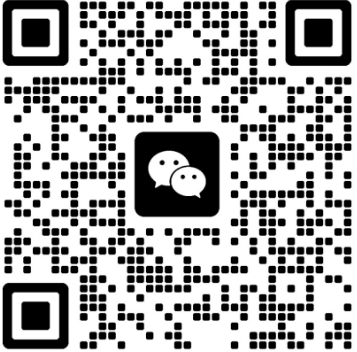