Task 1
Let us consider an infinite sequence of stacks indexed from 0, and an exchange operation that removes two tokens from a stack and adds one token to the next stack.
For example, let's assume there are two tokens on stack 0 and three tokens on stack 1. After that operation, there are:
- 2 tokens from stack 0 may be exchanged for one new token on stack 1.
- After that operation, there are four tokens on stack 1 that may be exchanged for two new tokens on stack 2.
- Finally, a new token may be added to stack 3 by exchanging two tokens from stack 2.
This gives us: stacks 0, 1, and 2 empty, and stack 3 with one token.
Problem Statement
Given the heights of the first N stacks, find the minimum number of tokens that may remain after any number of exchange operations.
You may assume that all of the tokens are identical.
All uninitialized stacks are empty by default.
Function Signature
class Solution { public int solution(int[] A); }
Parameters
int A[]
: An array ofN
integers representing the heights of the firstN
stacks in the sequence.
Returns
int
: The minimum number of tokens which may remain on the stacks after any number of exchange operations.
Example
Input
A = [2, 3, 0, 0]
Process
- Stack 0: 2 tokens → exchanged for 1 token in Stack 1.
- Stack 1: (original 3 + 1 from Stack 0) = 4 tokens → exchanged for 2 tokens in Stack 2.
- Stack 2: 2 tokens → exchanged for 1 token in Stack 3.
Output
1
Constraints
1 ≤ N ≤ 100,000
0 ≤ A[i] ≤ 1,000,000,000
Task 2
A car manufacturer has data about the production processes of N different cars (numbered from 0 to N-1) and wants to maximize the number of cars produced in the upcoming month.
The manufacturing information is described by an array H, where H[k] denotes the number of hours required to produce the k-th car.
There are two assembly lines in the factory:
- The first assembly line works for X hours in a month.
- The second assembly line works for Y hours in a month.
Each car can be constructed using either one of these lines.
Only one car at a time can be produced on each assembly line, and it is not possible to switch lines after starting the car’s production.
Objective
Find the maximum number of different cars that can be produced in the upcoming month.
Function Signature
def solution(H, X, Y)
Parameters
H
: an array ofN
integers representing the time required to produce each car.X
: an integer, the total available hours of the first assembly line.Y
: an integer, the total available hours of the second assembly line.
Returns
- An integer: the maximum number of different cars that can be produced.
Examples
Example 1
H = [1, 1, 3]
X = 1
Y = 1
Output:
2
Explanation:
- Only cars whose assembly time requires 1 hour can be constructed.
Example 2
H = [6, 5, 5, 4, 3]
X = 8
Y = 9
Output:
4
Explanation:
- The cars that need 3 and 5 hours can be produced on the first assembly line.
- The car that needs 5 hours and the car that needs 4 hours can be produced using the second assembly line.
Example 3
H = [6, 5, 2, 1, 8, 1]
X = 17
Y = 5
Output:
5
Explanation:
- The car that needs 5 hours can be produced on the second line.
- The four other cars can be produced on the first line.
Example 4
H = [5, 5, 4, 6]
X = 8
Y = 8
Output:
2
Explanation:
- Only one car can be produced on each line.
Constraints
1 ≤ N ≤ 100,000
1 ≤ H[i] ≤ 1,000,000,000
1 ≤ X, Y ≤ 1,000,000,000
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
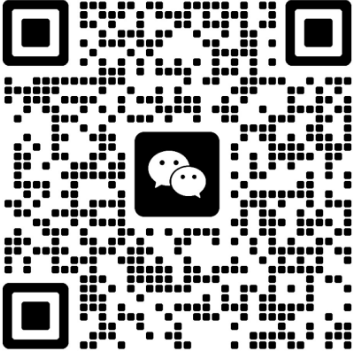