Amazon's technical interviews are known for their high pressure and difficulty. Candidates need to demonstrate deep expertise in algorithms, data structures, system design, and problem-solving while effectively communicating their thought process under tight time constraints. However, the harsh reality is that many promising candidates, despite their extensive preparation, struggle with nervousness, suboptimal problem-solving approaches, or difficulty articulating their thoughts clearly. As a result, they lose out on opportunities they were otherwise qualified for. This is where CSOAHELP's real-time remote interview assistance becomes invaluable—helping candidates stay composed, structure their responses, and tackle challenges effectively in high-stakes interviews.
Let's analyze a real Amazon interview question and see how CSOAHELP's support can significantly enhance your performance.
Amazon Real Interview Question
"We're trying to build an information retrieval system for bot prompts. Information matches can exist in multiple data sources where-in each data source responds back to your service with a sorted list of matches. We now need functionality to take these individual sorted lists and output a single sorted list that merges all of them."
Input: List<List> sortedLists
Output: List
Example:
Input: [[1,4,5],[1,3,4],[2,6]]
Output: [1,1,2,3,4,4,5,6]
This problem is essentially merging multiple sorted linked lists (or arrays), a well-known problem in algorithm interviews. Many candidates, under pressure, may resort to the brute-force approach—concatenating all lists and sorting them. The initial implementation might look like this:
def mergeSortedLists(sortedLists):
result = []
for lst in sortedLists:
result.extend(lst)
return sorted(result)
Time Complexity: O(N log N), which is not optimal! An interviewer will likely challenge this approach, asking for a more efficient solution.
If a candidate is unprepared for follow-up questions, they might struggle to come up with an optimized solution. At this critical juncture, CSOAHELP's real-time assistance can be the game-changer. Our algorithm experts provide instant guidance through voice prompts, nudging candidates towards optimal solutions and ensuring they refine their approach in real-time. With our support, candidates quickly realize that a Min Heap (Priority Queue) is a more efficient solution that brings down the time complexity to O(N log k) (where N is the total number of elements and k is the number of lists).
Optimal Solution: Using Min Heap (PriorityQueue)
The core idea is to maintain a min heap that holds the smallest elements from each list and extract the minimum at each step while maintaining sorted order.
Optimized Python Code:
from heapq import heappush, heappop
def mergeSortedLists(sortedLists):
min_heap = []
result = []
for lst in sortedLists:
if lst:
heappush(min_heap, (lst[0], lst, 1))
while min_heap:
val, lst, index = heappop(min_heap)
result.append(val)
if index < len(lst):
heappush(min_heap, (lst[index], lst, index + 1))
return result
Time Complexity: O(N log k), which is significantly more efficient than the brute-force approach.
Handling Follow-Up Questions with CSOAHELP's Real-Time Guidance
Even if a candidate derives the optimal solution, Amazon interviewers frequently push further:
- "Can you solve this in O(N) time complexity?"
- "How would you handle massive datasets (millions of elements) efficiently?"
- "What if this data is streamed dynamically—how would you adjust your approach?"
- "Besides Min Heap, can you suggest alternative data structures for solving this problem?"
Many candidates crumble under these follow-up questions due to stress and lack of structured thinking. This is where CSOAHELP’s real-time assistance ensures candidates stay composed, articulate clear responses, and avoid losing momentum.
For example, when asked, "How would you optimize memory usage for extremely large datasets?" our expert support provides instant voice prompts such as:
- "Try using iterators instead of loading all data at once."
- "Mention lazy loading to optimize memory consumption."
- "Suggest streaming-based processing instead of storing all elements upfront."
Why Real-Time Remote Assistance is Critical for Success
1. Instant Guidance for Optimal Solutions
When faced with pressure, even the most prepared candidates might blank out. CSOAHELP ensures you remember and apply the best algorithms effectively.
2. Handling Follow-Up Questions Confidently
Interviewers dig deep, and hesitation can cost you the role. We help you tackle layered questioning with structured responses.
3. Error Correction in Real-Time
Even experienced developers make minor syntax mistakes or miss edge cases. Our team ensures that your code remains error-free.
4. Improved Communication & Clarity
Amazon values candidates who can clearly express their thought process. We refine your explanations, making them crisp and persuasive.
CSOAHELP: Your Partner in Cracking Amazon’s Toughest Interviews
Amazon, Google, and Meta demand perfection in technical interviews. Merely practicing problems isn’t enough—you need to excel under pressure. Many talented developers fail not because of a lack of knowledge, but due to nervousness, disorganized answers, or suboptimal problem-solving approaches.
With CSOAHELP’s real-time remote interview assistance, you gain:
- Live prompts guiding you to the best solutions
- Strategies to confidently tackle follow-up questions
- Code optimization and error rectification in real-time
- Enhanced communication techniques to impress interviewers
You focus on solving the problem—we handle the rest.
Let CSOAHELP be the edge you need to land your dream job at Amazon, Google, or any top-tier tech company!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
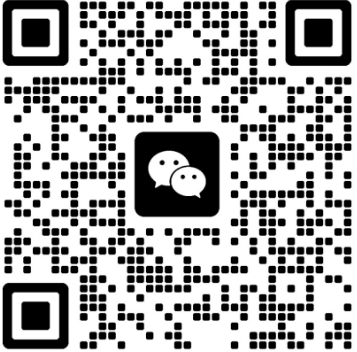