Given an array of integers numbers, your task is to count all numbers that are not equal to numbers[0] or numbers[1], if they exist in the array.
Assume that array indices are 0-based.
Note:
You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(numbers.length²) will fit within the execution time limit.
Example:
1.
For numbers = [4, 3, 2, 3, 2, 5, 4, 3], the output should be:
solution(numbers) = 3.
Explanation:
- numbers[0] = 4, numbers[1] = 3
- There are three elements in numbers that are not equal to 4 or 3:
- numbers[2] = 2
- numbers[4] = 2
- numbers[5] = 5
Thus, the answer is 3.
2.
For numbers = [3, 3, 1, 1, 3], the output should be:
solution(numbers) = 2.
Explanation:
- numbers[0] = 3, numbers[1] = 3
- There are two elements in numbers that are not equal to 3:
- numbers[2] = 1
- numbers[3] = 1
Thus, the answer is 2.
3.
For numbers = [-2], the output should be:
solution(numbers) = 0.
Explanation:
- numbers[0] = -2, and numbers[1] does not exist.
- The only requirement is to count numbers different from -2.
- There are no elements in numbers that are not equal to -2, so the answer is 0.
Input/Output
- Execution time limit: 3 seconds (Java)
- Memory limit: 1 GB
- Input: array of integers numbers
- Output: integer (count of numbers that are not equal to numbers[0] or numbers[1])
You are given a string text consisting of unique lowercase English words that are divided by spaces.
Your task is to count the absolute difference between the number of vowels (which are 'a', 'e', 'i', 'o', 'u') and the number of consonants in each word. You are to return the array of words in the ascending order of these absolute differences. If the absolute difference is the same, sort the words in alphabetical order.
Note:
You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(text.length²) will fit within the execution time limit.
Example:
Input:
text = "penelope lives in hawaii"
Output:
solution(text) = ["in", "penelope", "lives", "hawaii"]
Explanation:
- Word "penelope"
- Consonants: p, n, l, p (4)
- Vowels: e, e, o, e (4)
- Absolute difference: |4 - 4| = 0
- Word "lives"
- Consonants: l, v, s (3)
- Vowels: i, e (2)
- Absolute difference: |3 - 2| = 1
- Word "in"
- Consonants: n (1)
- Vowels: i (1)
- Absolute difference: |1 - 1| = 0
- Word "hawaii"
- Consonants: h, w (2)
- Vowels: a, a, i, i (4)
- Absolute difference: |2 - 4| = 2
Sorting Order:
- Words with absolute difference 0: ["in", "penelope"] (sorted alphabetically).
- Words with absolute difference 1: ["lives"].
- Words with absolute difference 2: ["hawaii"].
Thus, the final output is:
["in", "penelope", "lives", "hawaii"]
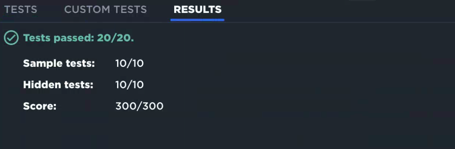
You are given a matrix of integers matrix
, your goal is to find all local maximums in it. An element is considered to be a local maximum if it is not zero and there are no greater or equal elements in its region. The region for the element matrix[i][j]
is the square with the side length of matrix[i][j] * 2 + 1
and center coordinates at (i, j)
, excluding the square’s corner elements. The coordinates of the element is 0
-based indices of the corresponding row and column.
See an example of the region below. For matrix[2][2] = 2
, the size of the square is (matrix[2][2] * 2 + 1) × (matrix[2][2] * 2 + 1)
, which is 5 × 5
, with square’s corner elements being excluded:
Note: if the region is not fully inside the matrix
, no special rules are applied. You should only consider the part of the region within the matrix
.
Return the 2
-dimensional array, where each element is the array of size 2
, containing coordinates (row, col)
of every local maximum within matrix
. The coordinates in the resulting array should be sorted in ascending order by row index or, in case of a tie, by column index.
Note: You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(matrix.length² * matrix[0].length²)
will fit within the execution time limit.
Example
For
matrix = [
[3, 0, 0, 0, 0],
[0, 0, 1, 0, 0],
[0, 0, 2, 0, 0],
[0, 0, 0, 0, 0],
[0, 3, 0, 0, 3]
]
the output should be
solution(matrix) = [[0, 0], [2, 2]]
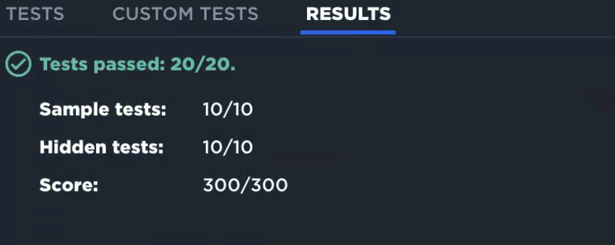
Given an empty array that should contain integers numbers
, your task is to process a list of queries
on it. Specifically, there are two types of queries:
"+x"
- add integerx
tonumbers
.numbers
may contain multiple instances of the same integer."-x"
- remove a single instance of integerx
fromnumbers
.
After processing each query, record the number of pairs in numbers
with a difference equal to a given diff
. The final output should be an array of such values for all queries
.
Notes:
- All numbers in queries are guaranteed to be in the range of
-10⁹
to10⁹
, inclusive. - It is also guaranteed that for every
"-x"
query, the specified numberx
exists innumbers
. - It is guaranteed that the answer for each query fits into a signed 32-bit integer type.
Example
For
queries = ["+4", "+5", "+2", "-4"]
diff = 1
the output should be
solution(queries, diff) = [0, 1, 1, 0]
- Process
queries[0] = "+4"
and add4
tonumbers
, resulting innumbers = [4]
.
There are no pairs withdiff = 1
, so append0
to the output. - Process
queries[1] = "+5"
and add5
tonumbers
, resulting innumbers = [4, 5]
.
The numbers4
and5
have differencediff = 1
, so append1
to the output. - Process
queries[2] = "+2"
and add2
tonumbers
, resulting innumbers = [4, 5, 2]
.
The number of pairs with differencediff = 1
remains the same, so append1
to the output. - Process
queries[3] = "-4"
and remove4
fromnumbers
, resulting innumbers = [5, 2]
.
There are no pairs withdiff = 1
, so append0
to the output.
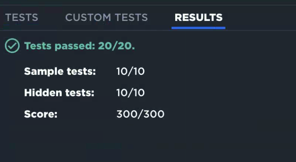
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
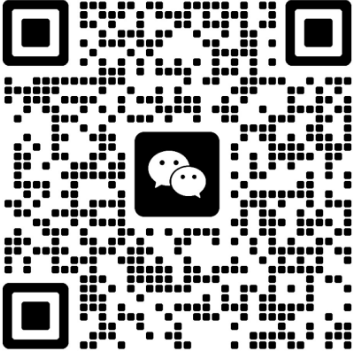