Substring Matching with Prefix and Suffix Scores
Given three strings: text
, prefixString
, and suffixString
, find:
- Prefix score: The longest substring of
text
that matches the end ofprefixString
. - Suffix score: The longest substring of
text
that matches the start ofsuffixString
. - Total score: The sum of the prefix score and the suffix score.
Return the substring of text
that:
- Begins with the matched prefix.
- Ends with the matched suffix.
- Has the highest total score.
Example
Input:
text = "engine"
prefixString = "raven"
suffixString = "ginkgo"
Processing:
"engine"
matches"raven"
at the end, soprefixScore = 2
("ne"
)."engine"
matches"ginkgo"
at the start, sosuffixScore = 3
("gin"
).totalScore = 2 + 3 = 5
.- The substring
"engin"
is selected as it starts with"ne"
and ends with"gin"
.
Output:
"engin"
Solution Approach
Use dynamic programming to compute the longest common suffix and prefix efficiently.
Counting Unique Palindromic Substrings
A palindrome is a string that reads the same forwards and backwards, such as "121"
or "tacocat"
.
A substring is a continuous sequence of characters within a string.
Problem
Given a string s
, count how many unique substrings of s
are palindromes.
Example
Input:
s = "mokkori"
Distinct Palindromes:
The unique palindromic substrings in "mokkori"
are:
"m"
"o"
"k"
"kk"
"r"
"i"
"okk"
Output:
7
Solution Approach
- Expand Around Centers: For each character (or pair of adjacent characters), expand outwards while maintaining a palindrome.
- Use a HashSet: Store palindromic substrings to ensure uniqueness.
- Time Complexity:
O(n^2)
using a two-pointer expansion method.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
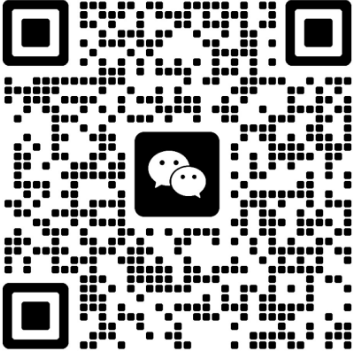