Given n images to process, where each image requires specific filters applied for a defined time frame. The cost to apply filters to the i-th image is filterCost[i]. Each image must undergo processing from the start day, startDay[i], to the end day, endDay[i] (inclusive), where 0 ≤ i < n.
There is an exclusive offer that a filter may be applied to all n images at a discounted rate, discountPrice, per day.
Devise a strategy to minimize costs and apply the necessary filters to each image during its designated processing period. The goal is to create an efficient image processing plan that adheres to time constraints and budget considerations. Return the minimum cost modulo (10⁹ + 7).
Example
Given n = 3,
filterCost = [2, 3, 4],
startDay = [1, 1, 2],
endDay = [2, 3, 4],
discountPrice = 6.
Day-wise breakdown:
Day | Images | Total Cost |
---|---|---|
1 | [1, 2] | 2 + 3 = 5 |
2 | [1, 2, 3] | 2 + 3 + 4 = 9 |
3 | [2, 3] | 3 + 4 = 7 |
4 | [3] | 4 |
Applying filters on all the images at 6 per day on the 2nd and 3rd day will be optimal.
The modulo of the final cost is 5 + 6 + 6 + 4 = 21.
Function Description
Complete the function getMinProcessingCost in the editor below.
Function signature:
def getMinProcessingCost(filterCost: List[int], startDay: List[int], endDay: List[int], discountPrice: int) -> int:
Parameters:
- filterCost[n]: the cost of filtering each image for processing.
- startDay[n]: the first day each image should be processed.
- endDay[n]: the last day each image should be processed.
- discountPrice: the discounted rate at which filters can be applied to all the images on one day.
Returns:
- The minimum cost modulo (10⁹ + 7).
A cyber attack involves different levels of security systems. The attack has n levels and can be initiated at any level i with an initial energy reserve of k. On an i-th level, the attack must breach the security system's layers[i] units of protection at a cost of layers[i] units of energy. If there is not enough energy left, the attack fails, and the attack ends. If after breaching an i-th level, the remaining energy reserve is at least energy[i], one point is awarded to the attacker.
Given the arrays layers and energy, and an integer k, starting from each level i from 1 to n, find the number of points that the attacker can score.
Example
Given, n = 3,
layers = [5, 8, 1],
energy = [4, 2, 1],
k = 10.
Attack breakdown:
Start Level | Attack | Max. Points |
---|---|---|
1 | Level 1: Energy Left = 10, layers = 5, Remaining Energy = 5 → Point awarded (remaining energy ≥ 4) → Level 2: Energy Left = 5, layers = 8 (attack fails) | 1 |
2 | Level 2: Energy Left = 10, layers = 8, Remaining Energy = 2 → Point awarded (remaining energy ≥ 2) → Level 3: Energy Left = 2, layers = 1, Remaining Energy = 1 → Point awarded (remaining energy ≥ 1) | 2 |
3 | Level 3: Energy Left = 10, layers = 1, Remaining Energy = 9 → Point awarded (remaining energy ≥ 1) | 1 |
Final Answer:
[1, 2, 1].
- Maximum Order Volume
During the day, a supermarket will receive calls from customers who want to place orders. The supermarket manager knows in advance the number of calls that will be attempted, the start time, duration, and order volume for each call. Only one call can be in progress at any one time, and if a call is not answered, the caller will not call back. The manager must choose which calls to service in order to maximize order volume. Determine the maximum order volume.
Example
Given:
start = [10, 5, 15, 18, 30]
duration = [30, 12, 20, 35, 35]
volume = [50, 51, 20, 25, 10]
Table Representation:
Caller | Start time | Duration | Order volume |
---|---|---|---|
1 | 10 | 30 | 50 |
2 | 5 | 12 | 51 |
3 | 15 | 20 | 20 |
4 | 18 | 35 | 25 |
5 | 30 | 35 | 10 |
- The first call will start at time = 10, and last until time = 40.
- The second call will start at time = 5, and last until time = 17.
- The third call will start at time = 15, and last until time = 35.
- The fourth call will start at time = 18, and last until time = 53.
- The fifth call will start at time = 30, and last until time = 65.
The first call completely overlaps the second and third calls, and partially overlaps the fourth and fifth calls. Choosing calls that do not overlap, and answering the 2nd and 4th calls leads to the maximum total order volume of 51 + 25 = 76.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
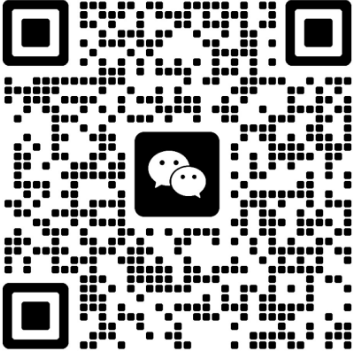