Similar Password
A password detection system for HackerRank accounts detects a password as similar if the number of vowels is equal to the number of consonants in the password.
Passwords consist of lowercase English characters only, and vowels are {'a', 'e', 'i', 'o', 'u'}.
To check the strength of a password and how easily it can be hacked, some manipulations can be made to the password. In one operation, any character of the string can either be incremented or decremented. For example, 'f' can be incremented to 'g', or decremented to 'e'. Note that character 'a' cannot be decremented and 'z' cannot be incremented.
Find the minimum number of operations in which the password can be made similar.
Example
Consider password = "hack"
. The 'h' can be changed to 'i' in one operation. The resultant string is "iack"
which has 2 vowels ('i', 'a') and 2 consonants ('c', 'k') and hence the string is similar. Return 1, the minimum number of operations required.
Function Description
Complete the function countMinimumOperations in the editor below.
countMinimumOperations has the following parameter:
- string password: the password
Returns
- int: the minimum number of operations required to make the password similar
Constraints
- 2 ≤ |password| ≤ 3 * 10^5
- It is guaranteed that the length of the password is even.
- The given string consists of lowercase Latin characters only.
Sample Input for Custom Testing
STDIN
FUNCTION
abcd → password = "abcd"
Sample Output
1
Explanation
In one operation, 'd' can be changed to 'e'. The resultant string is "abce"
which has an equal number of vowels and consonants.
Counting Triplets
There is an integer array arr[n] and an integer value d. The array is indexed from 1 to n.
Count the number of distinct triplets (i, j, k) such that 0 < i < j < k ≤ n and the sum (a[i] + a[j] + a[k]) is divisible by d.
Example
a = [3, 3, 4, 7, 8]
d = 5
The following triplets are divisible by d = 5. Following are the triplets whose sum is divisible by d (1-based indexing):
- Indices (1, 2, 3), sum = 3 + 3 + 4 = 10
- Indices (1, 3, 5), sum = 3 + 4 + 8 = 15
- Indices (2, 3, 4), sum = 3 + 4 + 8 = 15
Since there is no other triplet divisible by d = 5, return 3.
Function Description
Complete the function getTripletCount in the editor below.
getTripletCount has the following parameters:
- int a[n]: an array of integers
- int d: an integer
Returns
- int: the number of distinct triplets
Constraints
- 3 ≤ n ≤ 10^3
- 1 ≤ a[i] ≤ 10^9
- 2 ≤ d ≤ 10^6
Sample Input For Custom Testing
STDIN
FUNCTION
4 → n = 4
2 3 1 6 → a = [2, 3, 1, 6]
3 → d = 3
Sample Output
2
Explanation
- Indices (1, 2, 3), sum = 2 + 3 + 1 = 6
- Indices (1, 3, 4), sum = 2 + 1 + 6 = 9
Job Execution
There are n jobs that can be executed in parallel on a processor, where the execution time of the iᵗʰ job is executionTime[i]. To speed up execution, the following strategy is used.
In one operation, a job is chosen, the major job, and is executed for x seconds. All other jobs are executed for y seconds where y < x.
A job is complete when it has been executed for at least executionTime[i] seconds, then it exits the pool. Find the minimum number of operations in which the processor can completely execute all the jobs if run optimally.
Example
Consider n = 5, executionTime = [3, 4, 7, 6], x = 4 and y = 2.
The following strategy is optimal using 1-based indexing.
- Choose job 4 as the major job and reduce the execution times of job 4 by x = 4 and other jobs by y = 2.
- Now executionTime = [2, 1, 3, 4]. Job 3 is complete, so it is removed.
- Choose job 4 as the major job again and reduce executionTime = [1, 0, 1, 2].
- Job 2 is complete, so jobs 1, 2, and 4 are now complete.
- Choose job 5, executionTime = [−1, −1, −1, −2].
- Job 5 is complete.
It takes 3 operations to execute all the jobs so the answer is 3.
Function Description
Complete the function getMinimumOperations in the editor below.
getMinimumOperations has the following parameters:
- int executionTime[n]: the execution times of each job
- int x: the time for which the major job is executed
- int y: the time for which all other jobs are executed
Returns
- int: the minimum number of operations in which the processor can complete the jobs
Constraints
- 1 ≤ n ≤ 10⁵
- 1 ≤ executionTime[i] ≤ 10⁹
- 1 ≤ y < x ≤ 10⁹
Sample Input For Custom Testing
STDIN
FUNCTION
executionTime[] size, n = 5
executionTime[] = [3, 4, 7, 6, 9]
x = 3
y = 1
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
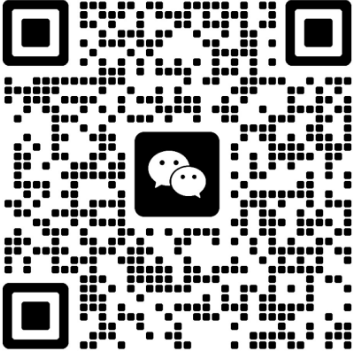